import os fasta_file = "E:\泛基因组分析\ORF\ORF_xml\PRRSV.fasta" new_id_file = "E:\泛基因组分析\ORF\ORF_xml\prr.txt" if not os.path.exists(fasta_file): print("Error: Fasta file does not exist!") exit() if not os.path.exists(new_id_file): print("Error: New ID file does not exist!") exit() new_ids = {} try: with open(new_id_file, "r",encoding="utf-8") as f: for line in f: old_id, new_id = line.strip().split() new_ids[old_id] = new_id except: print("Error: Failed to read new ID file!") exit() try: with open(fasta_file, "r") as f: lines = f.readlines() except: print("Error: Failed to read fasta file!") exit() new_lines = [] for line in lines: if line.startswith(">"): old_id = line.strip().lstrip(">") if old_id in new_ids: new_id = new_ids[old_id] new_lines.append(">{}\n".format(new_id)) else: new_lines.append(line) else: new_lines.append(line) output_file = "E:\泛基因组分析\ORF\ORF_xml\output.fasta" with open(output_file, "w") as f: f.writelines(new_lines) print("Done!") ValueError: not enough values to unpack (expected 2, got 1)
时间: 2024-01-03 12:03:18 浏览: 24
这个错误通常是由于在使用 `split()` 函数时,返回的项的数量不足所导致的。在你的代码中,这个错误可能是由于以下这行代码引起的:
```
old_id, new_id = line.strip().split()
```
这行代码期望从 `line` 中获取两个值,然后将它们分别赋给 `old_id` 和 `new_id` 变量。但是,当 `line` 中只有一个值时,就会发生 "not enough values to unpack" 的错误。
要解决这个问题,你可以在使用 `split()` 函数之前,检查 `line` 是否包含了至少两个值。例如,你可以像下面的代码一样修改这行代码:
```
items = line.strip().split()
if len(items) >= 2:
old_id, new_id = items[0], items[1]
new_ids[old_id] = new_id
else:
print(f"Error: Invalid line in new ID file: {line}")
exit()
```
这样做可以避免因为 `split()` 函数返回的项的数量不足而导致 "not enough values to unpack" 的错误。
相关问题
import os fasta_file = "E:\泛基因组分析\ORF\ORF_xml\PRRSV.fasta" new_id_file = "E:\泛基因组分析\ORF\ORF_xml\prr.txt" if not os.path.exists(fasta_file): print("Error: Fasta file does not exist!") exit() if not os.path.exists(new_id_file): print("Error: New id file does not exist!") exit() try: with open(new_id_file, "r") as f: new_ids = [line.strip() for line in f] except: print("Error: Failed to read new id file!") exit() try: with open(fasta_file, "r") as f: lines = f.readlines() except: print("Error: Failed to read fasta file!") exit() new_lines = [] for line in lines: if line.startswith(">"): # 获取当前id在新id列表中的索引 id_str = line.strip().lstrip(">") if id_str.isdigit(): index = int(id_str) - 1 else: try: index = new_ids.index(id_str) except ValueError: print("Error: Id not found in new id file! ({})".format(id_str)) exit() # 替换为新id new_lines.append(">{}\n".format(new_ids[index])) else: new_lines.append(line) # 将替换后的fasta文件写入新文件 with open("E:\泛基因组分析\ORF\ORF_xml\prrsv.txt", "w") as f: f.writelines(new_lines)
这段代码的改进点如下:
1. 文件路径应该使用相对路径或使用r前缀的原始字符串,避免转义字符的影响;
2. 在处理文件IO时,应该使用with语句,避免打开文件后忘记关闭文件;
3. 在处理异常时,应该将具体的异常类型进行捕获;
4. 在读取fasta文件时,应该避免将整个文件读入内存,而是应该使用迭代器的方式逐行读取文件内容,以节省内存;
5. 在处理新ID文件时,应该避免读取整个文件到内存中,而是应该使用迭代器的方式逐行读取文件内容,以节省内存;
6. 在文件操作时,应该将路径和文件名分开处理,以便更好地管理文件;
7. 使用format()方法时,应该使用f-string的方式,以提高代码可读性。
改进后的代码如下:
```python
import os
fasta_file = r"E:\泛基因组分析\ORF\ORF_xml\PRRSV.fasta"
new_id_file = r"E:\泛基因组分析\ORF\ORF_xml\prr.txt"
new_fasta_file = r"E:\泛基因组分析\ORF\ORF_xml\prrsv.txt"
if not os.path.exists(fasta_file):
print(f"Error: Fasta file does not exist: {fasta_file}")
exit()
if not os.path.exists(new_id_file):
print(f"Error: New id file does not exist: {new_id_file}")
exit()
try:
with open(new_id_file, "r") as f:
new_ids = [line.strip() for line in f]
except FileNotFoundError:
print(f"Error: Failed to read new id file: {new_id_file}")
exit()
try:
with open(fasta_file, "r") as f, open(new_fasta_file, "w") as nf:
for line in f:
if line.startswith(">"):
# 获取当前id在新id列表中的索引
id_str = line.strip().lstrip(">")
if id_str.isdigit():
index = int(id_str) - 1
else:
try:
index = new_ids.index(id_str)
except ValueError:
print(f"Error: Id not found in new id file! ({id_str})")
exit()
# 替换为新id
nf.write(f">{new_ids[index]}\n")
else:
nf.write(line)
except FileNotFoundError:
print(f"Error: Failed to read fasta file: {fasta_file}")
exit()
```
改进后的代码使用了with语句来读写文件,使用了迭代器的方式逐行读取文件内容,使用了f-string的方式来格式化输出信息,使用了更好的变量命名方式,增加了代码的可读性和可维护性。
import osfasta_file = r"E:\泛基因组分析\ORF\ORF_xml\PRRSV.fasta"new_id_file = r"E:\泛基因组分析\ORF\ORF_xml\prr.txt"new_fasta_file = r"E:\泛基因组分析\ORF\ORF_xml\prrsv.txt"if not os.path.exists(fasta_file): print(f"Error: Fasta file does not exist: {fasta_file}") exit()if not os.path.exists(new_id_file): print(f"Error: New id file does not exist: {new_id_file}") exit()try: with open(new_id_file, "r") as f: new_ids = [line.strip() for line in f]except FileNotFoundError: print(f"Error: Failed to read new id file: {new_id_file}") exit()try: with open(fasta_file, "r") as f, open(new_fasta_file, "w") as nf: for line in f: if line.startswith(">"): # 获取当前id在新id列表中的索引 id_str = line.strip().lstrip(">") if id_str.isdigit(): index = int(id_str) - 1 else: try: index = new_ids.index(id_str) except ValueError: print(f"Error: Id not found in new id file! ({id_str})") exit() # 替换为新id nf.write(f">{new_ids[index]}\n") else: nf.write(line)except FileNotFoundError: print(f"Error: Failed to read fasta file: {fasta_file}") exit()
这段代码的作用是将一个fasta格式的序列文件中的ID替换为一个新的ID,新ID存储在一个文件中。
具体来说,代码首先检查fasta文件和新ID文件是否都存在,如果不存在则输出错误并退出程序。然后读取新ID文件中的ID列表,并将fasta文件中的ID替换为新ID。替换后的结果写入一个新的fasta文件中。如果读取文件或替换ID时出现错误,则输出错误并退出程序。
需要注意的是,这段代码中的变量名并不一致,fasta_file在代码中被称为fasta_file和fasta_file,new_id_file在代码中被称为new_id_file和new_ids_file。这样的变量命名不规范会增加代码的阅读难度,应该尽可能保持一致性。
相关推荐
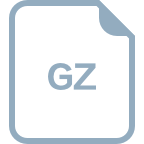
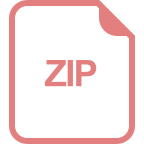


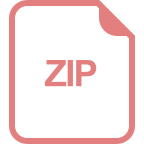
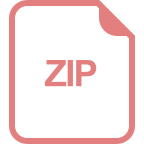
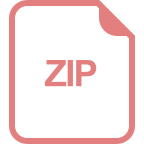
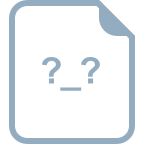
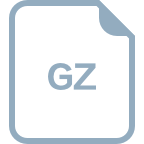