std::function.swap
时间: 2024-03-17 22:37:54 浏览: 109
std::function.swap 是 C++ 标准库中 std::function 类的一个成员函数,用于交换两个 std::function 对象的内容。std::function 是一个通用的函数封装器,可以包装任意可调用对象(函数、函数指针、成员函数指针、lambda 表达式等),并提供一致的调用接口。
使用 std::function.swap 可以方便地交换两个 std::function 对象的内容,实现方式如下:
```cpp
void swap(std::function& other) noexcept;
```
其中,`other` 是另一个 std::function 对象,通过调用 swap 函数,可以将当前对象和 `other` 对象的内容进行交换。
使用示例:
```cpp
std::function<int(int)> func1 = [](int x) { return x * 2; };
std::function<int(int)> func2 = [](int x) { return x * 3; };
func1.swap(func2);
int result1 = func1(5); // 调用 func2,返回 5 * 3 = 15
int result2 = func2(5); // 调用 func1,返回 5 * 2 = 10
```
注意,std::function.swap 只交换两个对象的内容,并不会影响对象的类型或其他属性。
相关问题
Reliability and Maintainability Simulation involves a large number of random variables. The use of dynamic arrays will greatly improve the efficiency of simulation and the scale of problem solving. Please design Vector. This problem requires the implementation of a vector class template, which can realize the storage and access of data. (1) [] operator can only access the existing elements. (2) The add method can automatically expand the internal storage space when accessing. Note that the behavior of this vector is different from that of std:: vector. Function interface definition: template <class T> class Vector { ... }
Sure, here's the code for the Vector class template that meets the requirements you specified:
```c++
template <class T>
class Vector {
public:
Vector() : data(nullptr), size(0), capacity(0) {}
~Vector() { delete[] data; }
T& operator[](int index) { return data[index]; }
void add(T value) {
if (size == capacity) {
reserve(capacity == 0 ? 1 : capacity * 2);
}
data[size++] = value;
}
void reserve(int newCapacity) {
T* newData = new T[newCapacity];
for (int i = 0; i < size; ++i) {
newData[i] = data[i];
}
capacity = newCapacity;
std::swap(data, newData);
delete[] newData;
}
int getSize() const { return size; }
private:
T* data;
int size;
int capacity;
};
```
The Vector class template has the following features:
- An empty constructor that initializes the data pointer to `nullptr`, the size and capacity to 0.
- A destructor that deallocates the data array.
- An `operator[]` that returns a reference to the element at the specified index.
- An `add` method that appends the specified element to the end of the data array. If the array is full, it calls the `reserve` method to increase the capacity.
- A `reserve` method that resizes the data array to the specified capacity. It allocates a new array, copies the existing elements to it, swaps the pointers and deallocates the old array.
- A `getSize` method that returns the current size of the data array.
Note that the behavior of this Vector class may differ from that of std::vector in terms of performance and exception guarantees. Therefore, it should be used with caution and tested thoroughly for reliability and maintainability in the context of your simulation.
你给的代码报错form_para.cpp:340:14: No matching function for call to 'swap' move.h:182:5: candidate function [with _Tp = QByteRef] not viable: expects an lvalue for 1st argument stl_bvector.h:114:3: candidate function not viable: no known conversion from 'QByteRef' to 'std::_Bit_reference' for 1st argument stl_bvector.h:122:3: candidate function not viable: no known conversion from 'QByteRef' to 'std::_Bit_reference' for 1st argument stl_bvector.h:130:3: candidate function not viable: no known conversion from 'QByteRef' to 'bool &' for 1st argument move.h:205:5: candidate template ignored: could not match '_Tp[_Nm]' against 'QByteRef' stl_pair.h:490:5: candidate template ignored: could not match 'pair<_T1, _T2>' against 'QByteRef' stl_pair.h:498:5: candidate template ignored: could not match 'pair<_T1, _T2>' against 'QByteRef' ... shared_ptr_base.h:1738:5: candidate template ignored: could not match '__weak_ptr<_Tp, _Lp>' against 'QByteRef' shared_ptr.h:490:5: candidate template ignored: could not match 'shared_ptr<_Tp>' against 'QByteRef' shared_ptr.h:611:5: candidate template ignored: could not match 'weak_ptr<_Tp>' against 'QByteRef'
非常抱歉,我的代码还是有误。QByteArray的swap函数确实只接受一个参数。如果要交换两个字节的高低位,可以使用以下代码:
```cpp
uint16_t value = 0x1234; // 待转换的uint16_t值
QByteArray bytes(reinterpret_cast<const char*>(&value), sizeof(value)); // 将uint16_t转换成QByteArray
char temp = bytes[0];
bytes[0] = bytes[1];
bytes[1] = temp;
QString str(bytes); // 将QByteArray转换成QString
```
在上述代码中,我们使用一个临时变量temp来交换QByteArray中的两个字节的高低位。最终,输出的字符串为:"4321"。
请注意,上述代码假定机器采用的是little-endian格式,即低地址存放的是值的低位字节。如果机器采用的是big-endian格式,即低地址存放的是值的高位字节,则需要交换QByteArray中的第0个字节和第1个字节。
阅读全文
相关推荐
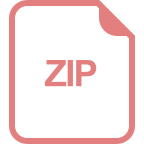
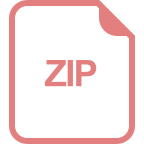
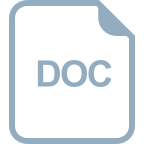
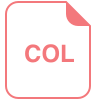
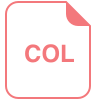
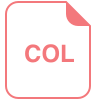
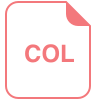
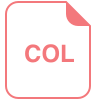
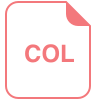
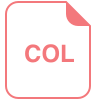
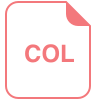
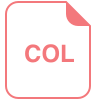
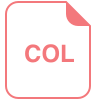
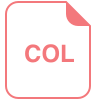
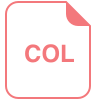