请给出Inception-V2的pytorch代码
时间: 2024-02-13 07:01:53 浏览: 24
以下是Inception-V2的PyTorch代码实现:
```python
import torch
import torch.nn as nn
class InceptionV2Block(nn.Module):
def __init__(self, in_channels, out_1x1, reduce_3x3, out_3x3, reduce_5x5, out_5x5, out_pool):
super(InceptionV2Block, self).__init__()
# 1x1 Convolution branch
self.branch1 = nn.Sequential(
nn.Conv2d(in_channels, out_1x1, kernel_size=1),
nn.BatchNorm2d(out_1x1),
nn.ReLU(inplace=True)
)
# 3x3 Convolution branch
self.branch2 = nn.Sequential(
nn.Conv2d(in_channels, reduce_3x3, kernel_size=1),
nn.BatchNorm2d(reduce_3x3),
nn.ReLU(inplace=True),
nn.Conv2d(reduce_3x3, out_3x3, kernel_size=3, padding=1),
nn.BatchNorm2d(out_3x3),
nn.ReLU(inplace=True)
)
# 5x5 Convolution branch
self.branch3 = nn.Sequential(
nn.Conv2d(in_channels, reduce_5x5, kernel_size=1),
nn.BatchNorm2d(reduce_5x5),
nn.ReLU(inplace=True),
nn.Conv2d(reduce_5x5, out_5x5, kernel_size=5, padding=2),
nn.BatchNorm2d(out_5x5),
nn.ReLU(inplace=True)
)
# Max Pooling branch
self.branch4 = nn.Sequential(
nn.MaxPool2d(kernel_size=3, stride=1, padding=1),
nn.Conv2d(in_channels, out_pool, kernel_size=1),
nn.BatchNorm2d(out_pool),
nn.ReLU(inplace=True)
)
def forward(self, x):
branch1_output = self.branch1(x)
branch2_output = self.branch2(x)
branch3_output = self.branch3(x)
branch4_output = self.branch4(x)
return torch.cat([branch1_output, branch2_output, branch3_output, branch4_output], 1)
class InceptionV2(nn.Module):
def __init__(self, num_classes=1000):
super(InceptionV2, self).__init__()
self.conv1 = nn.Sequential(
nn.Conv2d(3, 64, kernel_size=7, stride=2, padding=3),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1)
)
self.conv2 = nn.Sequential(
nn.Conv2d(64, 64, kernel_size=1),
nn.BatchNorm2d(64),
nn.ReLU(inplace=True),
nn.Conv2d(64, 192, kernel_size=3, padding=1),
nn.BatchNorm2d(192),
nn.ReLU(inplace=True),
nn.MaxPool2d(kernel_size=3, stride=2, padding=1)
)
self.inception3a = InceptionV2Block(192, 64, 96, 128, 16, 32, 32)
self.inception3b = InceptionV2Block(256, 128, 128, 192, 32, 96, 64)
self.inception4a = InceptionV2Block(480, 192, 96, 208, 16, 48, 64)
self.inception4b = InceptionV2Block(512, 160, 112, 224, 24, 64, 64)
self.inception4c = InceptionV2Block(512, 128, 128, 256, 24, 64, 64)
self.inception4d = InceptionV2Block(512, 112, 144, 288, 32, 64, 64)
self.inception4e = InceptionV2Block(528, 256, 160, 320, 32, 128, 128)
self.inception5a = InceptionV2Block(832, 256, 160, 320, 32, 128, 128)
self.inception5b = InceptionV2Block(832, 384, 192, 384, 48, 128, 128)
self.avg_pool = nn.AdaptiveAvgPool2d((1, 1))
self.dropout = nn.Dropout(0.4)
self.fc = nn.Linear(1024, num_classes)
def forward(self, x):
x = self.conv1(x)
x = self.conv2(x)
x = self.inception3a(x)
x = self.inception3b(x)
x = self.inception4a(x)
x = self.inception4b(x)
x = self.inception4c(x)
x = self.inception4d(x)
x = self.inception4e(x)
x = self.inception5a(x)
x = self.inception5b(x)
x = self.avg_pool(x)
x = x.view(x.size(0), -1)
x = self.dropout(x)
x = self.fc(x)
return x
```
相关推荐
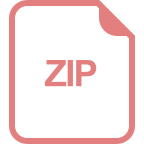
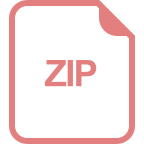














