通过Carla模拟器,设置车的速度
时间: 2023-10-01 13:06:37 浏览: 1012
要设置车的速度,需要在Carla模拟器中先创建一个车辆。在创建车辆之后,可以使用以下代码将车速设置为指定的值:
```
import carla
# 建立连接
client = carla.Client('localhost', 2000)
client.set_timeout(2.0)
# 获取世界
world = client.get_world()
# 创建车辆
vehicle_bp = world.get_blueprint_library().find('vehicle.tesla.model3')
spawn_point = carla.Transform(carla.Location(x=50, y=50, z=2), carla.Rotation())
vehicle = world.spawn_actor(vehicle_bp, spawn_point)
# 设置速度
vehicle.set_target_velocity(carla.Vector3D(x=20, y=0, z=0))
```
在这个例子中,我们使用了Tesla Model 3作为车辆蓝图,并将车辆生成在世界坐标系中`(x=50, y=50, z=2)`的位置。最后,我们将车速设置为20m/s。
相关问题
如何在carla驾驶模拟器的python文件中找到word中所有车辆储存的位置以及这些车对应的驾驶数据
要找到Carla驾驶模拟器中所有车辆的位置和对应的驾驶数据,可以使用Carla Python API中提供的函数和类。以下是一个简单的示例代码,可以获取所有车辆的位置和速度信息:
```python
import carla
# 连接到Carla服务器
client = carla.Client('localhost', 2000)
client.set_timeout(2.0)
# 获取世界对象
world = client.get_world()
# 获取所有车辆
vehicles = world.get_actors().filter('vehicle.*')
# 遍历所有车辆,获取位置和速度信息
for vehicle in vehicles:
location = vehicle.get_location()
velocity = vehicle.get_velocity()
print(f"Vehicle {vehicle.id} is at {location} with velocity {velocity}")
```
要获取车辆的驾驶数据,需要使用车辆的控制器对象。下面的示例代码演示了如何获取所有车辆的控制器并获取其转向和加速度:
```python
import carla
# 连接到Carla服务器
client = carla.Client('localhost', 2000)
client.set_timeout(2.0)
# 获取世界对象
world = client.get_world()
# 获取所有车辆
vehicles = world.get_actors().filter('vehicle.*')
# 遍历所有车辆,获取控制器信息
for vehicle in vehicles:
# 获取控制器对象
controller = vehicle.get_control()
# 获取转向和加速度信息
steer = controller.steer
throttle = controller.throttle
brake = controller.brake
print(f"Vehicle {vehicle.id} has steer={steer}, throttle={throttle}, brake={brake}")
```
注意,以上代码只是一个简单示例,您可以根据您的需求进行更改和扩展。
如何在carla中设置4个NPC车辆,并将这4个NPC车辆的实时速度与玩家车辆之间的距离放在一个新的csv表里
要在CARLA中设置4个NPC车辆并将它们的实时速度和与玩家车辆之间的距离放在一个新的CSV表中,您可以执行以下步骤:
1. 在CARLA模拟器中打开一个新的场景,并将玩家车辆放置在其中。
2. 使用Python API创建4个NPC车辆并将它们放置在场景中。
3. 使用CARLA的Python API获取玩家车辆和4个NPC车辆的实时速度和位置信息。
4. 计算每个NPC车辆与玩家车辆之间的距离。
5. 将速度和距离信息存储在一个新的CSV文件中。
以下是示例代码,可以帮助您完成这项任务:
``` python
import carla
import csv
# Connect to the CARLA simulator
client = carla.Client('localhost', 2000)
client.set_timeout(10.0)
world = client.get_world()
# Create a blueprint of a vehicle
vehicle_bp = world.get_blueprint_library().find('vehicle.tesla.model3')
# Spawn the player vehicle
player_transform = carla.Transform(carla.Location(x=50, y=50, z=2), carla.Rotation(yaw=0))
player_vehicle = world.spawn_actor(vehicle_bp, player_transform)
# Spawn the NPC vehicles
npc_transforms = [
carla.Transform(carla.Location(x=100, y=50, z=2), carla.Rotation(yaw=0)),
carla.Transform(carla.Location(x=50, y=100, z=2), carla.Rotation(yaw=90)),
carla.Transform(carla.Location(x=0, y=50, z=2), carla.Rotation(yaw=180)),
carla.Transform(carla.Location(x=50, y=0, z=2), carla.Rotation(yaw=270)),
]
npc_vehicles = []
for transform in npc_transforms:
npc_vehicle = world.spawn_actor(vehicle_bp, transform)
npc_vehicles.append(npc_vehicle)
# Open a new CSV file
csv_file = open('speed_distance.csv', 'w', newline='')
csv_writer = csv.writer(csv_file)
# Loop to get the speed and distance data
while True:
# Get the player vehicle's speed and location
player_speed = player_vehicle.get_velocity().x
player_location = player_vehicle.get_location()
# Loop through each NPC vehicle
for i, npc_vehicle in enumerate(npc_vehicles):
# Get the NPC vehicle's speed and location
npc_speed = npc_vehicle.get_velocity().x
npc_location = npc_vehicle.get_location()
# Calculate the distance between the player and NPC vehicle
distance = player_location.distance(npc_location)
# Write the data to the CSV file
csv_writer.writerow([i, npc_speed, distance, player_speed])
# Wait for a short time before getting the next data point
world.wait_for_tick()
# Close the CSV file and clean up the actors
csv_file.close()
for vehicle in npc_vehicles + [player_vehicle]:
vehicle.destroy()
```
此代码可以在CARLA中设置4个NPC车辆,计算它们和玩家车之间的距离,并将数据写入新的CSV文件中。
阅读全文
相关推荐
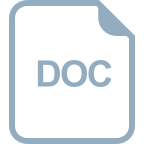
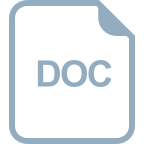
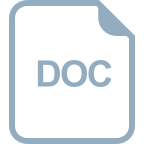
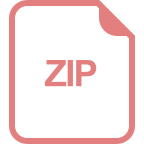
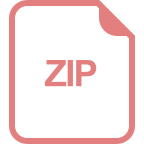
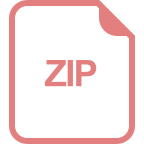
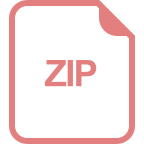
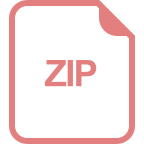
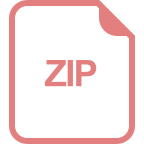
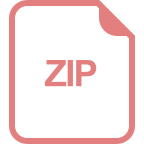
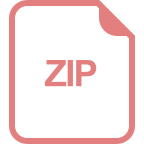
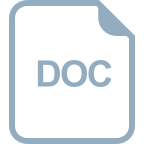
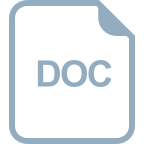
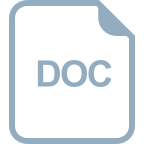
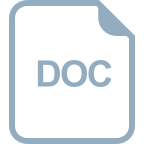
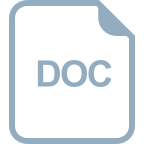
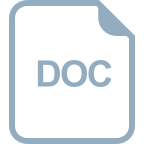