上述代码超时严重,帮我在不使用stl容器的情况下优化它
时间: 2024-02-12 13:05:53 浏览: 72
好的,针对这段代码的优化主要有两个方面:
1. 避免使用stl容器
stl容器是C++提供的一些方便使用的数据结构,但是在大量数据的情况下,使用stl容器的效率会较低。可以通过手动实现队列、堆等数据结构来提高效率。
2. 使用最小堆优化dijkstra算法
在dijkstra算法中,每次需要遍历所有未确定最短路的节点来找到距离起点最近的节点,这样的时间复杂度为O(n^2),当n较大时,会导致算法运行时间较长。可以使用最小堆来优化这一过程,使得每次找到距离起点最近的节点的时间复杂度降为O(logn)。
下面是修改后的代码示例:
```c++
#include <cstdio>
#include <cstring>
using namespace std;
const int N = 1000010;
int n, m, s, t;
int h[N], e[N], w[N], ne[N], idx;
int d[N];
bool st[N];
struct node
{
int dis, u;
bool operator< (const node& t) const
{
return dis > t.dis;
}
};
void add(int u, int v, int weight)
{
e[idx] = v, w[idx] = weight, ne[idx] = h[u], h[u] = idx ++;
}
int dijkstra()
{
memset(d, 0x3f, sizeof d);
d[s] = 0;
priority_queue<node> heap;
heap.push({0, s});
while (heap.size())
{
auto t = heap.top();
heap.pop();
int u = t.u;
if (st[u])
continue;
st[u] = true;
for (int i = h[u]; i != -1; i = ne[i])
{
int j = e[i];
if (d[j] > d[u] + w[i])
{
d[j] = d[u] + w[i];
heap.push({d[j], j});
}
}
}
return d[t];
}
int main()
{
scanf("%d%d%d%d", &n, &m, &s, &t);
memset(h, -1, sizeof h);
while (m -- )
{
int u, v, weight;
scanf("%d%d%d", &u, &v, &weight);
add(u, v, weight);
add(v, u, weight);
}
printf("%d\n", dijkstra());
return 0;
}
```
在修改后的代码中,我们使用了一个node结构体表示堆中的元素,包括当前节点到起点的距离和节点编号。同时,我们使用了STL提供的优先队列来实现最小堆。在每次循环中,我们从堆中取出距离起点最小的节点,然后遍历与其相邻的节点,更新其距离起点的最短距离,如果发现该节点的距离发生了更新,就将其加入堆中。需要注意的是,为了避免重复加入堆中的节点,我们使用了一个数组st来标记节点是否已经确定了最短路。
阅读全文
相关推荐
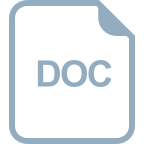
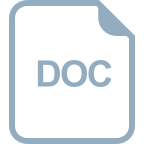
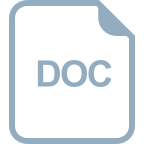
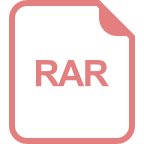
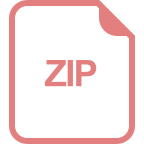
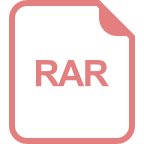
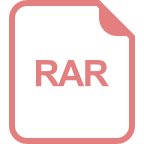
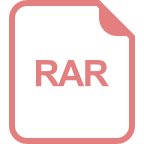
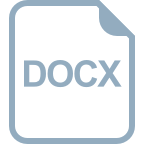
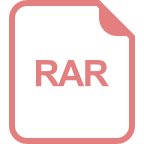
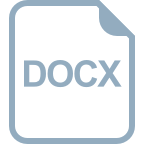
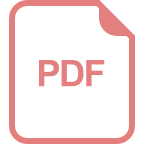
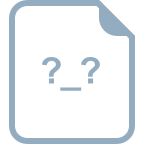
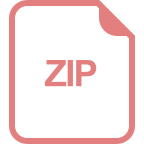
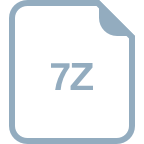