Newtonsoft 排序
时间: 2023-12-06 19:48:10 浏览: 74
Newtonsoft.Json 是一个流行的 JSON 库,它提供了一些方法来处理 JSON 数据。然而,Newtonsoft.Json 并没有直接提供排序 JSON 数据的功能。如果你想要对 JSON 数据进行排序,你可以先将其转换为适当的数据结构(例如 Dictionary 或 List),然后使用适当的排序算法对数据进行排序。完成排序后,你可以再将排序后的数据转换回 JSON 格式。
以下是一个使用 Newtonsoft.Json 对 JSON 数据进行排序的示例代码:
```csharp
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.Linq;
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
public class Program
{
public static void Main()
{
string json = @"[
{ ""Name"": ""John"", ""Age"": 30 },
{ ""Name"": ""Jane"", ""Age"": 25 },
{ ""Name"": ""Bob"", ""Age"": 40 }
]";
List<Person> people = JsonConvert.DeserializeObject<List<Person>>(json);
List<Person> sortedPeople = people.OrderBy(p => p.Name).ToList();
string sortedJson = JsonConvert.SerializeObject(sortedPeople, Formatting.Indented);
Console.WriteLine(sortedJson);
}
}
```
在上面的示例中,我们首先将 JSON 数据转换为 `List<Person>` 对象。然后使用 LINQ 的 `OrderBy` 方法对列表进行排序,并将结果转换为 `List<Person>` 对象。后,我们使用 `JsonConvert.SerializeObject` 方法将排序后的列表转换回 JSON 格式,并使用 `Formatting.Indented` 参数指定输出格式为带缩进的格式。
运行以上代码,输出将会是按姓名排序后的 JSON 数据:
```json
[
{
"Name": "Bob",
"Age": 40
},
{
"Name": "Jane",
"Age": 25
},
{
"Name": "John",
"Age": 30
}
]
```
这是一个简单的示例,你可以根据实际需求进行相应的修改和扩展。希望能对你有所帮助!
阅读全文
相关推荐
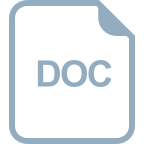
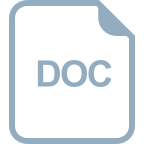
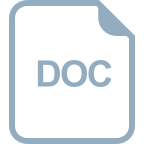
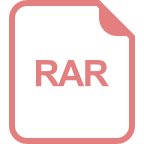
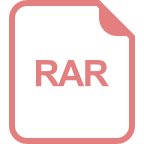
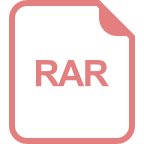
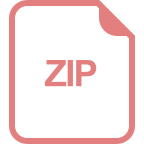
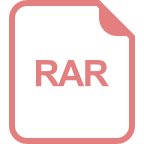
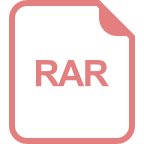
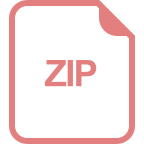
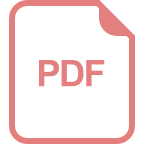
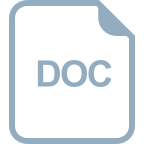

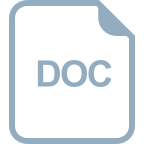
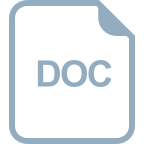
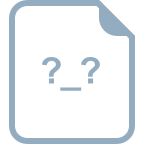
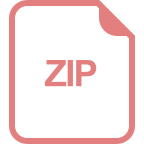