parallel list C#
时间: 2023-07-27 21:08:22 浏览: 40
In C#, a parallel list refers to the concept of having multiple lists that are synchronized, meaning that the elements at corresponding positions in different lists are related to each other. One way to achieve this is by using the `Tuple` class or defining a custom class with multiple properties.
Here's an example of using parallel lists with `Tuple`:
```csharp
List<int> numbers = new List<int> { 1, 2, 3 };
List<string> words = new List<string> { "one", "two", "three" };
List<Tuple<int, string>> parallelList = new List<Tuple<int, string>>();
for (int i = 0; i < numbers.Count; i++)
{
parallelList.Add(Tuple.Create(numbers[i], words[i]));
}
foreach (var item in parallelList)
{
Console.WriteLine($"Number: {item.Item1}, Word: {item.Item2}");
}
```
In this example, we have two lists (`numbers` and `words`) containing related data. We create a third list (`parallelList`) that holds tuples, where each tuple contains an element from `numbers` and `words` at the same index. We then iterate over `parallelList` and display the corresponding elements from each list.
Note that starting from C# 7, you can use value tuples instead of `Tuple`. The syntax and usage are slightly different but serve a similar purpose.
相关推荐
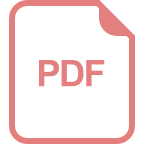
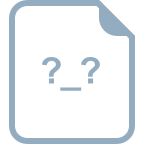
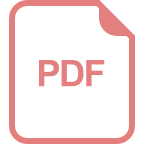















