3des加密算法c++
时间: 2024-10-17 21:03:28 浏览: 46
三重DES (Triple DES) 是一种广泛使用的数据加密标准,它实际上是DES算法三次应用的过程,通过两个独立的DES密钥对数据进行两次加密,然后再用第三个密钥解密。在C++中,你可以使用OpenSSL库来进行3DES操作,因为其包含了对这种加密技术的支持。
以下是一个简单的示例,展示如何使用OpenSSL的`cryptopp`库进行3DES加密和解密:
```cpp
#include <openssl/des.h>
#include <string>
std::string encrypt_des(const std::string& plaintext, const std::string& key1, const std::string& key2) {
CryptoPP::DES::Encryption des1(key1, CryptoPP::DEFAULT_KEYLENGTH);
CryptoPP::DES::Encryption des2(key2, CryptoPP::DEFAULT_KEYLENGTH);
// 首先用key1加密
std::string encrypted = des1.ProcessBlock(reinterpret_cast<const unsigned char*>(plaintext.c_str()), true);
// 然后再用key2加密结果
return des2.ProcessBlock(reinterpret_cast<const unsigned char*>(encrypted.c_str()), false);
}
std::string decrypt_des(const std::string& ciphertext, const std::string& key1, const std::string& key2) {
CryptoPP::DES::Decryption des1(key1, CryptoPP::DEFAULT_KEYLENGTH);
CryptoPP::DES::Decryption des2(key2, CryptoPP::DEFAULT_KEYLENGTH);
// 先用key2解密
std::string decrypted = des2.ProcessBlock(reinterpret_cast<const unsigned char*>(ciphertext.c_str()), true);
// 再次使用key1解密原始数据
return des1.ProcessBlock(reinterpret_cast<const unsigned char*>(decrypted.c_str()), false);
}
```
注意,实际应用中你需要处理密钥管理和填充/前缀等问题。同时,OpenSSL库需要链接到你的项目中才能使用。
阅读全文
相关推荐
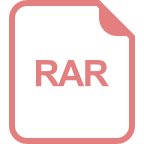
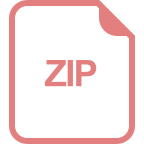
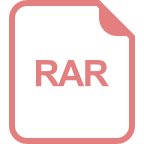
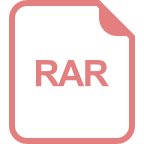

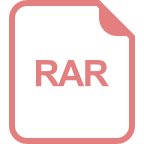
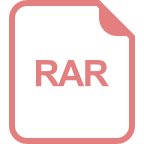

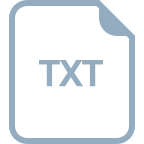
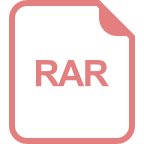
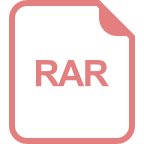

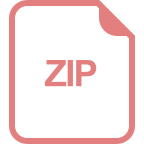
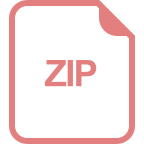