public Date getPubdate() { return pubdate; }
时间: 2024-04-03 11:31:08 浏览: 12
根据代码中的实现来看,`getPubdate()` 是一个Java类中的公有方法(public method),用于获取该类中私有成员变量 `pubdate` 的值。由于方法返回值类型为 `Date`,因此可以猜测 `pubdate` 可能是一个日期类型的变量。该方法的作用是返回 `pubdate` 的值,供其他代码使用。
相关问题
使用注解的方式,实现对虚拟数据表 news(id(int),title(varcha(100)),author(varchar(50)),content(text),pubdate(date)) 进行操作的三层结构,写出测试程序
注解方式的三层结构包括:实体类、数据访问层(DAO)、业务逻辑层(Service)。以下是对应的代码示例:
实体类 News:
```java
public class News {
private int id;
private String title;
private String author;
private String content;
private Date pubdate;
public News() {}
public News(int id, String title, String author, String content, Date pubdate) {
this.id = id;
this.title = title;
this.author = author;
this.content = content;
this.pubdate = pubdate;
}
// getter and setter methods
}
```
数据访问层 NewsDAO:
```java
public interface NewsDAO {
@Select("SELECT * FROM news WHERE id=#{id}")
News findById(int id);
@Select("SELECT * FROM news ORDER BY pubdate DESC")
List<News> findAll();
@Insert("INSERT INTO news (title, author, content, pubdate) VALUES (#{title}, #{author}, #{content}, #{pubdate})")
int addNews(News news);
@Update("UPDATE news SET title=#{title}, author=#{author}, content=#{content}, pubdate=#{pubdate} WHERE id=#{id}")
int updateNews(News news);
@Delete("DELETE FROM news WHERE id=#{id}")
int deleteNews(int id);
}
```
业务逻辑层 NewsService:
```java
@Service
public class NewsService {
@Autowired
private NewsDAO newsDAO;
public News getNewsById(int id) {
return newsDAO.findById(id);
}
public List<News> getAllNews() {
return newsDAO.findAll();
}
public void addNews(News news) {
newsDAO.addNews(news);
}
public void updateNews(News news) {
newsDAO.updateNews(news);
}
public void deleteNews(int id) {
newsDAO.deleteNews(id);
}
}
```
测试程序 NewsTest:
```java
@RunWith(SpringRunner.class)
@SpringBootTest
public class NewsTest {
@Autowired
private NewsService newsService;
@Test
public void testCRUD() {
// 添加新闻
News news = new News(1, "Java 从入门到精通", "张三", "Java 是一门很好的语言", new Date());
newsService.addNews(news);
assertThat(news.getId()).isGreaterThan(0);
// 查询新闻
News savedNews = newsService.getNewsById(news.getId());
assertThat(savedNews.getTitle()).isEqualTo("Java 从入门到精通");
// 更新新闻
savedNews.setTitle("Java 从入门到放弃");
newsService.updateNews(savedNews);
News updatedNews = newsService.getNewsById(savedNews.getId());
assertThat(updatedNews.getTitle()).isEqualTo("Java 从入门到放弃");
// 删除新闻
newsService.deleteNews(updatedNews.getId());
News deletedNews = newsService.getNewsById(updatedNews.getId());
assertThat(deletedNews).isNull();
}
}
```
以上代码示例使用了 Spring Boot 和 MyBatis 框架,可根据实际情况进行调整。
用java实现图书借阅管理系统
图书借阅管理系统涉及到很多方面,包括图书、读者、借阅记录等等,因此需要设计多个实体类,还需要考虑到数据的持久化存储等问题。下面是一个简单的图书借阅管理系统的程序示例,供参考:
1.实体类设计
(1)图书类Book
```
public class Book {
private String id; //图书编号
private String name; //图书名称
private String author; //作者
private String pub; //出版社
private String pubDate;//出版日期
private int status; //借阅状态,0表示未借出,1表示已借出
public Book(){}
public Book(String id, String name, String author, String pub, String pubDate, int status) {
this.id = id;
this.name = name;
this.author = author;
this.pub = pub;
this.pubDate = pubDate;
this.status = status;
}
//getters and setters
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getPub() {
return pub;
}
public void setPub(String pub) {
this.pub = pub;
}
public String getPubDate() {
return pubDate;
}
public void setPubDate(String pubDate) {
this.pubDate = pubDate;
}
public int getStatus() {
return status;
}
public void setStatus(int status) {
this.status = status;
}
}
```
(2)读者类Reader
```
public class Reader {
private String id; //读者编号
private String name; //读者姓名
private String phoneNum; //联系电话
private String email; //电子邮件
private int maxNum; //最多借阅数量
private int borrowNum; //已借阅数量
public Reader(){}
public Reader(String id, String name, String phoneNum, String email, int maxNum, int borrowNum) {
this.id = id;
this.name = name;
this.phoneNum = phoneNum;
this.email = email;
this.maxNum = maxNum;
this.borrowNum = borrowNum;
}
//getters and setters
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getPhoneNum() {
return phoneNum;
}
public void setPhoneNum(String phoneNum) {
this.phoneNum = phoneNum;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public int getMaxNum() {
return maxNum;
}
public void setMaxNum(int maxNum) {
this.maxNum = maxNum;
}
public int getBorrowNum() {
return borrowNum;
}
public void setBorrowNum(int borrowNum) {
this.borrowNum = borrowNum;
}
}
```
(3)借阅记录类BorrowRecord
```
public class BorrowRecord {
private String id; //借阅记录编号
private String bookId; //图书编号
private String readerId; //读者编号
private String borrowDate; //借阅日期
private String returnDate; //归还日期
private int renewTimes; //续借次数
public BorrowRecord(){}
public BorrowRecord(String id, String bookId, String readerId, String borrowDate, String returnDate, int renewTimes) {
this.id = id;
this.bookId = bookId;
this.readerId = readerId;
this.borrowDate = borrowDate;
this.returnDate = returnDate;
this.renewTimes = renewTimes;
}
//getters and setters
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getBookId() {
return bookId;
}
public void setBookId(String bookId) {
this.bookId = bookId;
}
public String getReaderId() {
return readerId;
}
public void setReaderId(String readerId) {
this.readerId = readerId;
}
public String getBorrowDate() {
return borrowDate;
}
public void setBorrowDate(String borrowDate) {
this.borrowDate = borrowDate;
}
public String getReturnDate() {
return returnDate;
}
public void setReturnDate(String returnDate) {
this.returnDate = returnDate;
}
public int getRenewTimes() {
return renewTimes;
}
public void setRenewTimes(int renewTimes) {
this.renewTimes = renewTimes;
}
}
```
2.数据存储设计
这里使用MySQL数据库作为数据存储,需要新建三个表分别存储图书、读者和借阅记录。下面是三个表的SQL语句:
(1)图书表books
```
CREATE TABLE `books` (
`id` varchar(50) NOT NULL,
`name` varchar(100) NOT NULL,
`author` varchar(100) NOT NULL,
`pub` varchar(100) NOT NULL,
`pubDate` varchar(20) NOT NULL,
`status` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
(2)读者表readers
```
CREATE TABLE `readers` (
`id` varchar(50) NOT NULL,
`name` varchar(50) NOT NULL,
`phoneNum` varchar(20) NOT NULL,
`email` varchar(50) NOT NULL,
`maxNum` int(11) NOT NULL DEFAULT '5',
`borrowNum` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
(3)借阅记录表borrow_records
```
CREATE TABLE `borrow_records` (
`id` varchar(50) NOT NULL,
`bookId` varchar(50) NOT NULL,
`readerId` varchar(50) NOT NULL,
`borrowDate` varchar(20) NOT NULL,
`returnDate` varchar(20) DEFAULT NULL,
`renewTimes` int(11) NOT NULL DEFAULT '0',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```
3.程序实现
(1)添加图书
```
public void addBook(Book book){
try {
Connection conn = DBUtil.getConnection();
String sql = "insert into books(id, name, author, pub, pubDate) values(?,?,?,?,?)";
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, book.getId());
ps.setString(2, book.getName());
ps.setString(3, book.getAuthor());
ps.setString(4, book.getPub());
ps.setString(5, book.getPubDate());
ps.executeUpdate();
ps.close();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
(2)删除图书
```
public void deleteBook(String id){
try {
Connection conn = DBUtil.getConnection();
String sql = "delete from books where id=?";
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, id);
ps.executeUpdate();
ps.close();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
(3)修改图书信息
```
public void updateBook(Book book){
try {
Connection conn = DBUtil.getConnection();
String sql = "update books set name=?, author=?, pub=?, pubDate=?, status=? where id=?";
PreparedStatement ps = conn.prepareStatement(sql);
ps.setString(1, book.getName());
ps.setString(2, book.getAuthor());
ps.setString(3, book.getPub());
ps.setString(4, book.getPubDate());
ps.setInt(5, book.getStatus());
ps.setString(6, book.getId());
ps.executeUpdate();
ps.close();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
(4)查询图书信息
```
public List<Book> queryBooks(){
List<Book> books = new ArrayList<>();
try {
Connection conn = DBUtil.getConnection();
String sql = "select * from books";
PreparedStatement ps = conn.prepareStatement(sql);
ResultSet rs = ps.executeQuery();
while(rs.next()){
Book book = new Book();
book.setId(rs.getString("id"));
book.setName(rs.getString("name"));
book.setAuthor(rs.getString("author"));
book.setPub(rs.getString("pub"));
book.setPubDate(rs.getString("pubDate"));
book.setStatus(rs.getInt("status"));
books.add(book);
}
rs.close();
ps.close();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
return books;
}
```
(5)借阅图书
```
public void borrowBook(String bookId, String readerId){
try {
Connection conn = DBUtil.getConnection();
//判断该图书是否已经借出
String sql1 = "select status from books where id=?";
PreparedStatement ps1 = conn.prepareStatement(sql1);
ps1.setString(1, bookId);
ResultSet rs1 = ps1.executeQuery();
int status = 0;
if(rs1.next()){
status = rs1.getInt("status");
}
if(status == 1){
System.out.println("该图书已经借出!");
return;
}
//判断该读者是否还能借阅
String sql2 = "select maxNum, borrowNum from readers where id=?";
PreparedStatement ps2 = conn.prepareStatement(sql2);
ps2.setString(1, readerId);
ResultSet rs2 = ps2.executeQuery();
int maxNum = 0;
int borrowNum = 0;
if(rs2.next()){
maxNum = rs2.getInt("maxNum");
borrowNum = rs2.getInt("borrowNum");
}
if(borrowNum >= maxNum){
System.out.println("该读者已经达到最大借阅数量!");
return;
}
//更新图书状态和读者借阅数量
String sql3 = "update books set status=1 where id=?";
PreparedStatement ps3 = conn.prepareStatement(sql3);
ps3.setString(1, bookId);
ps3.executeUpdate();
String sql4 = "update readers set borrowNum=borrowNum+1 where id=?";
PreparedStatement ps4 = conn.prepareStatement(sql4);
ps4.setString(1, readerId);
ps4.executeUpdate();
//添加借阅记录
String id = UUID.randomUUID().toString();
Date date = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
String borrowDate = sdf.format(date);
String sql5 = "insert into borrow_records(id, bookId, readerId, borrowDate) values(?,?,?,?)";
PreparedStatement ps5 = conn.prepareStatement(sql5);
ps5.setString(1, id);
ps5.setString(2, bookId);
ps5.setString(3, readerId);
ps5.setString(4, borrowDate);
ps5.executeUpdate();
rs1.close();
ps1.close();
rs2.close();
ps2.close();
ps3.close();
ps4.close();
ps5.close();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
```
(6)归还图书
```
public void returnBook(String bookId, String readerId){
try {
Connection conn = DBUtil.getConnection();
//判断该图书是否已经借出
String sql1 = "select status from books where id=?";
PreparedStatement ps1 = conn.prepareStatement(sql1);
ps1.setString(1, bookId);
ResultSet rs1 = ps1.executeQuery();
int status = 0;
if(rs1.next()){
status = rs1.getInt("status");
}
if(status == 0){
System.out.println("该图书未借出!");
return;
}
//更新图书状态和读者借阅数量
String sql2 = "update books set status=0 where id=?";
PreparedStatement ps2 = conn.prepareStatement(sql2);
ps2.setString(1, bookId);
ps2.executeUpdate();
String sql3 = "update readers set borrowNum=borrowNum-1 where id=?";
PreparedStatement ps3 = conn.prepareStatement(sql3);
ps3.setString(1, readerId);
ps3.executeUpdate();
//更新借阅记录
String sql4 = "update borrow_records set returnDate=? where bookId=? and readerId=? and returnDate is null";
PreparedStatement ps4 = conn.prepareStatement(sql4);
Date date = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
String returnDate = sdf.format(date);
ps4.setString(1, returnDate);
ps4.setString(2, bookId);
ps4.setString(3, readerId);
ps4.executeUpdate();
//计算罚款金额
String sql5 = "select id, borrowDate, returnDate from borrow_records where bookId=? and readerId=? and returnDate is not null";
PreparedStatement ps5 = conn.prepareStatement(sql5);
ps5.setString(1, bookId);
ps5.setString(2, readerId);
ResultSet rs5 = ps5.executeQuery();
double fine = 0.0;
while(rs5.next()){
String id = rs5.getString("id");
String borrowDate = rs5.getString("borrowDate");
String returnDate = rs5.getString("returnDate");
long days = (sdf.parse(returnDate).getTime() - sdf.parse(borrowDate).getTime()) / (24 * 60 * 60 * 1000);
if(days > 30){
fine += (days - 30) * 0.1;
}
}
if(fine > 0){
System.out.println("该读者需要缴纳罚款:" + fine + "元。");
}
rs1.close();
ps1.close();
ps2.close();
ps3.close();
ps4.close();
rs5.close();
ps5.close();
conn.close();
} catch (SQLException | ParseException e) {
e.printStackTrace();
}
}
```
(7)查询借阅记录
```
public List<BorrowRecord> queryBorrowRecords(){
List<BorrowRecord> records = new ArrayList<>();
try {
Connection conn = DBUtil.getConnection();
String sql = "select * from borrow_records";
PreparedStatement ps = conn.prepareStatement(sql);
ResultSet rs = ps.executeQuery();
while(rs.next()){
BorrowRecord record = new BorrowRecord();
record.setId(rs.getString("id"));
record.setBookId(rs.getString("bookId"));
record.setReaderId(rs.getString("readerId"));
record.setBorrowDate(rs.getString("borrowDate"));
record.setReturnDate(rs.getString("returnDate"));
record.setRenewTimes(rs.getInt("renewTimes"));
records.add(record);
}
rs.close();
ps.close();
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
return records;
}
```
以上是一个简单的图书借阅管理系统的程序示例,实际应用中还需要考虑到很多细节问题,比如图书和读者信息的修改和删除、借阅记录的续借和归还等等。
相关推荐
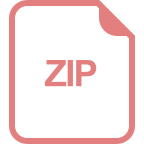
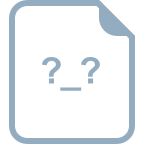






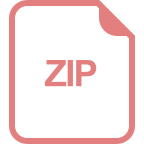
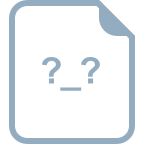
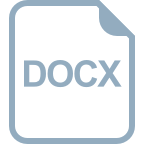
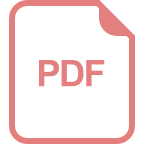
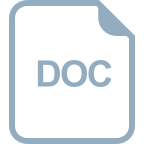
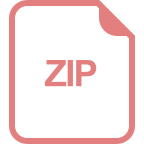