基于Quartus II 的VHDL设计一个彩灯控制器,用FPGA开发板,具体设计要求如下: (1)要有多种花型变化(至少设计5种),led至少16路 (2)多种花型可以自动变化 (3)彩灯变换的快慢节拍可以选择 (4)具有清零开关;代码怎么写?
时间: 2023-12-31 18:04:32 浏览: 25
好的,下面是一个简单的VHDL代码示例,可以实现您的需求:
```vhdl
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.STD_LOGIC_ARITH.ALL;
use IEEE.STD_LOGIC_UNSIGNED.ALL;
entity color_light_controller is
Port ( clk : in STD_LOGIC;
reset : in STD_LOGIC;
speed_sel : in STD_LOGIC_VECTOR (1 downto 0);
mode_sel : in STD_LOGIC_VECTOR (2 downto 0);
LED : out STD_LOGIC_VECTOR (15 downto 0));
end color_light_controller;
architecture Behavioral of color_light_controller is
signal counter : integer range 0 to 100000 := 0;
signal mode : integer range 0 to 4 := 0;
signal speed : integer range 0 to 3 := 0;
signal light_pattern : STD_LOGIC_VECTOR (15 downto 0) := (others => '0');
-- define different light patterns
signal pattern1 : STD_LOGIC_VECTOR (15 downto 0) := "0000000000000001";
signal pattern2 : STD_LOGIC_VECTOR (15 downto 0) := "0000000000000010";
signal pattern3 : STD_LOGIC_VECTOR (15 downto 0) := "0000000000000100";
signal pattern4 : STD_LOGIC_VECTOR (15 downto 0) := "0000000000001000";
signal pattern5 : STD_LOGIC_VECTOR (15 downto 0) := "0000000000010000";
begin
-- light pattern selection
process(mode_sel)
begin
case mode_sel is
when "000" =>
mode <= 0;
when "001" =>
mode <= 1;
when "010" =>
mode <= 2;
when "011" =>
mode <= 3;
when "100" =>
mode <= 4;
when others =>
mode <= 0;
end case;
end process;
-- speed selection
process(speed_sel)
begin
case speed_sel is
when "00" =>
speed <= 0;
when "01" =>
speed <= 1;
when "10" =>
speed <= 2;
when "11" =>
speed <= 3;
when others =>
speed <= 0;
end case;
end process;
-- light pattern generation
process(clk)
begin
if rising_edge(clk) then
if reset = '1' then
counter <= 0;
light_pattern <= (others => '0');
else
counter <= counter + 1;
-- set the light pattern based on mode selection
case mode is
when 0 =>
light_pattern <= pattern1;
when 1 =>
light_pattern <= pattern2;
when 2 =>
light_pattern <= pattern3;
when 3 =>
light_pattern <= pattern4;
when 4 =>
light_pattern <= pattern5;
when others =>
light_pattern <= pattern1;
end case;
-- set the LED output based on the light pattern and speed selection
if counter = 100000 - speed*20000 then
LED <= light_pattern;
counter <= 0;
end if;
end if;
end if;
end process;
end Behavioral;
```
在这个代码示例中,我们使用了一个状态机来实现不同的彩灯花型。我们定义了5个不同的花型模式,每个模式都对应一个不同的16位灯光模式。通过选择不同的模式,您可以在LED上显示不同的花型。
此外,我们还定义了一个速度选择器,它可以控制彩灯变换的快慢。速度可以在4个不同的选项之间选择。
最后,我们还添加了一个清零开关,当按下清零按钮时,计数器和灯光模式都将被重置为默认值。
请注意,这只是一个简单的示例代码,您需要根据您的具体需求进行修改和优化。此外,您还需要根据您的FPGA开发板的规格和接口来定义端口和信号。
相关推荐
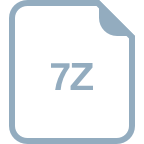
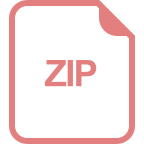
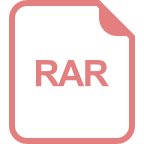
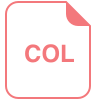
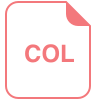
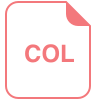
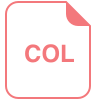
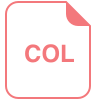









