["tid"]=2807,["uid"]=193712365,["count"]=5,["lv"]=90 这是一个字符串,写一个sql 把tid对应的2807值提取出来,可以使用正则表达式
时间: 2024-09-10 16:26:16 浏览: 76
这个字符串看起来像是JSON格式的数据,其中包含了"tid", "uid", "count", 和 "lv" 等键及其对应的值。如果你想通过SQL查询从这样的数据中提取"tid"为2807的信息,你需要先将字符串转换成实际的表结构或存储到数据库中。如果你有一个表格叫做`data`,并且它有一列`json_data`保存了这种格式的数据,你可以尝试使用一些函数来解析JSON(例如在PostgreSQL中使用`jsonb_extract_path_text()`)。
假设`json_data`是包含该信息的列,一个示例查询可能是这样的:
```sql
SELECT jsonb_extract_path_text(json_data, '$.tid') AS tid_value
FROM data
WHERE jsonb_extract_path_text(json_data, '$.tid')::integer = 2807;
```
这会返回所有tid值为2807的记录。如果直接处理字符串,你可能需要使用正则表达式(比如Python的`re`模块),但这通常不适合数据库操作,因为数据库有专门的工具处理JSON数据。
请注意,这个例子假设`$.tid`表示JSON路径,用于定位特定键。实际的路径可能会根据你的JSON结构有所不同。
相关问题
#include <unistd.h> #include <stdio.h> #include <pthread.h> void* daughter(void *num) { int *a=(int *) num; *a+=1; printf("I am daughter, count=%d\n",*a); } int main() { pthread_t son_tid, daughter_tid; int count=1; pthread_create(& son_tid,NULL, son,& count); pthread_create(& daughter_tid,NULL, daughter,& count); count++; printf("I am parent, count=%d\n", count); pthread_join(son_tid,NULL); pthread_join(daughter_tid,NULL); return 0; }将这个代码修改成正确的代码
这个代码存在几个问题:
1. `son` 函数未定义
2. `daughter` 函数中没有使用 `pthread_exit` 结束线程
3. 在主函数中创建线程后没有使用 `pthread_join` 等待线程结束
修改后的代码如下:
```c
#include <unistd.h>
#include <stdio.h>
#include <pthread.h>
void* son(void *num) {
int *a = (int *) num;
(*a)++;
printf("I am son, count=%d\n", *a);
pthread_exit(NULL);
}
void* daughter(void *num) {
int *a = (int *) num;
(*a)++;
printf("I am daughter, count=%d\n", *a);
pthread_exit(NULL);
}
int main() {
pthread_t son_tid, daughter_tid;
int count = 1;
pthread_create(&son_tid, NULL, son, &count);
pthread_create(&daughter_tid, NULL, daughter, &count);
count++;
printf("I am parent, count=%d\n", count);
pthread_join(son_tid, NULL);
pthread_join(daughter_tid, NULL);
return 0;
}
```
在修改后的代码中,我们定义了 `son` 函数并使用 `pthread_exit` 结束线程,同时在主函数中使用 `pthread_join` 等待线程结束。同样地,我们也定义了 `daughter` 函数并使用 `pthread_exit` 结束线程。最后,我们在主函数中创建线程并等待线程结束。
#include <unistd.h> #include <stdio.h> #include <pthread.h> void* son(void *num) { int *a = (int *) num; (*a)++; printf("I am son, count=%d\n", *a); pthread_exit(NULL); } void* daughter(void *num) { int *a = (int *) num; (*a)++; printf("I am daughter, count=%d\n", *a); pthread_exit(NULL); } int main() { pthread_t son_tid, daughter_tid; int count = 1; pthread_create(&son_tid, NULL, son, &count); pthread_create(&daughter_tid, NULL, daughter, &count); pthread_join(son_tid, NULL); pthread_join(daughter_tid, NULL); printf("I am parent, count=%d\n", count); return 0; }解释这段代码
这段代码是一个使用线程的程序,用于展示多个线程共享同一个变量的情况。在程序中,首先定义了两个线程 son 和 daughter,分别对应儿子和女儿的角色。同时定义了一个整型变量 count,初始值为 1。在 son 函数中,将传递进来的 num 指针转换为整型指针,并将其指向的变量值加 1,然后打印出 "I am son, count=2"。在 daughter 函数中,也是对传递进来的 num 指针指向的变量值加 1,然后打印出 "I am daughter, count=3"。在主函数中,先创建两个线程 son_tid 和 daughter_tid,分别执行 son 和 daughter 函数,并将 count 的地址传递给这两个线程。然后使用 pthread_join 函数等待这两个线程执行完毕。最后,打印出 "I am parent, count=3",表示主线程执行完毕。由于 son 和 daughter 两个线程共享了同一个变量 count,因此在对 count 进行加 1 的时候可能会出现竞争条件。但是由于这两个线程是由 pthread_create 函数创建的,因此它们在执行的时候会交替执行,从而避免了竞争条件的出现。因此,程序的输出结果应该是 "I am son, count=2\nI am daughter, count=3\nI am parent, count=3\n"。
阅读全文
相关推荐
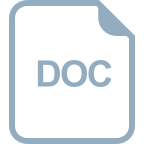
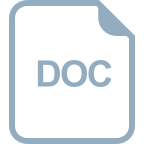
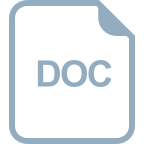









<c:set var="sum_price" value="0"></c:set> <c:set var="goods_count" value="0"></c:set> <c:set var="tids" value="#"></c:set> <c:forEach items="${trolleys }" var="trolley"> <c:set var="tids" value="${tids }#${trolley.tid }"></c:set>
${trolley.goods.gname } ${trolley.goods.color } ${trolley.goods.price }元 - <input type="text" value="${trolley.number }" id="num"> <c:set var="goods_count" value="${goods_count + trolley.number }"></c:set> + <c:set var="sum_price" value="${sum_price + trolley.goods.price * trolley.number }"></c:set> ${trolley.goods.price * trolley.number }元 删除 </c:forEach>

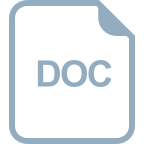
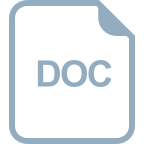
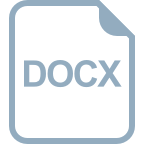