C语言请你实现用链表结构完成栈的入栈、出栈,队列的入队和出队操作
时间: 2024-05-08 19:18:21 浏览: 134
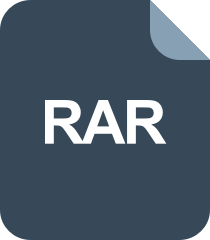
c语言实现链表的基本操作,链表是一种物理存储单元上非连续、非顺序的存储结构,数据元素的逻辑顺序是通过链表中的指针链接次序实现的。
好的,我可以为您提供一份 C 语言代码示例,用链表实现栈和队列。在下面的代码中,我们定义了一个结构体 ListNode,它有一个整数成员 data 和一个指向下一个节点的指针 next。我们还定义了两个指针 top 和 tail,用于维护栈和队列的头部和尾部:
```
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点的结构体
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
// 初始化栈和队列的头部和尾部指针
ListNode* stack_top = NULL;
ListNode* queue_head = NULL;
ListNode* queue_tail = NULL;
// 入栈操作
void push(int value) {
// 创建一个新的节点
ListNode* node = (ListNode*) malloc(sizeof(ListNode));
node->data = value;
node->next = stack_top;
stack_top = node;
}
// 出栈操作
int pop() {
if (stack_top == NULL) {
printf("Error: stack is empty.\n");
return -1;
}
int value = stack_top->data;
ListNode* temp = stack_top;
stack_top = stack_top->next;
free(temp);
return value;
}
// 入队操作
void enqueue(int value) {
// 创建一个新的节点
ListNode* node = (ListNode*) malloc(sizeof(ListNode));
node->data = value;
node->next = NULL;
if (queue_head == NULL) {
queue_head = node;
queue_tail = node;
} else {
queue_tail->next = node;
queue_tail = node;
}
}
// 出队操作
int dequeue() {
if (queue_head == NULL) {
printf("Error: queue is empty.\n");
return -1;
}
int value = queue_head->data;
ListNode* temp = queue_head;
queue_head = queue_head->next;
if (queue_head == NULL) {
queue_tail = NULL;
}
free(temp);
return value;
}
// 测试代码
int main() {
// 入栈操作
push(1);
push(2);
push(3);
// 出栈操作
printf("%d\n", pop()); // 3
printf("%d\n", pop()); // 2
printf("%d\n", pop()); // 1
printf("%d\n", pop()); // Error: stack is empty.
// 入队操作
enqueue(1);
enqueue(2);
enqueue(3);
// 出队操作
printf("%d\n", dequeue()); // 1
printf("%d\n", dequeue()); // 2
printf("%d\n", dequeue()); // 3
printf("%d\n", dequeue()); // Error: queue is empty.
return 0;
}
```
以上便是用链表实现栈和队列的代码示例,希望能对您有所帮助。
阅读全文
相关推荐
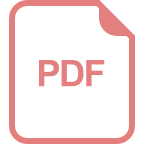
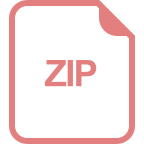
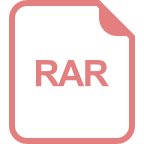
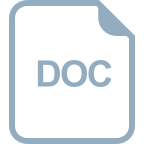
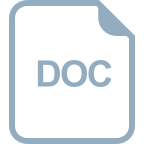
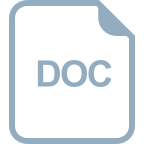
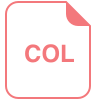


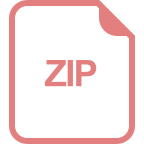
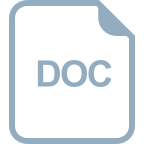
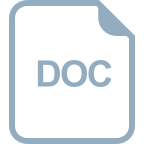
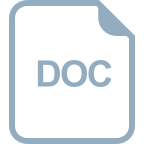
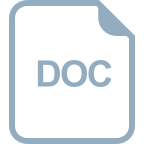
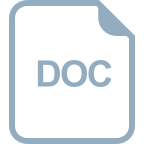
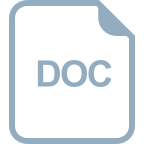
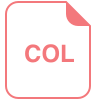