python获取邮件发送时间差8小时
时间: 2024-09-15 18:04:46 浏览: 43
在Python中,如果你想要获取邮件发送时间并减去8小时,通常你需要处理的是日期和时间的计算。你可以使用内置的`datetime`模块来进行这样的操作。假设你已经有了一个表示邮件发送时间的`datetime`对象,例如`mail_sent_at`,你可以这样做:
```python
from datetime import timedelta
# 假设 mail_sent_at 是一个 datetime 对象
mail_sent_at = datetime.strptime("邮件发送的具体时间", "%Y-%m-%d %H:%M:%S") # 根据实际情况解析时间字符串
# 减去8小时
time_8_hours_later = mail_sent_at - timedelta(hours=8)
print(time_8_hours_later)
```
请替换`"邮件发送的具体时间"`为你实际存储的邮件发送时间字符串,并按照适当的格式(如"%Y-%m-%d %H:%M:%S"代表"年-月-日 时:分:秒")。如果你想从某个电子邮件库中获取发送时间,比如IMAP4或POP3,那么首先需要读取这个信息,这取决于你使用的库。
相关问题
Python通过邮件发送文件
Python可以通过邮件发送文件,可以使用smtplib和email模块来实现。以下是一个示例代码:
```python
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.application import MIMEApplication
# 发送邮件的基本信息
sender = 'youremail@yourdomain.com'
password = 'yourpassword'
receiver = 'receiveremail@receiverdomain.com'
subject = 'Email Subject'
# 创建邮件对象,并添加邮件内容
msg = MIMEMultipart()
msg['From'] = sender
msg['To'] = receiver
msg['Subject'] = subject
# 添加邮件正文
body = 'Email Body'
msg.attach(MIMEText(body, 'plain'))
# 添加附件
with open('filename', 'rb') as f:
attachment = MIMEApplication(f.read(), _subtype='txt')
attachment.add_header('Content-Disposition', 'attachment', filename='filename.txt')
msg.attach(attachment)
# 发送邮件
try:
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login(sender, password)
text = msg.as_string()
server.sendmail(sender, receiver, text)
print('Email sent successfully!')
except Exception as e:
print(e)
finally:
server.quit()
```
这个示例代码通过SMTP服务器发送邮件。需要注意的是,如果使用Gmail邮箱发送邮件,需要在Gmail账户中开启\"允许安全性较低的应用程序\"选项。同时,需要修改代码中的SMTP服务器地址和端口号,以及发件人、收件人、附件等相关信息。
python电子邮件发送
要使用Python 3发送电子邮件,可以使用内置的smtplib库。以下是一个简单的示例代码:
```python
import smtplib
from email.mime.text import MIMEText
def send_email(sender, receiver, subject, message):
msg = MIMEText(message)
msg['Subject'] = subject
msg['From'] = sender
msg['To'] = receiver
try:
smtpObj = smtplib.SMTP('smtp.gmail.com', 587) # 根据你的邮箱提供商更改SMTP服务器和端口
smtpObj.starttls()
smtpObj.login('你的邮箱地址', '你的邮箱密码')
smtpObj.sendmail(sender, receiver, msg.as_string())
smtpObj.quit()
print("邮件发送成功")
except Exception as e:
print("邮件发送失败:", str(e))
# 用法示例
sender = '发件人邮箱地址'
receiver = '收件人邮箱地址'
subject = '测试邮件'
message = '这是一封测试邮件'
send_email(sender, receiver, subject, message)
```
在上面的代码中,你需要将`发件人邮箱地址`和`收件人邮箱地址`替换为实际的邮箱地址。你还需要提供SMTP服务器和端口,这取决于你的邮箱提供商。以Gmail为例,默认的SMTP服务器是`smtp.gmail.com`,端口是`587`。
此外,你需要提供发件人邮箱地址和密码,以便登录SMTP服务器进行身份验证。请确保启用了所使用的发件人邮箱的SMTP访问权限。
以上是一个基本的示例,你可以根据需要进行扩展和自定义。
相关推荐
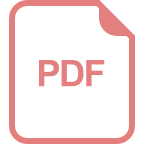
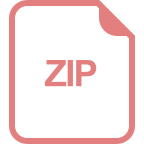
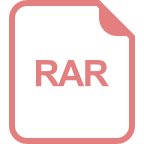













