c语言推箱子游戏代码
时间: 2023-10-23 09:02:47 浏览: 146
推箱子是一种经典的益智游戏,下面我将用300字来简要介绍一下C语言编写的推箱子游戏的代码实现。
推箱子游戏需要一个二维数组来表示游戏地图,玩家可控制的角色在地图上移动,目标是将所有的箱子推到指定位置。以下是代码主要的实现步骤:
1. 定义二维数组作为游戏地图,使用数字来表示各个元素。例如,0代表空地,1代表墙壁,2代表箱子,3代表目标位置,4代表角色。
2. 初始化地图,并通过循环遍历将地图元素打印到屏幕上。
3. 实现玩家角色的移动功能。可以使用键盘监听函数获取玩家输入的方向,并根据玩家输入更新角色所在位置。
4. 实现箱子的推动功能。当角色与箱子相邻时,判断箱子后面的位置是否为空地或目标位置,如果是,则更新地图,将箱子推到新位置,否则不移动箱子。
5. 在每次角色移动后,判断是否完成游戏目标。遍历地图上的目标位置,如果有一个位置上的箱子缺失,则游戏继续,否则游戏胜利。
6. 当游戏胜利时,显示相应的提示信息并退出游戏。
通过以上步骤,我们可以完成一个简单的推箱子游戏的C语言代码实现。当然,还可以添加其他功能,如关卡选择、计时等,以增加游戏的趣味性和挑战性。
相关问题
c语言推箱子完整代码
当然,下面是一个简单的 C 语言推箱子游戏的完整代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#define MAX_ROW 10
#define MAX_COL 10
// 定义地图元素类型
typedef enum {
EMPTY,
WALL,
BOX,
TARGET,
PLAYER
} element;
// 定义地图结构体
typedef struct {
int row;
int col;
} position;
// 初始化地图
void initializeMap(element map[MAX_ROW][MAX_COL], position *player, position targets[], int *numTargets) {
element initialMap[MAX_ROW][MAX_COL] = {
{WALL, WALL, WALL, WALL, WALL, WALL, WALL, WALL, WALL, WALL},
{WALL, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, WALL},
{WALL, EMPTY, BOX, BOX, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, WALL},
{WALL, EMPTY, BOX, TARGET, EMPTY, EMPTY, EMPTY, EMPTY, EMPTY, WALL},
{WALL, PLAYER, EMPTY, EMPTY, EMPTY, TARGET, EMPTY, BOX, EMPTY, WALL},
{WALL, EMPTY, BOX, BOX, EMPTY, EMPTY, TARGET, TARGET, TARGET, WALL},
{WALL, EMPTY, BOX, EMPTY, TARGET, EMPTY, TARGET, PLAYER, EMPTY, WALL},
{WALL, EMPTY, BOX, EMPTY, TARGET, BOX, TARGET, TARGET, EMPTY, WALL},
{WALL, EMPTY, BOX, TARGET, TARGET, TARGET, TARGET, TARGET ,EMPTY ,WALL},
{WALL ,WALL ,WALL ,WALL ,WALL ,WALL ,WALL ,WALL ,WALL ,WALL}
};
for (int i = 0; i < MAX_ROW; i++) {
for (int j = 0; j < MAX_COL; j++) {
map[i][j] = initialMap[i][j];
if (map[i][j] == PLAYER) {
player->row = i;
player->col = j;
}
if (map[i][j] == TARGET) {
targets[*numTargets].row = i;
targets[*numTargets].col = j;
(*numTargets)++;
}
}
}
}
// 渲染地图
void renderMap(element map[MAX_ROW][MAX_COL]) {
for (int i = 0; i < MAX_ROW; i++) {
for (int j = 0; j < MAX_COL; j++) {
switch(map[i][j]) {
case WALL:
printf("#");
break;
case EMPTY:
printf(" ");
break;
case BOX:
printf("$");
break;
case TARGET:
printf(".");
break;
case PLAYER:
printf("@");
break;
}
}
printf("\n");
}
}
// 移动玩家
void movePlayer(element map[MAX_ROW][MAX_COL], position *player, int row, int col) {
int newRow = player->row + row;
int newCol = player->col + col;
if (map[newRow][newCol] == EMPTY || map[newRow][newCol] == TARGET) {
map[player->row][player->col] = (map[player->row][player->col] == TARGET) ? TARGET : EMPTY;
player->row = newRow;
player->col = newCol;
map[player->row][player->col] = PLAYER;
}
else if (map[newRow][newCol] == BOX) {
int newBoxRow = newRow + row;
int newBoxCol = newCol + col;
if (map[newBoxRow][newBoxCol] == EMPTY || map[newBoxRow][newBoxCol] == TARGET) {
map[player->row][player->col] = (map[player->row][player->col] == TARGET) ? TARGET : EMPTY;
player->row = newRow;
player->col = newCol;
map[player->row][player->col] = PLAYER;
map[newBoxRow][newBoxCol] = (map[newBoxRow][newBoxCol] == TARGET) ? BOX : TARGET;
}
}
}
int main() {
element map[MAX_ROW][MAX_COL];
position player;
position targets[MAX_ROW * MAX_COL];
int numTargets = 0;
initializeMap(map, &player, targets, &numTargets);
while (1) {
system("cls");
renderMap(map);
if (numTargets == 0) {
printf("You win!\n");
break;
}
char input = getch();
switch(input) {
case 'w':
movePlayer(map, &player, -1, 0);
break;
case 's':
movePlayer(map, &player, 1, 0);
break;
case 'a':
movePlayer(map, &player, 0, -1);
break;
case 'd':
movePlayer(map, &player, 0, 1);
break;
case 'q':
printf("Goodbye!\n");
exit(0);
}
}
return 0;
}
```
这段代码实现了一个简单的推箱子游戏。你可以使用WASD键来控制玩家的移动,目标是将所有的箱子推到目标位置上。运行代码后,地图会显示在控制台上,直至完成游戏或按下Q键退出。
c语言推箱子代码
推箱子是一种经典的游戏,C语言实现推箱子游戏的代码如下:
```c
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <windows.h>
#define ROW 10 // 地图行数
#define COL 10 // 地图列数
int map[ROW][COL] = { // 地图数组,0表示空地,1表示墙,2表示箱子,3表示目标点,4表示人
{1,1,1,1,1,1,1,1,1,1},
{1,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,3,0,1},
{1,0,0,0,0,0,0,0,2,1},
{1,0,0,0,0,0,0,0,0,1},
{1,3,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,1},
{1,0,0,0,0,0,0,0,0,1},
{1,1,1,1,1,1,1,1,1,1}
};
int px, py; // 人的位置
void drawMap() { // 绘制地图
system("cls");
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
switch (map[i][j]) {
case 0: // 空地
printf(" ");
break;
case 1: // 墙
printf("□");
break;
case 2: // 箱子
printf("■");
break;
case 3: // 目标点
printf("☆");
break;
case 4: // 人
printf("♀");
px = i;
py = j;
break;
default:
break;
}
}
printf("\n");
}
}
int main() {
drawMap();
getch();
while (1) {
int key = getch();
int mx = px, my = py;
switch (key) {
case 72: // 上
mx--;
break;
case 80: // 下
mx++;
break;
case 75: // 左
my--;
break;
case 77: // 右
my++;
break;
default:
break;
}
if (map[mx][my] == 1) { // 撞到墙,不动
continue;
}
if (map[mx][my] == 2) { // 推箱子
int nx = mx - px + mx, ny = my - py + my;
if (map[nx][ny] == 1 || map[nx][ny] == 2) { // 箱子撞到墙或者箱子,不动
continue;
}
map[nx][ny] = 2;
map[mx][my] = 4;
map[px][py] = 0;
px = mx;
py = my;
}
else { // 普通移动
map[mx][my] = 4;
map[px][py] = 0;
px = mx;
py = my;
}
drawMap();
// 判断是否完成
int flag = 1;
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
if (map[i][j] == 3 || map[i][j] == 2) {
flag = 0;
break;
}
}
}
if (flag) {
printf("Congratulations, you have completed the game!\n");
break;
}
}
getch();
return 0;
}
```
运行程序后,使用方向键控制人物移动,推动箱子到目标点即可完成游戏。
相关推荐
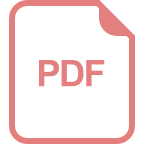
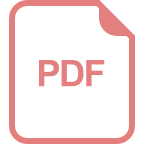












