词法分析程序详细实现 python
时间: 2023-05-13 16:01:04 浏览: 74
词法分析是编译器的第一步,也是最基础的处理过程,它的主要作用是将程序代码中的字符序列转换为单词序列,在编译器编译过程中起到了非常重要的作用。在Python中,我们可以采用Lex yacc工具来实现词法分析。
Python中的Lex工具是一个生成词法分析器的工具,它可以根据用户所输入的规则,生成词法分析器的代码。这些规则是用正则表达式形式描述的,词法分析器会根据这些规则扫描源程序代码,将其转换成代表程序中各种构造的单词序列。
在Python中实现词法分析器,首先需要安装好对应的Lex程序。在设置好工作目录后,我们可以在命令提示符输入“lex”命令,根据安装包提示进行相关设置。
然后,我们需要定义程序中所使用的关键字、操作符、标识符等信息,以便词法分析器能够正确识别源程序中的各种符号。定义完成后,词法分析器会自动生成对应的转换代码,将源程序的字符序列转换为单词序列。
接着,我们需要创建一个词法分析器的主程序,读入源程序代码并进行词法分析。主程序可以采用Python自带的input函数读取用户输入的代码字符串,然后调用词法分析器进行处理。
在对源程序进行词法分析时,我们可以用Python中的Lexer类来实现。该类的主要作用是将程序代码转换为单词序列,其中包括每个单词的类型和值等相关信息。Lexer类还可以自定义输出模板,输出词法分析结果。
总体来说,在Python中实现词法分析程序的过程较为简单,只需在Lex程序中定义关键字、符号等信息,生成词法分析器代码,然后在Python中调用Lexer类进行处理即可。
相关问题
python词法分析程序设计
Python词法分析程序设计是指设计和实现一个能够将Python代码进行词法分析的程序。词法分析是编译器的第一个阶段,它将源代码分解成一个个的词法单元(token),并生成一个词法单元流。
在Python中,词法分析器通常使用正则表达式来匹配和提取不同的词法单元。以下是Python词法分析程序设计的一般步骤:
1. 定义词法规则:根据Python语法规范,定义不同的词法规则,如标识符、关键字、运算符、常量等。
2. 使用正则表达式匹配:使用正则表达式来匹配源代码中的不同词法单元。每个词法规则对应一个正则表达式模式。
3. 生成词法单元流:根据匹配结果,将源代码分解成一个个的词法单元,并生成一个词法单元流。
4. 返回词法单元流:将生成的词法单元流返回给调用者,供后续的语法分析和语义分析使用。
Python中有一些常用的工具和库可以帮助进行词法分析程序设计,如ply(Python Lex-Yacc)、re模块等。
自己设计词法分析程序实现
词法分析器是编译器中的一个重要组成部分,可以将源代码分解成一个个单独的符号(token)并为每个符号指定一个类型。下面是一个简单的词法分析器的实现示例:
```python
import re
# 定义关键字
keywords = ['if', 'else', 'while', 'for', 'int', 'float', 'double', 'char']
# 定义符号
symbols = ['(', ')', '{', '}', ';', ',', '+', '-', '*', '/']
# 定义正则表达式
regex = {
'identifier': '^[a-zA-Z_][a-zA-Z0-9_]*$',
'number': '^(0|[1-9][0-9]*)$',
'operator': '^(\+|\-|\*|\/)$',
}
# 定义词法分析函数
def lex(text):
tokens = []
i = 0
while i < len(text):
# 跳过空格
if text[i].isspace():
i += 1
continue
# 匹配关键字或标识符
if text[i].isalpha() or text[i] == '_':
j = i + 1
while j < len(text) and (text[j].isalnum() or text[j] == '_'):
j += 1
token = text[i:j]
if token in keywords:
tokens.append((token, 'keyword'))
else:
match = re.match(regex['identifier'], token)
if match:
tokens.append((token, 'identifier'))
else:
raise ValueError(f"Invalid identifier: {token}")
i = j
continue
# 匹配数字
if text[i].isdigit():
j = i + 1
while j < len(text) and text[j].isdigit():
j += 1
token = text[i:j]
match = re.match(regex['number'], token)
if match:
tokens.append((token, 'number'))
else:
raise ValueError(f"Invalid number: {token}")
i = j
continue
# 匹配符号
if text[i] in symbols:
tokens.append((text[i], 'symbol'))
i += 1
continue
# 匹配运算符
if text[i] in ['+', '-', '*', '/']:
match = re.match(regex['operator'], text[i])
if match:
tokens.append((text[i], 'operator'))
i += 1
continue
else:
raise ValueError(f"Invalid operator: {text[i]}")
# 无法识别的字符
raise ValueError(f"Invalid character: {text[i]}")
return tokens
```
该词法分析器支持识别关键字、标识符、数字、符号和运算符,并使用正则表达式进行匹配。在词法分析过程中,词法分析器会逐个扫描源代码中的字符,并根据字符的类型将其归类为不同的符号类型,并返回一个符号序列。
下面是一个使用该词法分析器的示例:
```python
text = """
int main() {
int a = 1;
int b = 2;
int c = a + b;
return c;
}
"""
tokens = lex(text)
for token in tokens:
print(token)
```
输出结果如下:
```
('int', 'keyword')
('main', 'identifier')
('(', 'symbol')
(')', 'symbol')
('{', 'symbol')
('int', 'keyword')
('a', 'identifier')
('=', 'symbol')
('1', 'number')
(';', 'symbol')
('int', 'keyword')
('b', 'identifier')
('=', 'symbol')
('2', 'number')
(';', 'symbol')
('int', 'keyword')
('c', 'identifier')
('=', 'symbol')
('a', 'identifier')
('+', 'operator')
('b', 'identifier')
(';', 'symbol')
('return', 'keyword')
('c', 'identifier')
(';', 'symbol')
('}', 'symbol')
```
相关推荐
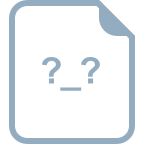
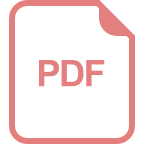
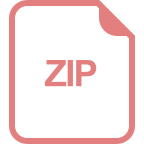












