基于stm32的串口通信课程设计
时间: 2023-12-17 20:02:11 浏览: 360
基于STM32的串口通信课程设计可以通过以下步骤实现:
1. 确定硬件平台:选择STM32F103单片机作为主控芯片,同时选择串口通信模块和LED模块作为外设。
2. 确定通信协议:选择虚拟串口软件实现串口助手与STM32的通信,可以使用常见的串口通信协议如UART、SPI等。
3. 实现LED控制:通过串口助手分别发送'A'、'B'、'C'控制三个LED亮;分别发送'a'、'b'、'c'控制三个LED灭。
4. 实现OLED显示:OLED显示通过串口接收到的字符数据,并且将收到的数据通过串口发送至串口助手端,实现串口双向通信。
5. 实现LoRa数据收发:使用Ra-01SC模组和LLCC68芯片实现LoRa数据的收发。
以下是一个基于STM32的串口通信课程设计的代码示例:
```c
#include "stm32f10x.h"
#include "stdio.h"
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void LED_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_13;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOC, &GPIO_InitStructure);
}
void USART1_SendChar(char ch)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
USART_SendData(USART1, (uint8_t)ch);
}
void USART1_SendString(char* str)
{
while (*str)
{
USART1_SendChar(*str++);
}
}
char USART1_ReceiveChar(void)
{
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
return (char)USART_ReceiveData(USART1);
}
int main(void)
{
char ch;
USART1_Init();
LED_Init();
while (1)
{
ch = USART1_ReceiveChar();
switch (ch)
{
case 'A':
GPIO_SetBits(GPIOC, GPIO_Pin_13);
break;
case 'B':
GPIO_ResetBits(GPIOC, GPIO_Pin_13);
break;
case 'C':
USART1_SendString("Hello World!\r\n");
break;
default:
break;
}
}
}
```
阅读全文
相关推荐
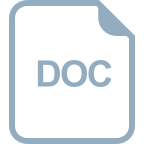
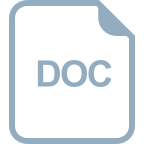
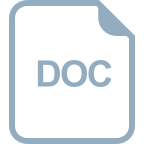
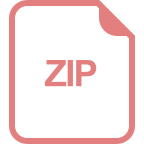
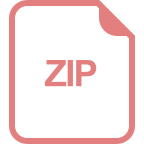
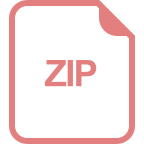
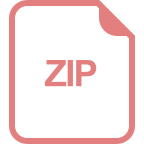
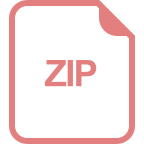
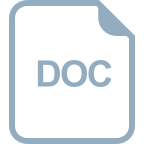
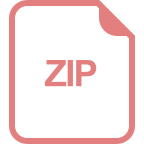
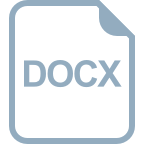
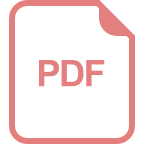
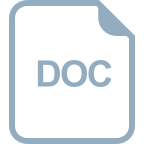
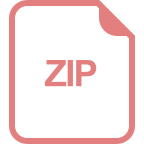
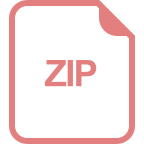
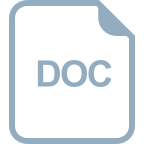
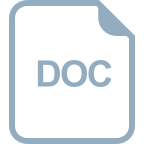
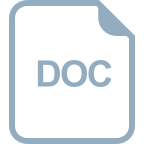
