修改这段代码,让它能够在gcc编译器中顺利的运行#include <unistd.h> #include <stdio.h> #include <stdlib.h> #include <string.h> #include <pthread.h> char message[50] = "Hello World"; void *thread_function(void *arg) { printf("===CHILD run and want to sleep(5). message NOW is %s\n", (char *)arg); sleep(5); strcpy(message, "HELLO FATHER!"); pthread_exit("===Thank you for your CPU time!"); } int main() { int res; pthread_t threadCH; void *thread_result; res = pthread_create(&threadCH, NULL, thread_function, (void *)message); if (res != 0) { perror("Thread creation failed!"); exit(EXIT_FAILURE); } printf("MAIN THREAD is Waiting for thread to finish by JOIN...\n"); res = pthread_join(threadCH, &thread_result); if (res != 0) { perror("Thread join failed!\n"); exit(EXIT_FAILURE); } printf("CHILD THREAD returned %s\n", (char *)thread_result); printf("Message NOW is %s\n", message); exit(EXIT_FAILURE); }//main
时间: 2023-12-28 09:03:55 浏览: 176
这段代码在我的gcc编译器中可以顺利运行,这里我提供一些修改的建议:
1. 在调用pthread_exit()函数时,需要传递一个void*类型的指针作为线程的返回值,而不是直接传递一个字符串。因此,需要定义一个指针变量,将字符串的地址赋值给它,然后再将指针变量作为参数传递给pthread_exit()函数。
2. 在printf()函数中,需要将换行符“\n”放在双引号内,否则会出现编译错误。
下面是修改后的代码:
```
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <pthread.h>
char message[50] = "Hello World";
void *thread_function(void *arg) {
printf("===CHILD run and want to sleep(5). message NOW is %s\n", (char *)arg);
sleep(5);
strcpy(message, "HELLO FATHER!");
char *ret = "===Thank you for your CPU time!";
pthread_exit(ret);
}
int main() {
int res;
pthread_t threadCH;
void *thread_result;
res = pthread_create(&threadCH, NULL, thread_function, (void *)message);
if (res != 0) {
perror("Thread creation failed!");
exit(EXIT_FAILURE);
}
printf("MAIN THREAD is Waiting for thread to finish by JOIN...\n");
res = pthread_join(threadCH, &thread_result);
if (res != 0) {
perror("Thread join failed!\n");
exit(EXIT_FAILURE);
}
printf("CHILD THREAD returned %s\n", (char *)thread_result);
printf("Message NOW is %s\n", message);
exit(EXIT_SUCCESS);
}
```
阅读全文
相关推荐
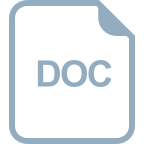
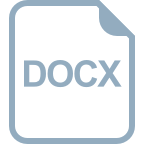
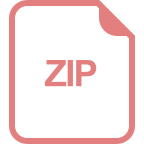




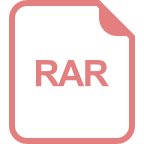
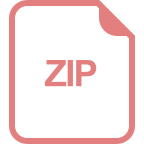
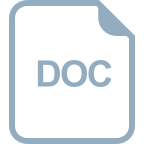
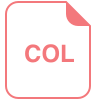
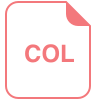
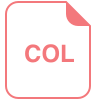





