qt 生成随机数
时间: 2023-07-05 14:10:38 浏览: 57
在 Qt 中生成随机数,可以使用 QRandomGenerator 类。以下是生成随机整数和实数的示例代码:
```cpp
// 生成随机整数
int randomInt = QRandomGenerator::global()->bounded(100); // 生成 0-99 之间的整数
// 生成随机实数
double randomReal = QRandomGenerator::global()->generateDouble(); // 生成 0-1 之间的实数
```
注意,在使用 QRandomGenerator 生成随机数之前,需要先调用 `qsrand` 函数进行初始化:
```cpp
QRandomGenerator::global()->seed(QDateTime::currentMSecsSinceEpoch() / 1000); // 初始化随机数生成器
```
这里使用了当前时间作为种子,可以保证每次生成的随机数序列都不同。
相关问题
QT 生成随机数 浮点数
您可以使用Qt的QRandomGenerator类来生成随机数。对于浮点数,您可以使用uniformReal函数。下面是一个示例代码:
```cpp
#include <QCoreApplication>
#include <QDebug>
#include <QRandomGenerator>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 设置种子,通常不需要手动设置,系统会自动设置种子
QRandomGenerator::global()->seed(QDateTime::currentMSecsSinceEpoch() / 1000);
// 生成一个0到1之间的随机浮点数
double randomValue = QRandomGenerator::global()->generateDouble();
qDebug() << "随机浮点数:" << randomValue;
return a.exec();
}
```
这段代码会生成一个0到1之间的随机浮点数,并输出到调试窗口。您可以根据需要调整生成的范围或精度。
qt现实生成随机数并显示在控件上
您好!关于您的问题,可以通过Qt自带的QRandomGenerator类生成随机数,并将其显示在控件上。具体实现可参考以下代码:
```
#include <QtWidgets>
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
// 生成随机数
int randomNumber = QRandomGenerator::global()->bounded(1, 100);
// 将随机数转为字符串
QString randomString = QString::number(randomNumber);
// 创建一个 QLabel 控件来显示随机数
QLabel label(randomString);
label.show();
return app.exec();
}
```
希望能对您有所帮助。如果还有其他问题,请随时提出。谢谢!
阅读全文
相关推荐
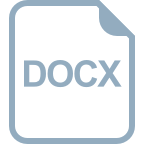
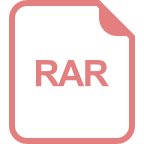
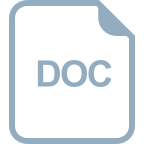
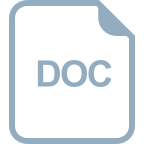









