用python读取并可视化obj文件
时间: 2023-07-18 11:13:15 浏览: 1447
要读取和可视化obj文件,可以使用Python中的Open3D库。以下是一个简单的示例代码:
```python
import open3d as o3d
# 读取obj文件
mesh = o3d.io.read_triangle_mesh("example.obj")
# 可视化网格
o3d.visualization.draw_geometries([mesh])
```
该代码会读取名为`example.obj`的obj文件,并在窗口中显示网格模型。你可以使用鼠标旋转和缩放视角来查看模型。
请确保已经安装了Open3D库,可以使用以下命令在终端中安装:
```
pip install open3d
```
注意:如果你的obj文件包含纹理贴图,你需要使用`read_triangle_mesh()`函数的`create_texture_from_texture_file()`参数来读取纹理贴图,并将其附加到网格模型上。
相关问题
用python读取并同时可视化多个obj文件
要读取并同时可视化多个obj文件,可以使用Python中的Open3D库。以下是一个简单的示例代码:
```python
import open3d as o3d
# 读取多个obj文件
meshes = []
meshes.append(o3d.io.read_triangle_mesh("example1.obj"))
meshes.append(o3d.io.read_triangle_mesh("example2.obj"))
meshes.append(o3d.io.read_triangle_mesh("example3.obj"))
# 将多个网格合并为一个
combined_mesh = o3d.geometry.TriangleMesh.create_merged_triangle_mesh(meshes)
# 可视化合并后的网格
o3d.visualization.draw_geometries([combined_mesh])
```
该代码会读取名为`example1.obj`、`example2.obj`和`example3.obj`的三个obj文件,并将它们合并为一个网格模型。然后,它会在窗口中显示合并后的模型。你可以使用鼠标旋转和缩放视角来查看模型。
请确保已经安装了Open3D库,可以使用以下命令在终端中安装:
```
pip install open3d
```
注意:要将多个网格合并为一个,需要确保它们具有相同的拓扑结构和顶点属性。如果它们的属性不同,你需要手动将它们对齐和匹配。
obj文件进行可视化python代码
要将obj文件进行可视化,可以使用Python中的PyOpenGL库。下面是一个简单的代码示例,可以读取.obj文件并将其渲染为3D模型:
```python
from OpenGL.GL import *
from OpenGL.GLUT import *
from OpenGL.GLU import *
import sys
def draw():
glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT)
glLoadIdentity()
gluLookAt(0,0,5,0,0,0,0,1,0)
glColor3f(1.0, 1.0, 1.0)
glBegin(GL_TRIANGLES)
for line in open('model.obj', 'r'):
if line.startswith('#'): continue
values = line.split()
if not values: continue
if values[0] == 'v':
glVertex3f(*map(float, values[1:]))
elif values[0] == 'vn':
continue
elif values[0] == 'f':
for v in values[1:]:
glVertex3f(*map(float, v.split('/')))
glEnd()
glutSwapBuffers()
glutInit(sys.argv)
glutInitDisplayMode(GLUT_RGBA|GLUT_DOUBLE|GLUT_DEPTH)
glutInitWindowSize(640, 480)
glutCreateWindow("Obj Viewer")
glutDisplayFunc(draw)
glEnable(GL_DEPTH_TEST)
glutMainLoop()
```
在上面的代码中,我们首先导入了必要的库。然后,我们定义了一个draw()函数,该函数用于渲染3D模型。在这个函数里,我们首先清空颜色缓存和深度缓存,然后设置摄像机的位置,让它面对模型。接着我们使用glColor3f()设置绘制颜色,glBegin(GL_TRIANGLES)开始绘制三角形,然后读取obj文件中的数据并将其转换为OpenGL可用的数据,最后使用glEnd()结束绘制。在最后,我们启用深度测试并调用glutMainLoop()开始渲染窗口。
请注意,这只是一个简单的示例,可能无法处理所有类型的.obj文件,但对于大多数情况它应该是足够的。
相关推荐
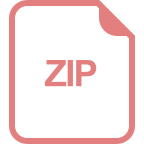
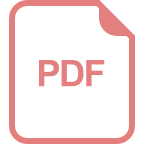












