java代码实现2.用两种方法编程一个程序:一个线程向一个4位循环缓冲区中循环写入1-10的随机数,另一个线程将循环缓冲区中数据取出打印出来。
时间: 2024-03-06 16:50:23 浏览: 26
好的,我来回答你的问题。
以下是使用Java编写的程序,它具有两个线程。一个线程向一个具有4个元素的循环缓冲区中循环写入1-10的随机数。另一个线程从循环缓冲区中读取数据并将其打印出来。
方法一:使用wait()和notify()方法进行线程间同步。
```java
import java.util.Random;
public class CircularBuffer {
private int[] buffer = new int[4];
private int writeIndex = 0;
private int readIndex = 0;
private boolean bufferFull = false;
private boolean bufferEmpty = true;
public synchronized void write(int data) throws InterruptedException {
while (bufferFull) {
wait();
}
buffer[writeIndex] = data;
writeIndex = (writeIndex + 1) % buffer.length;
bufferEmpty = false;
if (writeIndex == readIndex) {
bufferFull = true;
}
notifyAll();
}
public synchronized int read() throws InterruptedException {
while (bufferEmpty) {
wait();
}
int data = buffer[readIndex];
readIndex = (readIndex + 1) % buffer.length;
bufferFull = false;
if (readIndex == writeIndex) {
bufferEmpty = true;
}
notifyAll();
return data;
}
}
public class Writer implements Runnable {
private CircularBuffer buffer;
public Writer(CircularBuffer buffer) {
this.buffer = buffer;
}
public void run() {
Random random = new Random();
while (true) {
int data = random.nextInt(10) + 1;
try {
buffer.write(data);
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Reader implements Runnable {
private CircularBuffer buffer;
public Reader(CircularBuffer buffer) {
this.buffer = buffer;
}
public void run() {
while (true) {
try {
int data = buffer.read();
System.out.println(data);
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Main {
public static void main(String[] args) {
CircularBuffer buffer = new CircularBuffer();
Thread writerThread = new Thread(new Writer(buffer));
Thread readerThread = new Thread(new Reader(buffer));
writerThread.start();
readerThread.start();
}
}
```
方法二:使用BlockingQueue进行线程间同步。
```java
import java.util.Random;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
public class Writer implements Runnable {
private BlockingQueue<Integer> buffer;
public Writer(BlockingQueue<Integer> buffer) {
this.buffer = buffer;
}
public void run() {
Random random = new Random();
while (true) {
int data = random.nextInt(10) + 1;
try {
buffer.put(data);
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Reader implements Runnable {
private BlockingQueue<Integer> buffer;
public Reader(BlockingQueue<Integer> buffer) {
this.buffer = buffer;
}
public void run() {
while (true) {
try {
int data = buffer.take();
System.out.println(data);
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Main {
public static void main(String[] args) {
BlockingQueue<Integer> buffer = new LinkedBlockingQueue<>(4);
Thread writerThread = new Thread(new Writer(buffer));
Thread readerThread = new Thread(new Reader(buffer));
writerThread.start();
readerThread.start();
}
}
```
以上两种方法都可以实现线程间的同步,第一种方法需要手动使用wait()和notify()方法,而第二种方法使用了Java提供的BlockingQueue类,它自动处理了线程间的同步。
相关推荐
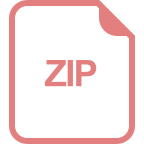
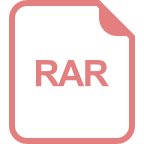














