、使用纯JSP技术开发一个web应用,其使用login.jsp进行登录,登录错误则返回登录页面并实现错误回显;成功则跳到list.jsp,显示欢迎xxx登录并用表格显示一个商品列表(数据自行模拟,至少5行4列)。
时间: 2023-06-13 08:06:36 浏览: 85
为了实现这个功能,您需要遵循以下步骤:
1. 创建一个login.jsp页面,包含一个表单,请求方法为POST,表单中有用户名和密码两个文本框以及一个提交按钮。
2. 创建一个Servlet来处理登录请求,将请求和响应对象作为参数传递给Servlet,从请求对象中获取用户名和密码,然后验证它们是否正确。如果用户名或密码不正确,则将错误消息设置为请求属性,然后将请求转发回login.jsp页面。如果用户名和密码正确,则将用户名设置为会话属性,然后重定向到list.jsp页面。
3. 创建一个list.jsp页面,它将检查会话属性中是否有用户名,如果有则显示欢迎消息和商品列表。商品列表可以使用HTML表格来呈现,您可以将数据硬编码到JSP页面中,或者从数据库或其他数据源获取数据。
下面是一个简单的实现示例:
login.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h1>Login</h1>
<% if (request.getAttribute("error") != null) { %>
<p><font color="red"><%= request.getAttribute("error") %></font></p>
<% } %>
<form method="post" action="login">
<label>Username:</label><br/>
<input type="text" name="username"><br/>
<label>Password:</label><br/>
<input type="password" name="password"><br/><br/>
<input type="submit" value="Login">
</form>
</body>
</html>
```
LoginServlet.java:
```
@WebServlet("/login")
public class LoginServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private static final String USERNAME = "admin";
private static final String PASSWORD = "password";
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String username = request.getParameter("username");
String password = request.getParameter("password");
if (USERNAME.equals(username) && PASSWORD.equals(password)) {
request.getSession().setAttribute("username", username);
response.sendRedirect("list.jsp");
} else {
request.setAttribute("error", "Invalid username or password");
request.getRequestDispatcher("login.jsp").forward(request, response);
}
}
}
```
list.jsp:
```
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>List</title>
</head>
<body>
<% if (session.getAttribute("username") != null) { %>
<h1>Welcome <%= session.getAttribute("username") %>!</h1>
<table border="1">
<tr>
<th>Product Name</th>
<th>Price</th>
<th>Quantity</th>
<th>Description</th>
</tr>
<tr>
<td>Product 1</td>
<td>$10.00</td>
<td>5</td>
<td>Description 1</td>
</tr>
<tr>
<td>Product 2</td>
<td>$20.00</td>
<td>3</td>
<td>Description 2</td>
</tr>
<tr>
<td>Product 3</td>
<td>$30.00</td>
<td>2</td>
<td>Description 3</td>
</tr>
<tr>
<td>Product 4</td>
<td>$40.00</td>
<td>1</td>
<td>Description 4</td>
</tr>
<tr>
<td>Product 5</td>
<td>$50.00</td>
<td>4</td>
<td>Description 5</td>
</tr>
</table>
<% } else { %>
<p>You must login first</p>
<a href="login.jsp">Login</a>
<% } %>
</body>
</html>
```
阅读全文
相关推荐
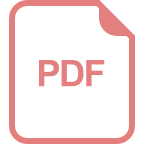
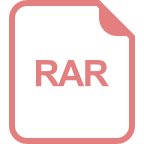
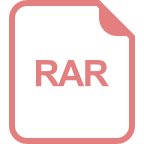
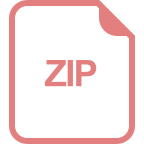
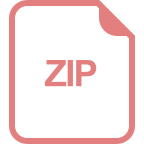
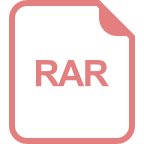
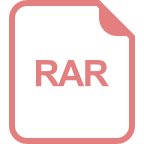
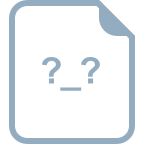
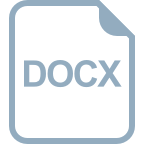
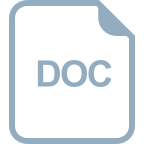
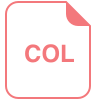
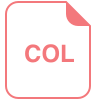

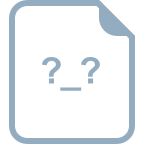
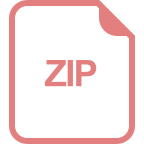
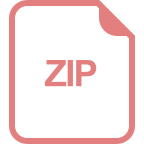