使用贪心算法求解Huffman编码问题,要求输出带权路径值,利用C++写
时间: 2023-08-04 20:26:11 浏览: 95
好的,下面是使用贪心算法求解Huffman编码问题的C++代码,包括输出带权路径值:
```c++
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
struct TreeNode {
int val;
TreeNode *left;
TreeNode *right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
struct cmp {
bool operator()(TreeNode *a, TreeNode *b) {
return a->val > b->val;
}
};
int Huffman(TreeNode *root, int depth) {
if (!root) {
return 0;
}
if (!root->left && !root->right) {
return root->val * depth;
}
return Huffman(root->left, depth + 1) + Huffman(root->right, depth + 1);
}
int main() {
int n;
cin >> n;
priority_queue<TreeNode *, vector<TreeNode *>, cmp> pq;
for (int i = 0; i < n; i++) {
int val;
cin >> val;
pq.push(new TreeNode(val));
}
while (pq.size() > 1) {
TreeNode *left = pq.top();
pq.pop();
TreeNode *right = pq.top();
pq.pop();
TreeNode *node = new TreeNode(left->val + right->val);
node->left = left;
node->right = right;
pq.push(node);
}
cout << Huffman(pq.top(), 0) << endl;
return 0;
}
```
其中,`cmp`是定义优先队列比较的结构体,`Huffman`是递归求解带权路径值的函数,`main`函数中首先读入权值,然后使用优先队列来构建Huffman树,最后输出带权路径值。
阅读全文
相关推荐
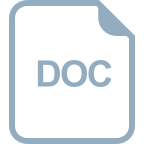
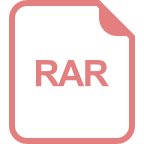


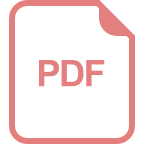
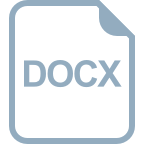
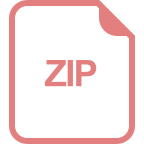
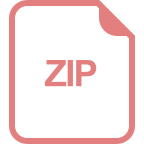
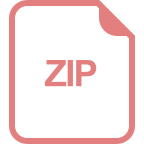
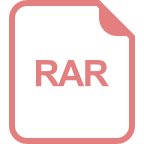
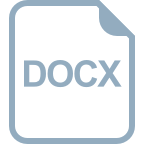
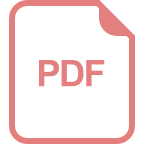
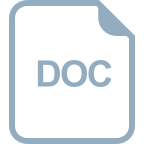
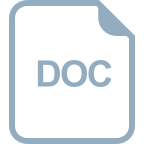
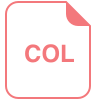
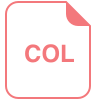