1.使用Spring框架的AOP技术完成程序,程序输出如图所示。其中定义的切面的切入点以方法为基本单位,当调用bean..S.tudent...show()时执行。< 前置通知运行 环绕通知开始运行 show()函数执行,输出信息:Name : zhangsan,Age: 30环绕通知结束运行 后置通知运行 最终通知运行 <
时间: 2024-03-10 16:50:42 浏览: 93
好的,下面是示例代码:
首先,我们需要定义一个切面类,实现前置通知、后置通知、环绕通知和最终通知:
```java
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.*;
@Aspect
public class StudentAspect {
@Before("execution(* bean.Student.show(..))")
public void beforeAdvice(JoinPoint joinPoint) {
System.out.println("< 前置通知运行");
}
@AfterReturning("execution(* bean.Student.show(..))")
public void afterReturningAdvice(JoinPoint joinPoint) {
System.out.println("后置通知运行");
}
@Around("execution(* bean.Student.show(..))")
public Object aroundAdvice(ProceedingJoinPoint proceedingJoinPoint) throws Throwable {
System.out.println("环绕通知开始运行");
Object result = proceedingJoinPoint.proceed();
System.out.println("环绕通知结束运行");
return result;
}
@After("execution(* bean.Student.show(..))")
public void afterAdvice(JoinPoint joinPoint) {
System.out.println("最终通知运行");
}
}
```
然后,我们需要在Spring配置文件中定义该切面:
```xml
<bean id="studentAspect" class="com.example.StudentAspect"/>
<aop:config>
<aop:aspect ref="studentAspect">
<aop:pointcut id="showPointcut" expression="execution(* bean.Student.show(..))"/>
<aop:before method="beforeAdvice" pointcut-ref="showPointcut"/>
<aop:after-returning method="afterReturningAdvice" pointcut-ref="showPointcut"/>
<aop:around method="aroundAdvice" pointcut-ref="showPointcut"/>
<aop:after method="afterAdvice" pointcut-ref="showPointcut"/>
</aop:aspect>
</aop:config>
```
最后,我们在bean包下定义一个Student类,实现show方法:
```java
package bean;
public class Student {
private String name;
private int age;
public void show() {
System.out.println("show()函数执行,输出信息:Name : zhangsan,Age: 30");
}
// getter和setter方法省略
}
```
现在,我们可以在main方法中调用show方法,观察输出:
```java
package bean;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Student student = (Student) context.getBean("student");
student.show();
}
}
```
输出如下:
```
< 前置通知运行
环绕通知开始运行
show()函数执行,输出信息:Name : zhangsan,Age: 30
环绕通知结束运行
后置通知运行
最终通知运行
```
阅读全文
相关推荐
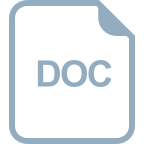
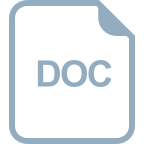
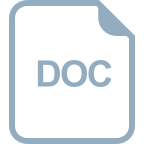

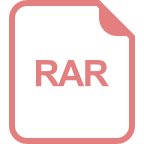
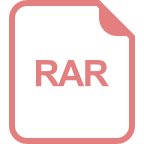
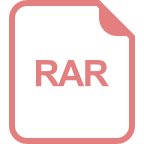
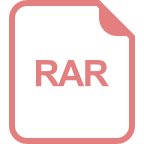
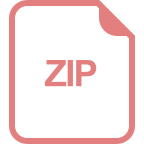
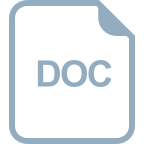
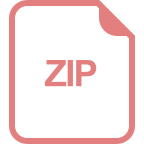
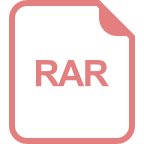
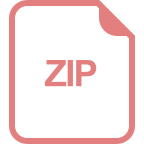
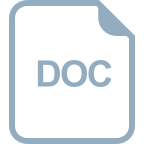



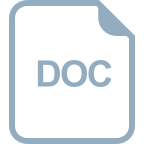
