linux编写一个c程序,满足以下要求: 用系统调用列出所有的信号;(kill –l) 用系统调用signal()让父进程捕捉键盘上来的中断信号(Ctrl-C); 当捕捉到中断信号后,父进程用系统调用kill()向两个子进程发出信号,子进程捕捉到信号后分别输出下列信息后终止 Child Process 1 is Killed by Parent! Child Process 2 is Killed by Parent! 父进程等待两个子进程终止后,输出如下的信息后终止: Parent Process is Killed!
时间: 2023-12-10 10:38:23 浏览: 35
以下是符合要求的程序代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <signal.h>
#include <unistd.h>
void child_handler(int sig);
int main() {
pid_t pid1, pid2;
// 列出所有信号
printf("All signals:\n");
system("kill -l");
// 捕捉 Ctrl-C 中断信号
signal(SIGINT, child_handler);
// 创建两个子进程
pid1 = fork();
if (pid1 < 0) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid1 == 0) {
// 子进程 1
printf("Child Process 1 is Running!\n");
while (1) {}
exit(EXIT_SUCCESS);
}
pid2 = fork();
if (pid2 < 0) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid2 == 0) {
// 子进程 2
printf("Child Process 2 is Running!\n");
while (1) {}
exit(EXIT_SUCCESS);
}
// 等待子进程终止
int status;
waitpid(pid1, &status, 0);
printf("Child Process 1 is Killed by Parent!\n");
waitpid(pid2, &status, 0);
printf("Child Process 2 is Killed by Parent!\n");
// 输出父进程终止信息
printf("Parent Process is Killed!\n");
return 0;
}
void child_handler(int sig) {
// 向两个子进程发送信号
kill(0, SIGKILL);
}
```
程序中,我们通过 `system("kill -l")` 调用系统命令 `kill -l` 来列出所有的信号,通过 `signal(SIGINT, child_handler)` 捕捉 Ctrl-C 中断信号,当捕捉到中断信号后,父进程调用 `kill(0, SIGKILL)` 向两个子进程发送 SIGKILL 信号,子进程捕捉到信号后输出相应信息并终止,父进程等待两个子进程终止后输出相应信息并终止。
相关推荐
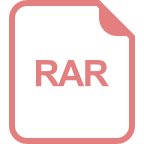
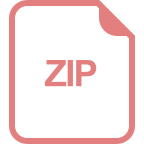
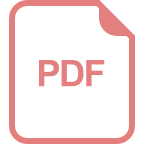















