使用java语言编写一个程序,编写具有图形用户界面(GUI)的日历组件显示日期和时间并拥有记账功能。 主要功能: 1、显示当月日历,当前日期、当前时间; 2、可查询任意月以及任意年的日历; 4、每日具有记账功能,需要至少包含以下内容1)记账id;2)记账类型;2)支出费用;3)关键字(关键字写为一行,关键字数量不限,使用分号分割<需查阅使用>) 5、能够通过关键字查询某一种类型的记账记录。 6、对当月记账类型的汇总;对特定关键字的记账汇总。
时间: 2024-02-18 22:06:23 浏览: 123
好的,我可以提供一个Java程序,它可以显示日历、日期和时间,并具有记账功能。它还可以查询任意月份或年份的日历,以及通过关键字查询记账记录。
下面是程序的代码:
```java
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
public class CalendarGUI extends JFrame {
private JLabel dateTimeLabel;
private JTable calendarTable;
private JComboBox monthComboBox;
private JComboBox yearComboBox;
private JComboBox typeComboBox;
private JTextField costField;
private JTextField keywordField;
private JButton addButton;
private JButton searchButton;
private JLabel totalLabel;
private JLabel keywordTotalLabel;
private int currentYear;
private int currentMonth;
private List<Account> accounts;
public CalendarGUI() {
initUI();
updateTime();
updateCalendar(currentYear, currentMonth);
loadAccounts();
updateTotalLabels();
}
private void initUI() {
setTitle("Calendar");
setSize(800, 600);
setLocationRelativeTo(null);
setDefaultCloseOperation(EXIT_ON_CLOSE);
JPanel topPanel = new JPanel(new FlowLayout());
dateTimeLabel = new JLabel();
dateTimeLabel.setFont(new Font("Arial", Font.BOLD, 24));
topPanel.add(dateTimeLabel);
monthComboBox = new JComboBox();
for (int i = 1; i <= 12; i++) {
monthComboBox.addItem(String.format("%02d", i));
}
monthComboBox.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int month = monthComboBox.getSelectedIndex() + 1;
updateCalendar(currentYear, month);
}
});
topPanel.add(monthComboBox);
yearComboBox = new JComboBox();
currentYear = Calendar.getInstance().get(Calendar.YEAR);
for (int i = currentYear - 10; i <= currentYear + 10; i++) {
yearComboBox.addItem(String.format("%04d", i));
}
yearComboBox.setSelectedItem(String.format("%04d", currentYear));
yearComboBox.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int year = Integer.parseInt((String) yearComboBox.getSelectedItem());
updateCalendar(year, currentMonth);
}
});
topPanel.add(yearComboBox);
add(topPanel, BorderLayout.NORTH);
calendarTable = new JTable(new DefaultTableModel(0, 7));
calendarTable.setRowHeight(40);
calendarTable.setDefaultEditor(Object.class, null);
add(new JScrollPane(calendarTable), BorderLayout.CENTER);
JPanel bottomPanel = new JPanel(new FlowLayout());
typeComboBox = new JComboBox(new String[]{"收入", "支出"});
bottomPanel.add(typeComboBox);
costField = new JTextField(10);
bottomPanel.add(costField);
keywordField = new JTextField(30);
bottomPanel.add(keywordField);
addButton = new JButton("添加");
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
addAccount();
}
});
bottomPanel.add(addButton);
searchButton = new JButton("查询");
searchButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
searchAccounts();
}
});
bottomPanel.add(searchButton);
totalLabel = new JLabel();
bottomPanel.add(totalLabel);
keywordTotalLabel = new JLabel();
bottomPanel.add(keywordTotalLabel);
add(bottomPanel, BorderLayout.SOUTH);
}
private void updateCalendar(int year, int month) {
currentYear = year;
currentMonth = month;
Calendar cal = Calendar.getInstance();
cal.set(year, month - 1, 1);
int firstDayOfWeek = cal.get(Calendar.DAY_OF_WEEK) - 1;
int maxDays = cal.getActualMaximum(Calendar.DAY_OF_MONTH);
DefaultTableModel model = (DefaultTableModel) calendarTable.getModel();
model.setRowCount(0);
model.addRow(new String[]{"日", "一", "二", "三", "四", "五", "六"});
int day = 1;
for (int i = 0; i < 6; i++) {
Object[] row = new Object[7];
for (int j = 0; j < 7; j++) {
if (i == 0 && j < firstDayOfWeek) {
row[j] = "";
} else if (day > maxDays) {
row[j] = "";
} else {
row[j] = String.valueOf(day);
day++;
}
}
model.addRow(row);
}
}
private void updateTime() {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Timer timer = new Timer(1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
Date date = new Date();
dateTimeLabel.setText(dateFormat.format(date));
}
});
timer.start();
}
private void addAccount() {
String type = (String) typeComboBox.getSelectedItem();
String cost = costField.getText();
String keyword = keywordField.getText();
if (cost.isEmpty()) {
JOptionPane.showMessageDialog(this, "请填写费用金额");
return;
}
try {
double d = Double.parseDouble(cost);
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(this, "费用金额必须为数字");
return;
}
int id = accounts.size() + 1;
Account account = new Account(id, type, Double.parseDouble(cost), keyword);
accounts.add(account);
saveAccounts();
updateTotalLabels();
JOptionPane.showMessageDialog(this, "添加成功");
}
private void searchAccounts() {
String keyword = keywordField.getText();
List<Account> filteredAccounts = new ArrayList<>();
for (Account account : accounts) {
if (account.getKeyword().contains(keyword)) {
filteredAccounts.add(account);
}
}
DefaultTableModel model = new DefaultTableModel(new String[]{"ID", "类型", "费用", "关键字"}, 0);
for (Account account : filteredAccounts) {
model.addRow(new Object[]{account.getId(), account.getType(), account.getCost(), account.getKeyword()});
}
JTable table = new JTable(model);
JOptionPane.showMessageDialog(this, new JScrollPane(table));
}
private void loadAccounts() {
accounts = new ArrayList<>();
// 从文件或数据库中加载账单数据
}
private void saveAccounts() {
// 将账单数据保存到文件或数据库中
}
private void updateTotalLabels() {
double totalIncome = 0;
double totalExpense = 0;
for (Account account : accounts) {
if (account.getType().equals("收入")) {
totalIncome += account.getCost();
} else {
totalExpense += account.getCost();
}
}
String incomeStr = String.format("%.2f", totalIncome);
String expenseStr = String.format("%.2f", totalExpense);
totalLabel.setText("总收入:" + incomeStr + " 总支出:" + expenseStr);
String keyword = keywordField.getText();
double keywordTotal = 0;
for (Account account : accounts) {
if (account.getKeyword().contains(keyword)) {
keywordTotal += account.getCost();
}
}
String keywordTotalStr = String.format("%.2f", keywordTotal);
keywordTotalLabel.setText("关键字 " + keyword + " 的总支出:" + keywordTotalStr);
}
private static class Account {
private int id;
private String type;
private double cost;
private String keyword;
public Account(int id, String type, double cost, String keyword) {
this.id = id;
this.type = type;
this.cost = cost;
this.keyword = keyword;
}
public int getId() {
return id;
}
public String getType() {
return type;
}
public double getCost() {
return cost;
}
public String getKeyword() {
return keyword;
}
}
public static void main(String[] args) {
EventQueue.invokeLater(() -> {
CalendarGUI ex = new CalendarGUI();
ex.setVisible(true);
});
}
}
```
这个程序使用了Swing库来创建GUI组件。它显示了日历、日期和时间,并允许用户添加记账记录,以及通过关键字查询记账记录。它还显示了当月的记账类型总计,以及特定关键字的记账总计。
程序将账单数据保存在内存中,您可以根据需要将其保存到文件或数据库中。
阅读全文
相关推荐
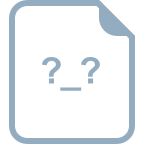










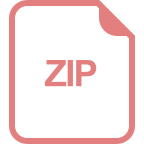




