3des加密算法c++实现
时间: 2024-10-08 21:27:07 浏览: 37
三重DES (Triple DES) 是DES算法的一个增强版本,它使用三个独立的DES密钥对数据进行三次加密。在C++中,你可以通过Boost库或者自行编写一些底层代码来实现。以下是一个简化的三重DES加密步骤的概述:
1. 导入必要的库:如果使用标准C++,你需要自定义函数;若使用Boost库,则`boost::cryptopp::(des)` 类将很有帮助。
2. 定义三个密钥(Key1、Key2 和 Key3)。
3. 创建一个DES加密器实例,每次使用其中一个密钥。
4. 对明文进行第一次加密(使用Key1)。
5. 将第一步的结果作为新的明文,再次加密(使用Key2)。
6. 最后一次加密结果,使用第三个密钥Key3。
7. 得到最终的三重DES加密后的密文。
以下是一个基础的C++代码示例(假设使用了Boost库):
```cpp
#include <boost/cryptopp/des.hpp>
#include <string>
std::string triple_des_encrypt(const std::string& plaintext, const std::string& key1, const std::string& key2, const std::string& key3) {
boost::cipher::des des(key1.begin(), boost::cipher::ecb);
std::string encrypted1 = des.encrypt(plaintext.c_str());
boost::cipher::des des2(key2.begin(), boost::cipher::ecb);
std::string intermediate = des2.encrypt(encrypted1.c_str());
boost::cipher::des des3(key3.begin(), boost::cipher::ecb);
return des3.encrypt(intermediate.c_str());
}
```
请注意,这只是一个简化版的实现,并未涉及错误处理和其他安全性考虑。实际应用中,你还需要妥善管理密钥和进行适当的输入验证。
相关推荐
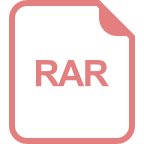
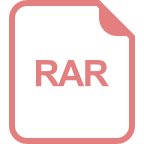
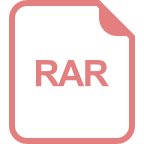
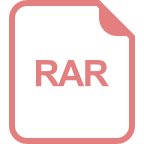
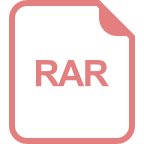
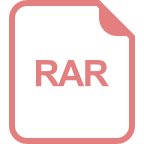
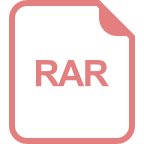
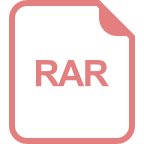
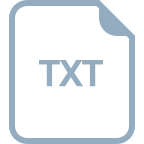



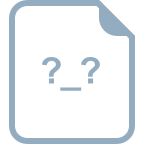
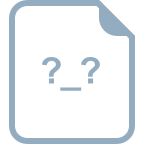