编写一个函数,返回储存在double类型数组中最大值的下标,编写一个函数将数组原有数据倒序排列,并在一个简单的程序中测试两个函数。
时间: 2023-04-14 19:01:15 浏览: 127
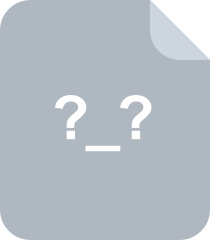
设计一个函数, 找到数组中的最大值以及下标。
下面是函数的代码:
```c++
#include <iostream>
using namespace std;
int findMaxIndex(double arr[], int size) {
int maxIndex = ;
for (int i = 1; i < size; i++) {
if (arr[i] > arr[maxIndex]) {
maxIndex = i;
}
}
return maxIndex;
}
void reverseArray(double arr[], int size) {
for (int i = ; i < size / 2; i++) {
double temp = arr[i];
arr[i] = arr[size - i - 1];
arr[size - i - 1] = temp;
}
}
int main() {
double arr[] = { 1.2, 3.4, 5.6, 7.8, 9. };
int size = sizeof(arr) / sizeof(double);
int maxIndex = findMaxIndex(arr, size);
cout << "Max value is " << arr[maxIndex] << " at index " << maxIndex << endl;
reverseArray(arr, size);
cout << "Reversed array: ";
for (int i = ; i < size; i++) {
cout << arr[i] << " ";
}
cout << endl;
return ;
}
```
这个程序首先定义了两个函数,一个是 `findMaxIndex`,用于找到数组中最大值的下标;另一个是 `reverseArray`,用于将数组原有数据倒序排列。
在 `main` 函数中,我们定义了一个包含一些随机数的数组,并计算出数组的大小。然后我们调用 `findMaxIndex` 函数来找到最大值的下标,并输出结果。接着,我们调用 `reverseArray` 函数来将数组倒序排列,并输出结果。
这个程序的输出应该是这样的:
```
Max value is 9 at index 4
Reversed array: 9 7.8 5.6 3.4 1.2
```
这表明我们的两个函数都能正常工作。
阅读全文
相关推荐
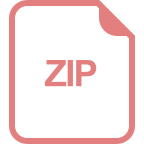











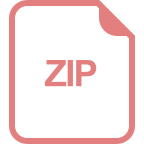



