编写一个十进制转换二进制的C语言代码
时间: 2024-11-09 12:19:32 浏览: 70
在C语言中,你可以使用循环和位运算来将一个十进制数转换成二进制。以下是一个简单的示例程序:
```c
#include <stdio.h>
#include <string.h>
// Function to convert decimal to binary
void decToBin(int num) {
int binaryNum[32]; // Assuming the maximum number will be less than 2^31
int i = 0;
while (num > 0) {
binaryNum[i] = num % 2; // Get the last bit
num = num / 2; // Remove the last bit from the number
i++;
}
// Print the binary representation in reverse order
for (int j = i - 1; j >= 0; j--) {
printf("%d", binaryNum[j]);
}
}
int main() {
int decimal;
printf("Enter a decimal number: ");
scanf("%d", &decimal);
printf("Binary equivalent of %d is ", decimal);
decToBin(decimal);
return 0;
}
```
当你运行这个程序并输入一个十进制数字,它会打印出相应的二进制表示。注意,这里我们假设输入的十进制数不超过`int`类型的存储范围。
阅读全文
相关推荐
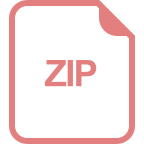
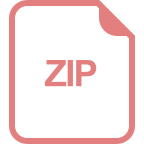
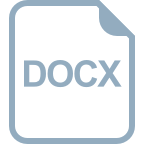
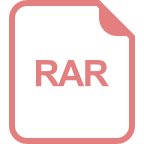









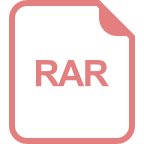
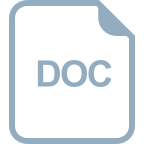



