初始化GD32串口1,开启接收和发送中断,并且接收中断里拉高引脚GPIOA,PIN1,发送字符0xFF
时间: 2023-11-28 19:06:03 浏览: 194
首先,需要配置串口1的参数,包括波特率、数据位、停止位等,然后开启串口1的接收和发送中断。
以下是初始化GD32串口1并开启中断的代码:
```c
// 定义串口1的接收缓冲区和发送缓冲区
#define USART1_RX_BUF_SIZE 256
#define USART1_TX_BUF_SIZE 256
// 定义串口1的接收缓冲区和发送缓冲区
volatile uint8_t usart1_rx_buf[USART1_RX_BUF_SIZE];
volatile uint8_t usart1_tx_buf[USART1_TX_BUF_SIZE];
// 定义串口1的接收缓冲区头指针和尾指针
volatile uint16_t usart1_rx_head = 0;
volatile uint16_t usart1_rx_tail = 0;
// 初始化串口1
void usart1_init(void)
{
// 使能串口1的时钟
rcu_periph_clock_enable(RCU_USART1);
// 配置串口1的GPIO引脚
gpio_af_set(GPIOA, GPIO_AF_1, GPIO_PIN_9 | GPIO_PIN_10);
// 配置串口1的参数
usart_deinit(USART1);
usart_baudrate_set(USART1, 115200);
usart_word_length_set(USART1, USART_WL_8BIT);
usart_stop_bit_set(USART1, USART_STB_1BIT);
usart_parity_config(USART1, USART_PM_NONE);
usart_hardware_flow_rts_config(USART1, USART_RTS_DISABLE);
usart_hardware_flow_cts_config(USART1, USART_CTS_DISABLE);
usart_receive_config(USART1, USART_RECEIVE_ENABLE);
usart_transmit_config(USART1, USART_TRANSMIT_ENABLE);
// 配置串口1中断
nvic_irq_enable(USART1_IRQn, 2, 0);
usart_interrupt_enable(USART1, USART_INT_TBE | USART_INT_RBNE);
// 使能串口1
usart_enable(USART1);
}
// 串口1中断服务函数
void USART1_IRQHandler(void)
{
if (RESET != usart_interrupt_flag_get(USART1, USART_INT_RBNE))
{
// 串口1接收中断
usart1_rx_buf[usart1_rx_head] = usart_data_receive(USART1);
usart1_rx_head = (usart1_rx_head + 1) % USART1_RX_BUF_SIZE;
// 拉高GPIOA,PIN1
gpio_bit_set(GPIOA, GPIO_PIN_1);
}
if (RESET != usart_interrupt_flag_get(USART1, USART_INT_TBE))
{
// 串口1发送中断
if (usart1_tx_head != usart1_tx_tail)
{
usart_data_transmit(USART1, usart1_tx_buf[usart1_tx_tail]);
usart1_tx_tail = (usart1_tx_tail + 1) % USART1_TX_BUF_SIZE;
}
else
{
// 发送完成,禁止发送中断
usart_interrupt_disable(USART1, USART_INT_TBE);
}
}
}
// 发送一个字符到串口1
void usart1_send_char(uint8_t ch)
{
uint16_t next_head = (usart1_tx_head + 1) % USART1_TX_BUF_SIZE;
while (next_head == usart1_tx_tail)
{
// 缓冲区已满,等待空闲
}
usart1_tx_buf[usart1_tx_head] = ch;
usart1_tx_head = next_head;
usart_interrupt_enable(USART1, USART_INT_TBE); // 使能发送中断
}
// 发送0xFF到串口1
usart1_send_char(0xFF);
```
在上面的代码中,我们定义了一个接收缓冲区和一个发送缓冲区,以及两个指针用于指向缓冲区的头和尾。在串口1接收中断服务函数中,我们将接收到的数据存入接收缓冲区,并将头指针往后移动一个位置,同时拉高GPIOA,PIN1。在串口1发送中断服务函数中,我们从发送缓冲区取出一个字符发送出去,如果发送缓冲区已经空了,就禁止发送中断。最后,我们定义了一个发送一个字符的函数,用于发送0xFF到串口1。
阅读全文
相关推荐
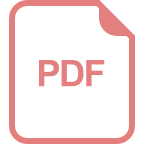
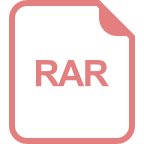













