用python做一个坦克大战游戏
时间: 2024-05-18 20:18:48 浏览: 30
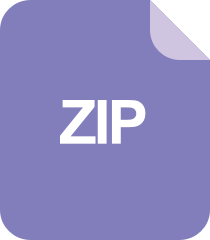
基于Python实现坦克大战游戏【100012786】
好的,下面是一个简单的坦克大战游戏的Python代码:
```python
import pygame
import random
# 初始化游戏
pygame.init()
# 定义游戏屏幕大小
screen_width = 640
screen_height = 480
# 创建游戏屏幕
screen = pygame.display.set_mode((screen_width, screen_height))
# 加载坦克和子弹图片
tank_img = pygame.image.load('tank.png')
bullet_img = pygame.image.load('bullet.png')
# 定义坦克类
class Tank:
def __init__(self, x, y):
self.x = x
self.y = y
self.direction = 'up'
self.speed = 3
self.bullets = []
# 移动坦克
def move(self):
if self.direction == 'up':
if self.y > 0:
self.y -= self.speed
elif self.direction == 'down':
if self.y < screen_height - 50:
self.y += self.speed
elif self.direction == 'left':
if self.x > 0:
self.x -= self.speed
elif self.direction == 'right':
if self.x < screen_width - 50:
self.x += self.speed
# 发射子弹
def fire(self):
bullet = Bullet(self.x + 20, self.y + 20, self.direction)
self.bullets.append(bullet)
# 绘制坦克和子弹
def draw(self):
screen.blit(tank_img, (self.x, self.y))
for bullet in self.bullets:
bullet.move()
bullet.draw()
# 定义子弹类
class Bullet:
def __init__(self, x, y, direction):
self.x = x
self.y = y
self.direction = direction
self.speed = 5
# 移动子弹
def move(self):
if self.direction == 'up':
if self.y > 0:
self.y -= self.speed
else:
self.kill()
elif self.direction == 'down':
if self.y < screen_height:
self.y += self.speed
else:
self.kill()
elif self.direction == 'left':
if self.x > 0:
self.x -= self.speed
else:
self.kill()
elif self.direction == 'right':
if self.x < screen_width:
self.x += self.speed
else:
self.kill()
# 绘制子弹
def draw(self):
screen.blit(bullet_img, (self.x, self.y))
# 移除子弹
def kill(self):
tank.bullets.remove(self)
# 创建坦克对象
tank = Tank(300, 400)
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
tank.direction = 'up'
elif event.key == pygame.K_DOWN:
tank.direction = 'down'
elif event.key == pygame.K_LEFT:
tank.direction = 'left'
elif event.key == pygame.K_RIGHT:
tank.direction = 'right'
elif event.key == pygame.K_SPACE:
tank.fire()
# 移动坦克和子弹
tank.move()
# 绘制背景
screen.fill((255, 255, 255))
# 绘制坦克和子弹
tank.draw()
# 更新屏幕
pygame.display.update()
```
这个游戏还比较简单,你可以根据自己的需求对代码进行修改和扩展。
阅读全文
相关推荐
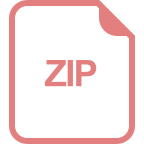










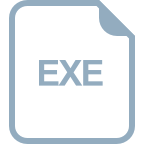
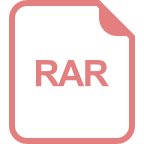
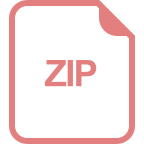
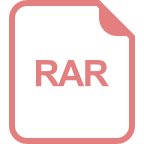