训练resnet模型并且保存代码
时间: 2023-12-26 10:22:39 浏览: 33
抱歉,作为AI语言模型,我没有实际的计算和存储功能,无法为您提供完整的代码。但我可以给您提供一些基本的步骤和示例代码,帮助您开始训练ResNet模型并保存代码。
1. 导入必要的库
```python
import tensorflow as tf
from tensorflow.keras.layers import Dense, Flatten, Conv2D
from tensorflow.keras import Model
from tensorflow.keras.applications.resnet50 import ResNet50
```
2. 加载数据集
```python
# 加载数据集,这里以CIFAR-10为例
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.cifar10.load_data()
# 数据预处理
x_train, x_test = x_train / 255.0, x_test / 255.0
```
3. 定义ResNet模型
```python
# 加载ResNet50预训练模型
base_model = ResNet50(weights='imagenet', include_top=False, input_shape=(32, 32, 3))
# 冻结前面的层不参与训练
for layer in base_model.layers:
layer.trainable = False
# 定义新的输出层
x = base_model.output
x = Flatten()(x)
x = Dense(1024, activation='relu')(x)
predictions = Dense(10, activation='softmax')(x)
# 组合成新的模型
model = Model(inputs=base_model.input, outputs=predictions)
```
4. 编译模型并训练
```python
model.compile(optimizer='adam',
loss='sparse_categorical_crossentropy',
metrics=['accuracy'])
model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test))
```
5. 保存模型
```python
# 保存模型结构和权重
model.save('my_resnet_model.h5')
# 保存模型结构
model_json = model.to_json()
with open('my_resnet_model.json', 'w') as json_file:
json_file.write(model_json)
# 保存权重
model.save_weights('my_resnet_weights.h5')
```
以上是一个简单的ResNet模型训练和保存的示例代码,您可以根据自己的需求进行修改和扩展。
相关推荐
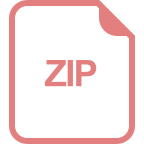
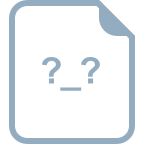
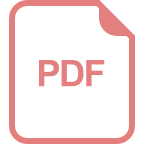
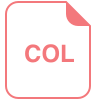













