用python搭建bp神经网络
时间: 2023-11-13 17:06:17 浏览: 36
构建BP神经网络主要分为以下几个步骤:
1. 定义神经网络的结构:输入层、隐藏层和输出层,每一层的神经元数量可以自行设置;
2. 初始化神经网络的权重和偏置;
3. 定义激活函数,常用的有sigmoid、ReLU等;
4. 通过前向传播计算输出值;
5. 计算误差,常用的误差函数有均方误差(MSE)和交叉熵(Cross-Entropy);
6. 反向传播计算梯度,更新权重和偏置。
下面是一个简单的BP神经网络的实现代码:
```python
import numpy as np
class NeuralNetwork:
def __init__(self, input_dim, hidden_dim, output_dim):
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.output_dim = output_dim
# 初始化权重和偏置
self.W1 = np.random.randn(input_dim, hidden_dim)
self.b1 = np.zeros(hidden_dim)
self.W2 = np.random.randn(hidden_dim, output_dim)
self.b2 = np.zeros(output_dim)
def sigmoid(self, x):
return 1 / (1 + np.exp(-x))
def forward(self, x):
# 前向传播计算输出值
self.z1 = np.dot(x, self.W1) + self.b1
self.a1 = self.sigmoid(self.z1)
self.z2 = np.dot(self.a1, self.W2) + self.b2
self.a2 = self.sigmoid(self.z2)
return self.a2
def sigmoid_derivative(self, x):
return x * (1 - x)
def backward(self, x, y, output):
# 反向传播计算梯度
self.delta2 = (output - y) * self.sigmoid_derivative(output)
self.delta1 = np.dot(self.delta2, self.W2.T) * self.sigmoid_derivative(self.a1)
dW2 = np.dot(self.a1.T, self.delta2)
db2 = np.sum(self.delta2, axis=0)
dW1 = np.dot(x.T, self.delta1)
db1 = np.sum(self.delta1, axis=0)
# 更新权重和偏置
self.W2 -= 0.1 * dW2
self.b2 -= 0.1 * db2
self.W1 -= 0.1 * dW1
self.b1 -= 0.1 * db1
def train(self, X, y):
output = self.forward(X)
self.backward(X, y, output)
```
其中,`input_dim`、`hidden_dim`和`output_dim`分别表示输入层、隐藏层和输出层的神经元数量,`W1`、`b1`、`W2`和`b2`分别表示输入层到隐藏层、隐藏层到输出层的权重和偏置,`sigmoid`函数为激活函数,`forward`函数为前向传播的实现,`backward`函数为反向传播的实现,`train`函数则是整个模型的训练过程。
相关推荐
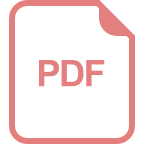
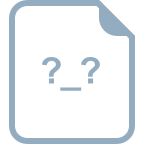














