用c语言实现以下功能:完成两个子线程之间的互斥。在主线程中使用系统调用函数CreateThread()创建两个子线程,并使两个子线程互斥地使用全局变量count。
时间: 2024-10-16 07:21:28 浏览: 27
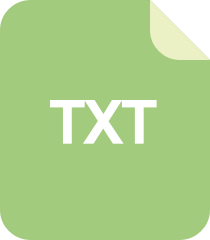
在C#中主线程和子线程如何实现互相传递数据.txt
在 C 语言中,我们可以使用 `pthread` 模块来创建和管理线程以及实现互斥访问。为了实现两个子线程之间的互斥,可以使用 `pthread_mutex_t` 结构体代表互斥锁。下面是一个简单的示例,展示了如何创建两个子线程并互斥地增加全局变量 count:
```c
#include <stdio.h>
#include <pthread.h>
// 定义全局变量和互斥锁
int count = 0;
pthread_mutex_t mutex;
void* thread_func_1(void* arg) {
for (int i = 0; i < 10; ++i) {
pthread_mutex_lock(&mutex); // 获取锁
printf("Thread 1: Count is %d\n", count++);
pthread_mutex_unlock(&mutex); // 释放锁
}
return NULL;
}
void* thread_func_2(void* arg) {
for (int i = 0; i < 10; ++i) {
pthread_mutex_lock(&mutex); // 获取锁
printf("Thread 2: Count is %d\n", count++);
pthread_mutex_unlock(&mutex); // 释放锁
}
return NULL;
}
int main() {
// 初始化互斥锁
pthread_mutex_init(&mutex, NULL);
// 创建两个线程
pthread_t t1, t2;
int rc1 = pthread_create(&t1, NULL, thread_func_1, NULL);
if (rc1 != 0) {
perror("Error creating thread 1");
exit(1);
}
int rc2 = pthread_create(&t2, NULL, thread_func_2, NULL);
if (rc2 != 0) {
perror("Error creating thread 2");
exit(1);
}
// 等待两个线程结束
pthread_join(t1, NULL);
pthread_join(t2, NULL);
// 清理资源
pthread_mutex_destroy(&mutex);
printf("Final count: %d\n", count);
return 0;
}
```
在这个例子中,`thread_func_1` 和 `thread_func_2` 都会尝试获取互斥锁,当其中一个线程获得锁后,其他线程将被阻塞直到锁被释放。这样就实现了两个子线程对全局变量 `count` 的互斥访问。
阅读全文
相关推荐
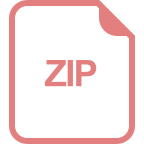
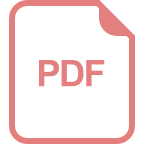














