帮我写一个GD32串口接收中断
时间: 2023-08-05 20:07:09 浏览: 133
当然可以帮你写一个GD32串口接收中断的代码。以下是一个示例:
```c
#include "gd32f10x.h"
#include <stdio.h>
#define USART0_BUFFER_SIZE 100
volatile uint8_t usart0_rx_buffer[USART0_BUFFER_SIZE];
volatile uint32_t usart0_rx_index = 0;
void usart0_init(void)
{
/* 使能串口时钟 */
rcu_periph_clock_enable(RCU_USART0);
/* 配置USART0引脚 */
gpio_init(GPIOA, GPIO_MODE_AF_PP, GPIO_OSPEED_50MHZ, GPIO_PIN_9); // TX
gpio_init(GPIOA, GPIO_MODE_IN_FLOATING, GPIO_OSPEED_50MHZ, GPIO_PIN_10); // RX
/* 配置USART0参数 */
usart_deinit(USART0);
usart_baudrate_set(USART0, 115200);
usart_word_length_set(USART0, USART_WL_8BIT);
usart_stop_bit_set(USART0, USART_STB_1BIT);
usart_parity_config(USART0, USART_PM_NONE);
usart_hardware_flow_rts_config(USART0, USART_RTS_DISABLE);
usart_hardware_flow_cts_config(USART0, USART_CTS_DISABLE);
usart_receive_config(USART0, USART_RECEIVE_ENABLE);
usart_interrupt_enable(USART0, USART_INT_RBNE);
/* 使能串口 */
usart_enable(USART0);
}
void USART0_IRQHandler(void)
{
if (usart_interrupt_flag_get(USART0, USART_INT_FLAG_RBNE))
{
/* 读取接收数据 */
uint8_t data = usart_data_receive(USART0);
/* 将接收到的数据存入缓冲区 */
usart0_rx_buffer[usart0_rx_index++] = data;
/* 缓冲区溢出处理 */
if (usart0_rx_index >= USART0_BUFFER_SIZE)
{
usart0_rx_index = 0;
}
}
}
int main(void)
{
/* 初始化串口 */
usart0_init();
while (1)
{
/* 在此处添加你的代码逻辑 */
}
}
```
这段代码实现了GD32的USART0串口接收中断功能。在中断处理函数`USART0_IRQHandler`中,当接收到数据时,将数据存入缓冲区`usart0_rx_buffer`中,并递增索引`usart0_rx_index`。你可以在主循环中添加自己的代码逻辑来处理接收到的数据。
请确保在使用之前,已经正确配置了GD32的时钟和GPIO引脚,以及启用了相应的中断。同时,根据你的需求,可能需要调整波特率、数据位数、停止位等串口参数。
阅读全文
相关推荐
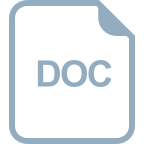
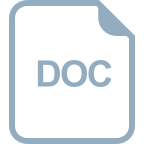
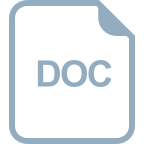
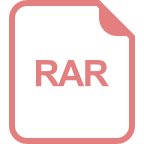
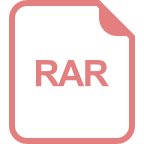








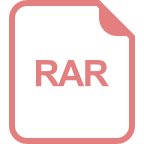




