写一个springboot 多线程调用接口请求的例子,并将所有接口请求的参数做聚合
时间: 2024-05-08 19:17:11 浏览: 12
首先,我们需要创建一个Controller类,用于接收前端请求,并开启多线程调用接口。
```
@RestController
public class AggregateController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/aggregate")
public String aggregate() {
List<Thread> threadList = new ArrayList<>();
for (int i = 0; i < 10; i++) {
Thread thread = new Thread(() -> {
// 调用接口
String result = restTemplate.getForObject("http://localhost:8080/api?param=" + i, String.class);
// 将结果保存到数据库或缓存中
saveResult(result);
});
threadList.add(thread);
thread.start();
}
// 等待所有线程执行完毕
for (Thread thread : threadList) {
try {
thread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// 从数据库或缓存中取出结果并做聚合
List<String> resultList = getResultList();
return String.join(",", resultList);
}
private void saveResult(String result) {
// 将结果保存到数据库或缓存中
}
private List<String> getResultList() {
// 从数据库或缓存中取出结果
return null;
}
}
```
在Controller中,我们使用了RestTemplate来调用接口,并开启了10个线程来并发调用接口。每个线程调用接口后将返回结果保存到数据库或缓存中,最后将所有结果取出并做聚合。
为了能够使用RestTemplate,我们需要在配置类中添加@Bean注解。
```
@Configuration
public class AppConfig {
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
```
最后,我们需要创建一个简单的接口来模拟请求。
```
@RestController
public class ApiController {
@GetMapping("/api")
public String api(@RequestParam String param) {
// 模拟请求
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "result-" + param;
}
}
```
在这个例子中,我们只是简单地模拟了一个请求,实际应用中需要根据具体情况进行修改。
总结:本例中使用了SpringBoot的多线程调用接口请求,并将所有接口请求的参数做聚合。开启多线程可以提高系统的并发处理能力,提高系统的性能。
相关推荐
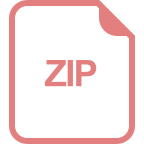
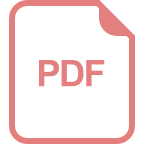
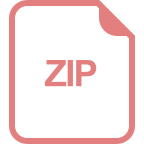














