用c实现系统调用函数CreateThread()创建多个子线程并发运行,并在子线程序中显示:Thread is running!,使用函数sleep()使得线程挂起10s,之后使用函数ExitThread()撤销线程。
时间: 2024-09-27 17:06:03 浏览: 46
在C语言中,创建并管理线程通常需要使用`CreateThread`、`Sleep`和`ExitThread`等Windows API函数。这里是一个简单的示例,展示了如何创建两个并发运行的子线程:
```c
#include <windows.h>
#include <stdio.h>
// 线程函数模板
void* thread_function(LPVOID lpParam) {
char *message = (char*)lpParam;
printf("Thread is running! (%s)\n", message);
Sleep(10000); // 挂起10秒
ExitThread(0); // 撤销线程
return NULL; // 这行在实际应用中不需要,因为线程会立即结束
}
int main() {
const int num_threads = 2;
DWORD thread_id[num_threads];
LPVOID param[num_threads] = {"Thread 1", "Thread 2"};
// 创建并启动每个线程
for (int i = 0; i < num_threads; i++) {
if (CreateThread(NULL, 0, thread_function, param[i], 0, &thread_id[i]) == 0) {
printf("Error creating thread %d\n", i);
exit(1);
}
else {
printf("Created thread with ID %d\n", thread_id[i]);
}
}
// 主线程等待所有子线程完成
for (int i = 0; i < num_threads; i++) {
WaitForSingleObject(thread_id[i], INFINITE); // 等待线程结束
}
printf("All threads finished.\n");
return 0;
}
```
在这个例子中,`thread_function`是线程的目标函数,它接收一个参数`lpParam`作为消息字符串。`main`函数首先创建指定数量的线程,然后通过`WaitForSingleObject`等待它们全部结束。
阅读全文
相关推荐
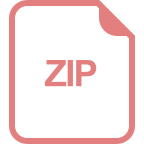
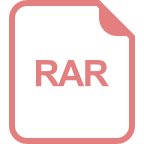
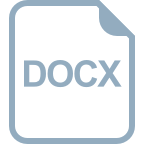















