完成两个子线程的互斥。在主线程中使用系统调用CreateThread()创建两个子线程,使用创建临界区的方式使两个子线程互斥地使用全局变量count,最后在主线程内挂起2s后输出count的值。两个子线程的代码如下所示。 P1,P2两进程共享变量COUNT(COUNT的初值为5) P1:{ R1=COUNT; 挂起1000ms R1=R1+1; COUNT=R1; 输出COUNT的值; } P2:{ R2=COUNT; 挂起500ms R2=R2+1; COUNT=R2; 输出COUNT的值; }
时间: 2024-02-26 19:58:41 浏览: 74
以下是使用临界区实现两个子线程互斥的示例代码:
```c++
#include <windows.h>
#include <stdio.h>
// 全局变量
int count = 5;
// 临界区
CRITICAL_SECTION cs;
// 子线程1
DWORD WINAPI Thread1(LPVOID lpParam) {
for (int i = 0; i < 5; i++) {
EnterCriticalSection(&cs); // 进入临界区
int r1 = count;
Sleep(1000);
r1++;
count = r1;
printf("Thread1: count = %d\n", count);
LeaveCriticalSection(&cs); // 离开临界区
}
return 0;
}
// 子线程2
DWORD WINAPI Thread2(LPVOID lpParam) {
for (int i = 0; i < 5; i++) {
EnterCriticalSection(&cs); // 进入临界区
int r2 = count;
Sleep(500);
r2++;
count = r2;
printf("Thread2: count = %d\n", count);
LeaveCriticalSection(&cs); // 离开临界区
}
return 0;
}
int main() {
InitializeCriticalSection(&cs); // 初始化临界区
// 创建子线程1
HANDLE hThread1 = CreateThread(NULL, 0, Thread1, NULL, 0, NULL);
if (hThread1 == NULL) {
printf("Failed to create thread1! Error code: %d\n", GetLastError());
return 1;
}
// 创建子线程2
HANDLE hThread2 = CreateThread(NULL, 0, Thread2, NULL, 0, NULL);
if (hThread2 == NULL) {
printf("Failed to create thread2! Error code: %d\n", GetLastError());
return 1;
}
// 等待子线程结束
WaitForSingleObject(hThread1, INFINITE);
WaitForSingleObject(hThread2, INFINITE);
// 销毁临界区
DeleteCriticalSection(&cs);
// 输出count的值
printf("count = %d\n", count);
// 等待2s
Sleep(2000);
return 0;
}
```
在以上代码中,我们使用了Windows API中提供的临界区对象来保证两个子线程对全局变量count的互斥访问。在子线程中,我们使用EnterCriticalSection函数进入临界区,使用LeaveCriticalSection函数离开临界区。这样,同一时刻只有一个子线程可以进入临界区,从而保证了两个子线程对count的互斥访问。
在主线程中,我们使用CreateThread函数创建了两个子线程,并使用WaitForSingleObject函数等待子线程结束。最后,我们输出了count的值,并使用Sleep函数等待2s,以便我们观察输出结果。
阅读全文
相关推荐
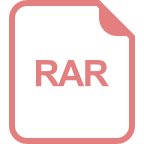
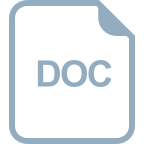
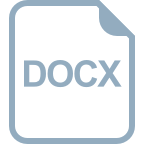




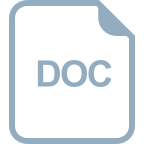
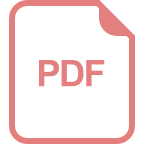
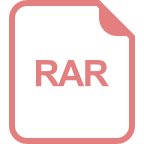
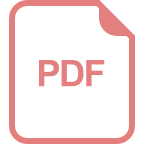
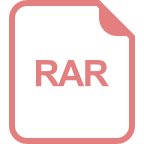
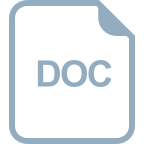
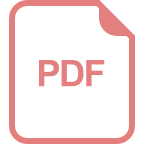
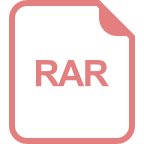
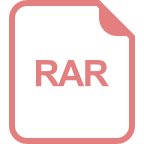
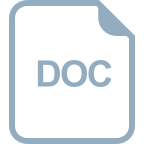
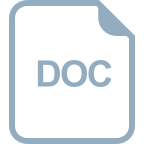
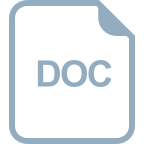