用一个模板实现对栈的操作,栈的数据结构由链表实现。实现栈的功能: 进栈、出栈和清空的功能。并在 main()函数中实现对 int 型栈和 struct CHAPA 型栈 (定义如下)的基本操作。 //--------------------------------------------------------------------------------------------------- struct CHAPA { char *pName; bool Gender; int Age; struct CHAPA *next; }; //---------------------------------------------------------------------------------------------------
时间: 2023-08-15 16:18:03 浏览: 75
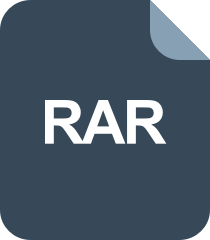
数据结构 栈 链表实现 c++ 模板
好的,以下是模板实现对链表实现的栈的基本操作的代码:
```c++
#include <iostream>
using namespace std;
template <class T>
class Stack {
private:
struct Node {
T data;
Node* next;
Node(T d):data(d), next(nullptr){};
};
Node* top_ptr;
public:
Stack(): top_ptr(nullptr){};
bool isEmpty() {
return top_ptr == nullptr;
}
void push(T data) {
Node* new_ptr = new Node(data);
new_ptr->next = top_ptr;
top_ptr = new_ptr;
}
T pop() {
if(isEmpty()) {
throw runtime_error("Stack is empty!");
}
T ret_data = top_ptr->data;
Node* temp = top_ptr;
top_ptr = top_ptr->next;
delete temp;
return ret_data;
}
void clear() {
while(!isEmpty()) {
pop();
}
}
};
struct CHAPA {
char *pName;
bool Gender;
int Age;
struct CHAPA *next;
};
int main() {
// test for int stack
Stack<int> int_stack;
int_stack.push(1);
int_stack.push(2);
int_stack.push(3);
cout << int_stack.pop() << endl;
cout << int_stack.pop() << endl;
int_stack.clear();
cout << int_stack.isEmpty() << endl;
// test for CHAPA stack
Stack<CHAPA*> chapa_stack;
CHAPA* chapa1 = new CHAPA;
chapa1->pName = new char[10];
chapa1->pName = "chapa1";
chapa1->Gender = true;
chapa1->Age = 20;
chapa_stack.push(chapa1);
CHAPA* chapa2 = new CHAPA;
chapa2->pName = new char[10];
chapa2->pName = "chapa2";
chapa2->Gender = true;
chapa2->Age = 21;
chapa_stack.push(chapa2);
CHAPA* chapa3 = new CHAPA;
chapa3->pName = new char[10];
chapa3->pName = "chapa3";
chapa3->Gender = false;
chapa3->Age = 22;
chapa_stack.push(chapa3);
cout << chapa_stack.pop()->pName << endl;
cout << chapa_stack.pop()->pName << endl;
chapa_stack.clear();
cout << chapa_stack.isEmpty() << endl;
return 0;
}
```
这里用了 C++ 的模板来实现栈的操作,同时用了链表来作为栈的数据结构。而对于 `struct CHAPA` 这个结构体,我们在实现时使用了指向它的指针 `CHAPA*`,并在操作时需要注意使用 `->` 来访问其成员。
阅读全文
相关推荐


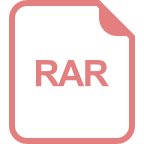
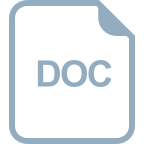
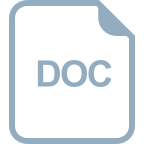
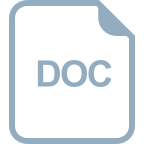
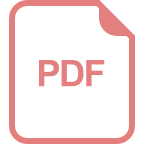
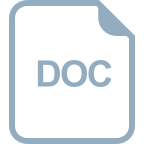
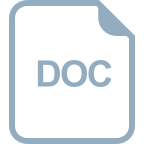
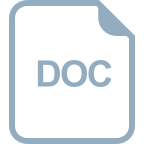
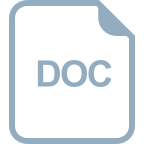
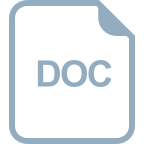
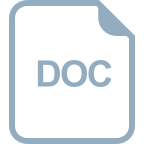
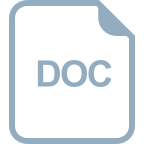
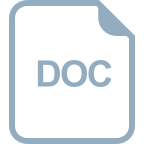
