QT C++QT的QCombobox如何只显示一个文字,不根据选择变化
时间: 2024-03-09 19:48:39 浏览: 20
如果需要将QComboBox只显示一个固定的文字,而不受选择影响,可以使用setItemText函数来设置显示的文字,这样无论选择哪个选项,都会显示设置的文字。示例代码如下:
```cpp
#include <QApplication>
#include <QComboBox>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
QComboBox combo_box;
combo_box.addItem("选项1");
combo_box.addItem("选项2");
combo_box.addItem("选项3");
combo_box.setCurrentIndex(0);
combo_box.setItemText(0, "固定文字");
combo_box.show();
return app.exec();
}
```
在上面的示例代码中,我们创建了一个QComboBox,将其选项设置为选项1、选项2和选项3,并将默认选中的选项设置为选项1。然后,使用setItemText函数将第一个选项的文字设置为固定文字,这样无论选择哪个选项,都会显示固定文字。这样就能实现一个QComboBox只显示一个固定的文字,而不受选择影响的效果。
相关问题
Qt c++QComboBox 居中显示
要在Qt C++中将QComboBox下拉列表中的文本居中显示,可以使用以下代码实现:
```cpp
void setComboBoxTextCenter(QComboBox *comboBox)
{
QStandardItemModel *model = qobject_cast<QStandardItemModel *>(comboBox->model());
if (model) {
for (int i = 0; i < model->rowCount(); ++i) {
QModelIndex index = model->index(i, comboBox->modelColumn(), comboBox->rootModelIndex());
QStandardItem *item = model->itemFromIndex(index);
item->setTextAlignment(Qt::AlignCenter);
}
}
}
```
然后在需要居中显示的QComboBox对象上调用该函数即可:
```cpp
QComboBox *comboBox = new QComboBox;
// 添加选项...
setComboBoxTextCenter(comboBox);
```
qt c++ 点击QComboBox控件中的其中一个元素后弹出文本对话框
您可以通过连接QComboBox的currentIndexChanged信号来实现点击QComboBox控件中的某个元素后弹出文本对话框。以下是一个示例代码:
首先,在您的头文件中声明一个槽函数用于处理currentIndexChanged信号:
```cpp
private slots:
void showTextDialog();
```
然后,在您的源文件中实现该槽函数:
```cpp
void YourClass::showTextDialog()
{
// 获取选中的文本
QString selectedText = comboBox->currentText();
// 创建一个文本对话框
QString inputText = QInputDialog::getText(this, "输入文本", "请输入文本:");
// 在此处可以根据需要处理输入的文本
// ...
// 在此处可以根据需要显示对话框中的文本
// ...
}
```
接下来,在您的构造函数或初始化函数中,将该槽函数与QComboBox的currentIndexChanged信号连接起来:
```cpp
// 创建一个QComboBox对象
comboBox = new QComboBox(this);
// 添加选项
comboBox->addItem("选项1");
comboBox->addItem("选项2");
comboBox->addItem("选项3");
// 连接currentIndexChanged信号与槽函数
connect(comboBox, QOverload<int>::of(&QComboBox::currentIndexChanged), this, &YourClass::showTextDialog);
```
当用户点击QComboBox控件中的某个元素时,会触发currentIndexChanged信号,槽函数showTextDialog将被调用,然后弹出一个文本对话框,用户可以在对话框中输入文本。您可以根据需要在槽函数中处理输入的文本并进行相关操作。
相关推荐
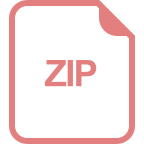












