请用java api写一个hbase的增删改查
时间: 2024-03-09 19:51:08 浏览: 65
好的,以下是Java API实现HBase的增删改查操作的示例代码:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.TableName;
import org.apache.hadoop.hbase.client.*;
public class HBaseExample {
public static void main(String[] args) throws Exception {
// 创建HBase配置对象
Configuration config = HBaseConfiguration.create();
// 设置Zookeeper地址
config.set("hbase.zookeeper.quorum", "localhost");
// 获取HBase连接
try (Connection connection = ConnectionFactory.createConnection(config)) {
// 获取表对象
Table table = connection.getTable(TableName.valueOf("test_table"));
// 插入数据
Put put = new Put("rowkey".getBytes());
put.addColumn("cf".getBytes(), "col1".getBytes(), "value1".getBytes());
put.addColumn("cf".getBytes(), "col2".getBytes(), "value2".getBytes());
table.put(put);
// 查询数据
Get get = new Get("rowkey".getBytes());
Result result = table.get(get);
byte[] value = result.getValue("cf".getBytes(), "col1".getBytes());
System.out.println(new String(value));
// 修改数据
Put update = new Put("rowkey".getBytes());
update.addColumn("cf".getBytes(), "col1".getBytes(), "new_value".getBytes());
table.put(update);
// 删除数据
Delete delete = new Delete("rowkey".getBytes());
delete.addColumn("cf".getBytes(), "col1".getBytes());
table.delete(delete);
// 关闭表对象
table.close();
}
}
}
```
注意替换代码中的`localhost`为你的Zookeeper地址,并替换`test_table`为你的表名。同时,需要添加HBase客户端依赖包到你的项目中。
阅读全文
相关推荐
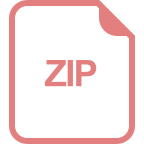
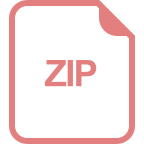
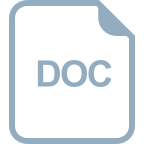














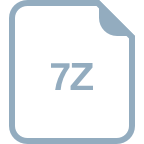