unity代码调整文字颜色透明度
时间: 2023-07-09 15:40:35 浏览: 711
要调整文本的颜色和透明度,你可以通过以下方式在Unity中更改Text组件的属性:
1. 打开Unity编辑器,进入你的场景。
2. 找到你想要更改颜色和透明度的Text组件。
3. 在Inspector面板中选择Text组件。
4. 在Text组件的属性面板中,找到“Color”选项。可以通过拖动RGB滑块来更改文本颜色。
5. 要更改透明度,请点击颜色选择器右侧的Alpha滑块,然后拖动滑块来更改透明度。
6. 你还可以通过代码来更改Text组件的颜色和透明度。例如,以下代码将文本颜色设置为红色,透明度设置为0.5:
```csharp
Text textComponent = GetComponent<Text>();
textComponent.color = new Color(1f, 0f, 0f, 0.5f);
```
这段代码首先获取Text组件,然后将其颜色设置为红色,透明度设置为0.5。
希望这能帮助到你!
相关问题
请帮我写一个能够在unity中实现检测到textmesh里的文字文本在透明度达到100时.文本能逐字出现的代码,并且告诉我该如何操作
### 回答1:
好的,以下是在Unity中实现文本逐字出现的代码:
```csharp
using UnityEngine;
using UnityEngine.UI;
using System.Collections;
public class TextAppear : MonoBehaviour {
public float letterPause = 0.2f;
public AudioClip sound;
string message;
Text textComp;
void Start () {
textComp = GetComponent<Text>();
message = textComp.text;
textComp.text = "";
StartCoroutine(TypeText ());
}
IEnumerator TypeText () {
foreach (char letter in message.ToCharArray()) {
textComp.text += letter;
if (sound)
AudioSource.PlayClipAtPoint(sound, transform.position);
yield return new WaitForSeconds (letterPause);
}
}
}
```
在透明度达到100时,可以在代码中添加以下内容:
```csharp
if (textComp.color.a == 1f) {
// Add code for letter by letter reveal here
}
```
然后将此代码附加到包含TextMesh组件的GameObject上即可。
操作步骤如下:
1. 创建一个空的GameObject,然后将TextMesh附加到其中。
2. 将上述代码复制并粘贴到新脚本中,并将新脚本附加到GameObject中。
3. 在文本字段中键入你所需的文本,然后调整文本颜色和透明度。
4. 运行场景并等待文本出现。
希望这能对你有帮助!
### 回答2:
在Unity中实现逐字出现的代码,需要以下几个步骤:
1. 创建一个3D文本对象:在Unity场景中创建一个TextMeshPro对象,该对象可以用来显示所需的文字。
2. 编写逐字出现的代码:在脚本文件中创建一个函数,用于逐字显示文本。在函数中,你可以使用循环来控制每个字符的显示时间和透明度。
以下是一个简短示例代码:
```csharp
using UnityEngine;
using TMPro;
public class TextAppear : MonoBehaviour
{
public TextMeshProUGUI textMesh; //将脚本拖拽到TextMeshPro对象上
private Coroutine appearCoroutine;
void Start()
{
appearCoroutine = StartCoroutine(ShowText());
}
IEnumerator ShowText()
{
textMesh.maxVisibleCharacters = 0;
while (textMesh.maxVisibleCharacters < textMesh.text.Length)
{
textMesh.maxVisibleCharacters += 1;
yield return new WaitForSeconds(0.1f); //每个字符显示的间隔时间
}
}
public void StopAppear()
{
if (appearCoroutine != null)
{
StopCoroutine(appearCoroutine);
}
}
}
```
3. 在场景中使用代码:将脚本挂载到文本对象上。在需要逐字显示文本的地方调用ShowText()函数即可开始逐字出现。如果需要停止逐字显示,使用StopAppear()函数。
希望以上代码和说明对你有帮助,如有其他问题请随时与我交流。
### 回答3:
在Unity中实现检测到TextMesh组件中的文字文本在透明度达到100时逐字出现的代码如下:
1. 首先,确保场景中有一个含有TextMesh组件的物体,该组件作为显示文字的容器。
2. 在脚本中添加以下代码:
```csharp
using UnityEngine;
public class TextFadeIn : MonoBehaviour
{
public TextMesh textMesh; // TextMesh组件
public float fadeInSpeed = 1f; // 文字出现的速度
private void Start()
{
textMesh.color = new Color(textMesh.color.r, textMesh.color.g, textMesh.color.b, 0f); // 设置TextMesh文本透明度为0
}
private void Update()
{
if (textMesh.color.a < 1f) // 如果TextMesh文本透明度小于1
{
float newAlpha = Mathf.Lerp(textMesh.color.a, 1f, fadeInSpeed * Time.deltaTime); // 使用Lerp进行渐变
textMesh.color = new Color(textMesh.color.r, textMesh.color.g, textMesh.color.b, newAlpha); // 更新TextMesh文本透明度
if (textMesh.color.a >= 1f) // 当文本透明度达到100时
{
// 逐字出现的实现代码
StartCoroutine(ShowText());
}
}
}
private System.Collections.IEnumerator ShowText()
{
int letterIndex = 0;
string text = textMesh.text;
while (letterIndex <= text.Length)
{
textMesh.text = text.Substring(0, letterIndex);
letterIndex++;
yield return new WaitForSeconds(0.1f); // 文字出现的间隔时间,根据需求调整
}
}
}
```
3. 给该脚本添加到场景中的TextMesh组件的容器物体上,并将相关参数进行设置和调整,如指定TextMesh组件、设定透明度变化速度等等。
4. 保存并运行场景,当TextMesh文本透明度达到100时,文字将逐字出现。
以上是实现在Unity中检测透明度达到100后,TextMesh文本逐字出现的代码和操作步骤。
请帮我写一个能够在unity中实现检测到textmesh里的文字文本在整体透明度达到100时,文本能逐字出现的代码,并且告诉我该如何操
作这段代码。
以下是Unity中实现文本逐字出现的代码:
1. 在Unity中创建一个TextMesh对象,并将其添加到场景中。
2. 在脚本中创建一个字符串变量,用于存储要逐字显示的文本。
3. 使用TextMesh组件的text属性将该字符串变量赋值给TextMesh对象,然后将TextMesh对象的颜色属性设置为透明。
4. 在Update()函数中,获取TextMesh组件的颜色属性,并检查它是否小于1.0。如果是,增加颜色属性的值。这将逐渐使文本变得可见。
5. 检查整个文本是否已经变得完全可见。如果是,取消将文本逐字显示的循环,并显示完整的文本。
以下是完整的代码:
```csharp
using UnityEngine;
using System.Collections;
public class TextFadeIn : MonoBehaviour {
public float fadeSpeed = 0.5f;
TextMesh textMesh;
string textToDisplay = "Hello, world!";
int currentCharacter = 0;
void Start () {
textMesh = GetComponent<TextMesh> ();
textMesh.text = "";
textMesh.color = new Color(textMesh.color.r, textMesh.color.g, textMesh.color.b, 0);
}
void Update () {
if(currentCharacter < textToDisplay.Length) {
textMesh.text += textToDisplay[currentCharacter];
currentCharacter++;
}
if(textMesh.color.a < 1.0f) {
textMesh.color = new Color(textMesh.color.r, textMesh.color.g, textMesh.color.b, textMesh.color.a + (fadeSpeed * Time.deltaTime));
}
if(currentCharacter == textToDisplay.Length && textMesh.color.a >= 1.0f) {
StopCoroutine(FadeTextToFullAlpha());
}
}
IEnumerator FadeTextToFullAlpha() {
while (textMesh.color.a < 1.0f) {
textMesh.color = new Color(textMesh.color.r, textMesh.color.g, textMesh.color.b, textMesh.color.a + (fadeSpeed * Time.deltaTime));
yield return null;
}
}
}
```
要使用此代码,请在Unity中创建一个TextMesh对象,并将其添加到场景中。然后将此脚本添加到TextMesh对象上。您可以通过更改fadeSpeed变量来调整文本淡入的速度,可以通过更改textToDisplay变量来设置要逐字显示的文本。
阅读全文
相关推荐
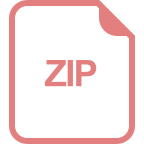
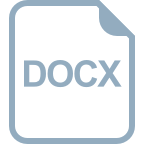
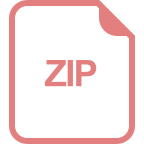
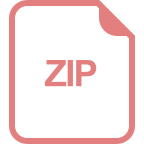
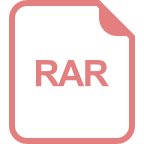
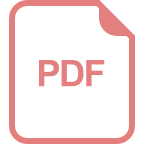
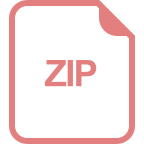
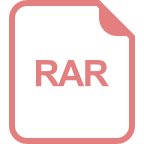
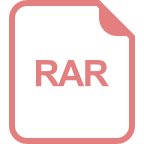
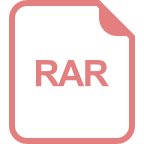
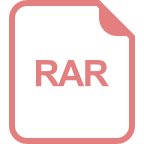
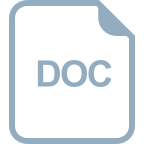


