exitthread
时间: 2023-12-10 22:01:42 浏览: 26
exitthread是一个在编程领域中常用的术语,指的是结束或退出线程的操作。在多线程编程中,每个线程都是独立运行的,exitthread可以用来指示线程停止运行并退出。通常情况下,线程在完成任务之后会自动退出,但有时候我们需要手动调用exitthread来结束线程的执行。
在使用exitthread时,需要注意一些潜在的问题,比如线程资源的释放、内存的回收等。正确地使用exitthread可以避免一些潜在的内存泄漏和资源浪费问题,确保程序的稳定和高效运行。
exitthread的使用需要依赖于具体的编程语言和平台,不同的语言和平台可能有不同的实现方式和调用规则。一般情况下,我们可以通过调用特定的API函数或者使用语言内置的关键字来实现exitthread操作。
总而言之,exitthread是一个重要的操作,能够在多线程编程中起到关键作用。合理使用exitthread可以提高程序的性能和稳定性,但也需要注意潜在的问题。所以在编写多线程程序时,需要谨慎使用exitthread,并且充分了解相关的编程语言和平台的特性和规范。
相关问题
用c++使用系统调用函数 CreateThread()创建一个子线程并在子线程序中显示:Thread isRuning!。为了能让用户清楚地看到线程的运行情况使用函数 Sleep()使线程挂起5s,之后使用函数ExitThread(0)撤销线程。
下面是一个使用 `CreateThread()` 函数创建子线程的例子,其中子线程会显示 "Thread isRunning!",然后挂起5秒钟后退出。
```c++
#include <windows.h>
#include <iostream>
using namespace std;
// 子线程的入口函数
DWORD WINAPI ThreadProc(LPVOID lpParam) {
cout << "Thread isRunning!" << endl;
Sleep(5000); // 挂起5秒钟
ExitThread(0); // 退出线程
}
int main() {
HANDLE hThread;
DWORD dwThreadId;
// 创建子线程
hThread = CreateThread(NULL, 0, ThreadProc, NULL, 0, &dwThreadId);
if (hThread == NULL) {
cout << "CreateThread failed!" << endl;
return 1;
}
// 等待子线程结束
WaitForSingleObject(hThread, INFINITE);
// 关闭线程句柄
CloseHandle(hThread);
return 0;
}
```
当程序运行时,会创建一个子线程并显示 "Thread isRunning!",然后挂起5秒钟后退出。主线程会等待子线程结束后再退出。
If Not ShowMyApp() Then Me.Close() 'EDIT(自動) >>> SR 2023/03/10 'End System.Windows.Forms.Application.ExitThread() System.Diagnostics.Process.GetCurrentProcess.Kill() '<<< EDIT(自動) 2023/03/10
这段代码是一个 VB.NET 中的条件语句,如果 `ShowMyApp()` 函数返回 `False`,则会执行以下代码块:
```vb.net
Me.Close()
System.Windows.Forms.Application.ExitThread()
System.Diagnostics.Process.GetCurrentProcess.Kill()
```
其中,`Me.Close()` 会关闭当前窗体,`System.Windows.Forms.Application.ExitThread()` 会退出当前应用程序的消息循环,`System.Diagnostics.Process.GetCurrentProcess.Kill()` 会强制结束当前进程。
需要注意的是,这段代码中的注释指出某些部分被自动编辑过,可能是由于某些原因修改代码的工具或程序所为。
相关推荐
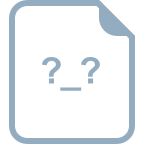
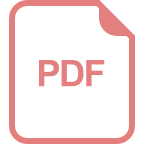
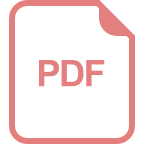












