java批量调用第三方接口的方法
时间: 2023-11-04 20:59:00 浏览: 140
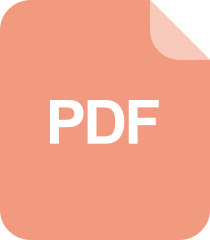
Java调用第三方接口示范的实现
Java批量调用第三方接口的方法可以使用以下步骤:
1. 创建一个HTTP客户端,如Apache HttpClient或OkHttp。
2. 创建一个HTTP请求对象,设置请求方法、请求URL、请求头和请求体等信息。
3. 遍历需要调用的接口列表,对于每个接口,设置请求参数和请求体等信息,将HTTP请求对象发送给第三方接口。
4. 接收第三方接口返回的HTTP响应对象,解析响应头和响应体,处理响应结果。
5. 将每个接口的响应结果保存到一个集合中,最后返回该集合。
以下是一个简单的示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class BatchCallApi {
public static void main(String[] args) throws IOException {
List<String> apiList = new ArrayList<>();
apiList.add("http://api.example.com/api1");
apiList.add("http://api.example.com/api2");
apiList.add("http://api.example.com/api3");
List<String> resultList = batchCallApi(apiList, "{\"param1\":\"value1\",\"param2\":\"value2\"}", "UTF-8");
for (String result : resultList) {
System.out.println(result);
}
}
public static List<String> batchCallApi(List<String> apiList, String requestBody, String charset) throws IOException {
List<String> resultList = new ArrayList<>();
HttpClient httpClient = HttpClients.createDefault();
for (String api : apiList) {
HttpPost httpPost = new HttpPost(api);
StringEntity stringEntity = new StringEntity(requestBody, charset);
httpPost.setEntity(stringEntity);
httpPost.setHeader("Content-Type", "application/json;charset=" + charset);
HttpResponse httpResponse = httpClient.execute(httpPost);
HttpEntity httpEntity = httpResponse.getEntity();
String result = EntityUtils.toString(httpEntity, charset);
resultList.add(result);
}
return resultList;
}
}
```
在这个示例中,我们使用Apache HttpClient作为HTTP客户端,遍历了一个包含三个API地址的列表,对于每个API地址,设置了请求参数和请求体,发送HTTP请求,接收HTTP响应,解析响应结果并保存到一个集合中,最后返回该集合。
阅读全文
相关推荐

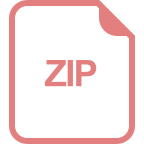

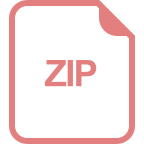
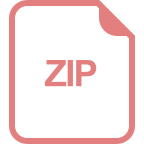
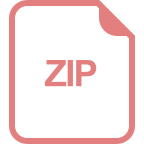
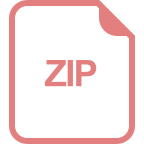
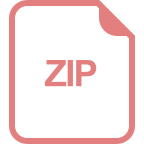
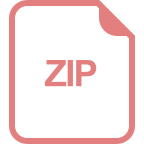
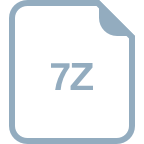
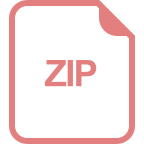
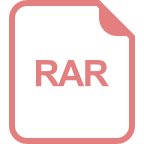
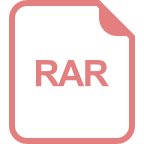
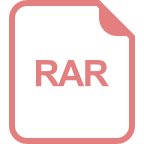
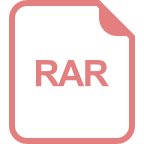