Java 实现文件hash 去重
时间: 2024-05-05 19:21:41 浏览: 86
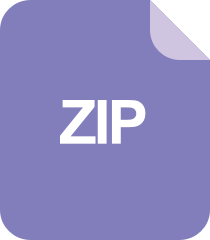
Java实现根据文件哈希值和文件大小,删除重复文件

文件的 hash 值可以通过 Java 的 MessageDigest 类来计算,具体实现步骤如下:
1. 读取文件内容,可以使用 FileInputStream 类来读取文件;
2. 使用 MessageDigest 类来计算文件内容的 hash 值;
3. 将 hash 值转换为十六进制字符串,可以使用 BigInteger 类来实现;
4. 将每个文件的 hash 值存储到一个 Set 集合中,用于判断文件是否重复。
以下是一个示例代码:
```java
import java.io.File;
import java.io.FileInputStream;
import java.math.BigInteger;
import java.security.MessageDigest;
import java.util.HashSet;
import java.util.Set;
public class FileHashDeduplication {
public static void main(String[] args) {
String directoryPath = "C:/path/to/directory";
Set<String> hashSet = new HashSet<>();
File directory = new File(directoryPath);
if (directory.isDirectory()) {
File[] files = directory.listFiles();
if (files != null) {
for (File file : files) {
try {
String hash = getFileHash(file);
if (!hashSet.contains(hash)) {
hashSet.add(hash);
// do something with the file
System.out.println(file.getPath());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
}
private static String getFileHash(File file) throws Exception {
MessageDigest messageDigest = MessageDigest.getInstance("MD5");
FileInputStream fileInputStream = new FileInputStream(file);
byte[] buffer = new byte[1024];
int len;
while ((len = fileInputStream.read(buffer)) != -1) {
messageDigest.update(buffer, 0, len);
}
fileInputStream.close();
BigInteger bigInteger = new BigInteger(1, messageDigest.digest());
return bigInteger.toString(16);
}
}
```
在上面的代码中,getFileHash() 方法用于计算文件的 hash 值,使用 MD5 算法来计算,计算出的结果以十六进制字符串的形式返回。getFileHash() 方法中的代码可以自行修改,例如可以使用 SHA-256 算法来计算文件的 hash 值。使用 Set 集合来判断文件是否重复,如果集合中已经包含了该文件的 hash 值,则说明该文件已经存在,否则将该文件的 hash 值添加到集合中。
阅读全文
相关推荐
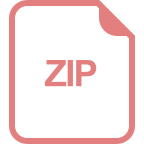
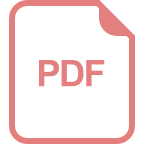

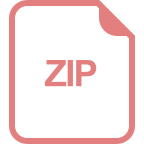
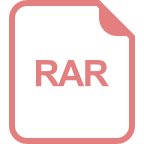
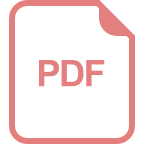
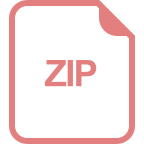
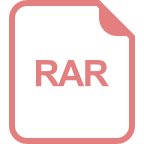
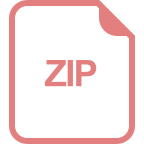
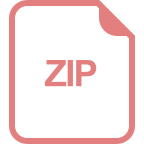
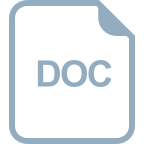
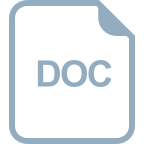
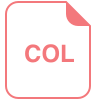
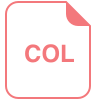
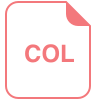
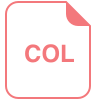
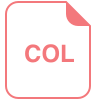
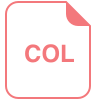