SpringBoot接口测试
时间: 2024-12-29 12:23:45 浏览: 6
### 测试Spring Boot应用程序的API或接口
对于Spring Boot应用程序而言,执行API或接口测试至关重要。这不仅有助于验证业务逻辑是否按预期工作,还能确保不同组件间的交互无误。
#### 使用@SpringBootTest进行集成测试
为了进行全面的集成测试,`@SpringBootTest`注解提供了加载整个应用程序上下文的能力[^2]。这意味着可以在真实的环境中模拟HTTP请求并评估响应结果。下面是一个简单的例子来展示如何配置此类测试:
```java
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.*;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.*;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.autoconfigure.web.servlet.AutoConfigureMockMvc;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.http.MediaType;
import org.springframework.test.web.servlet.MockMvc;
@SpringBootTest
@AutoConfigureMockMvc
public class UserControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void shouldReturnDefaultMessage() throws Exception {
this.mockMvc.perform(get("/api/user").accept(MediaType.APPLICATION_JSON))
.andExpect(status().isOk())
.andExpect(content().string("Hello, World"));
}
}
```
这段代码展示了怎样利用`MockMvc`对象发送GET请求给指定端点,并断言返回的状态码以及消息体的内容。
#### 利用Postman或其他第三方工具
除了编写单元和集成测试外,还可以借助像Postman这样的外部工具来进行手动或自动化测试。这类工具有助于快速创建复杂的HTTP请求序列,支持多种认证方式,并能直观查看服务器反馈的数据结构。
#### RestAssured库用于更简洁的测试语法
RestAssured是一种流行的Java库,它允许开发者以更加流畅的方式编写RESTful Web服务的验收测试。相较于传统的JUnit风格,RestAssured提供了一种链式调用来描述期望的行为模式,从而提高了代码可读性和维护效率。
```java
import io.restassured.RestAssured;
import static io.restassured.RestAssured.given;
import static org.hamcrest.Matchers.*;
// 设置基础URL
RestAssured.baseURI = "http://localhost:8080";
given().
when().
get("/api/user").
then().
assertThat().
statusCode(200).
and().
body(matchesJsonSchemaInClasspath("schemas/user-schema.json"));
```
上述片段说明了如何使用RestAssured发起GET请求至特定路径,并通过JSON Schema校验返回数据的有效性。
阅读全文
相关推荐





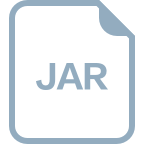


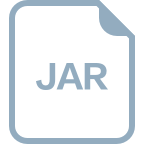








