通过按钮和文本框用pyqt5实现在tableWidget中数据的查找和筛选
时间: 2024-02-09 07:11:31 浏览: 22
好的,你可以通过以下的方法实现在tableWidget中数据的查找和筛选:
1. 创建一个 pyqt5 的界面,并添加一个 tableWidget 控件。
2. 在界面中添加一个 QLineEdit 控件和一个 QPushButton 控件,用于输入查找关键字和触发查找功能。
3. 创建一个函数,用于实现查找功能。在该函数中,获取 QLineEdit 控件中的文本,遍历 tableWidget 中的每个单元格,如果该单元格的文本包含查找关键字,则将该单元格所在的行显示出来,否则将该行隐藏。
4. 将该函数与 QPushButton 控件的 clicked 信号绑定,实现点击按钮即可触发查找功能。
下面是示例代码:
```
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QHBoxLayout, QVBoxLayout, QTextEdit, QPushButton, QLineEdit, QTableWidgetItem, QTableWidget
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# 创建界面布局
main_widget = QWidget()
main_layout = QVBoxLayout()
button_layout = QHBoxLayout()
# 添加 QLineEdit 控件和 QPushButton 控件
self.search_box = QLineEdit()
self.search_box.setPlaceholderText('输入关键字查找')
self.search_button = QPushButton('查找')
# 添加 tableWidget 控件
self.table_widget = QTableWidget()
header_labels = ['姓名', '年龄', '性别']
self.table_widget.setColumnCount(len(header_labels))
self.table_widget.setHorizontalHeaderLabels(header_labels)
data = [['张三', '20', '男'], ['李四', '25', '女'], ['王五', '22', '男']]
self.table_widget.setRowCount(len(data))
for i, row in enumerate(data):
for j, cell in enumerate(row):
item = QTableWidgetItem(cell)
self.table_widget.setItem(i, j, item)
# 将 QLineEdit 控件和 QPushButton 控件添加到按钮布局中
button_layout.addWidget(self.search_box)
button_layout.addWidget(self.search_button)
# 将按钮布局和 tableWidget 控件添加到主布局中
main_layout.addLayout(button_layout)
main_layout.addWidget(self.table_widget)
main_widget.setLayout(main_layout)
self.setCentralWidget(main_widget)
# 绑定按钮点击事件
self.search_button.clicked.connect(self.search)
# 查找函数
def search(self):
keyword = self.search_box.text()
for i in range(self.table_widget.rowCount()):
matches = False
for j in range(self.table_widget.columnCount()):
cell_text = self.table_widget.item(i, j).text()
if keyword in cell_text:
matches = True
break
if matches:
self.table_widget.showRow(i)
else:
self.table_widget.hideRow(i)
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在上述代码中,我们创建了一个带有 tableWidget 控件的界面,并添加了一个 QLineEdit 控件和一个 QPushButton 控件。当用户点击按钮时,程序会从 QLineEdit 控件中获取关键字,然后遍历 tableWidget 中的每个单元格,判断该单元格的文本是否包含关键字,如果包含,则显示该单元格所在的行。最后,将查找到的结果显示出来。
希望对你有帮助!
相关推荐
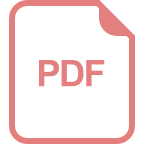
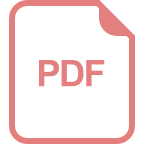
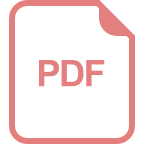














