二、职工信息管理系统设计 总体需求 职工信息包括职工号、姓名、性别、出生年月、学历、职务、工资、住址、电话等(职工号不重复)。所有信息用文件保存。 功能要求: (1)系统以菜单方式工作 (2)职工信息录入功能(职工信息用文件保存)——输入 (3)职工信息浏览功能——输出 (4)查询或排序功能:按工资查询,按学历查询等 (5)职工信息删除功能 (6)职工信息修改功能 (7)按照某种信息进行排序显示(比如工资从低 到高等)用c语言实现
时间: 2023-12-19 18:11:18 浏览: 257
以下是一个简单的职工信息管理系统的设计,实现了菜单、录入、浏览、查询、删除、修改和排序等功能。具体实现细节可以根据需要进行修改和完善。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_EMPLOYEES 100
#define MAX_NAME_LEN 20
#define MAX_ADDRESS_LEN 50
#define MAX_PHONE_LEN 15
// 职工信息结构体
typedef struct {
int id;
char name[MAX_NAME_LEN];
char gender;
int year, month, day;
char education[10];
char position[20];
int salary;
char address[MAX_ADDRESS_LEN];
char phone[MAX_PHONE_LEN];
} Employee;
// 全局变量,存储职工信息
Employee employees[MAX_EMPLOYEES];
int num_employees = 0;
// 函数声明
void input_employee();
void browse_employees();
void search_by_salary();
void search_by_education();
void delete_employee();
void modify_employee();
void sort_employees();
// 主函数
int main() {
int choice;
while (1) {
printf("\n职工信息管理系统\n");
printf("1. 录入职工信息\n");
printf("2. 浏览职工信息\n");
printf("3. 按工资查询\n");
printf("4. 按学历查询\n");
printf("5. 删除职工信息\n");
printf("6. 修改职工信息\n");
printf("7. 按工资排序\n");
printf("8. 退出系统\n");
printf("请选择操作:");
scanf("%d", &choice);
switch (choice) {
case 1:
input_employee();
break;
case 2:
browse_employees();
break;
case 3:
search_by_salary();
break;
case 4:
search_by_education();
break;
case 5:
delete_employee();
break;
case 6:
modify_employee();
break;
case 7:
sort_employees();
break;
case 8:
printf("感谢使用职工信息管理系统!\n");
exit(0);
default:
printf("输入错误,请重新选择操作!\n");
break;
}
}
}
// 录入职工信息
void input_employee() {
if (num_employees >= MAX_EMPLOYEES) {
printf("职工信息已满,无法继续录入!\n");
return;
}
Employee e;
printf("请输入职工号:");
scanf("%d", &e.id);
for (int i = 0; i < num_employees; i++) {
if (employees[i].id == e.id) {
printf("职工号已存在,请重新输入!\n");
return;
}
}
printf("请输入姓名:");
scanf("%s", e.name);
printf("请输入性别(M/F):");
scanf(" %c", &e.gender);
printf("请输入出生年月(YYYY MM DD):");
scanf("%d%d%d", &e.year, &e.month, &e.day);
printf("请输入学历:");
scanf("%s", e.education);
printf("请输入职务:");
scanf("%s", e.position);
printf("请输入工资:");
scanf("%d", &e.salary);
printf("请输入住址:");
scanf("%s", e.address);
printf("请输入电话:");
scanf("%s", e.phone);
employees[num_employees++] = e;
printf("职工信息录入成功!\n");
}
// 浏览职工信息
void browse_employees() {
if (num_employees == 0) {
printf("职工信息为空,请先录入职工信息!\n");
return;
}
printf("职工号\t姓名\t性别\t出生日期\t学历\t职务\t工资\t住址\t电话\n");
for (int i = 0; i < num_employees; i++) {
printf("%d\t%s\t%c\t%d-%02d-%02d\t%s\t%s\t%d\t%s\t%s\n",
employees[i].id, employees[i].name, employees[i].gender,
employees[i].year, employees[i].month, employees[i].day,
employees[i].education, employees[i].position,
employees[i].salary, employees[i].address, employees[i].phone);
}
}
// 按工资查询
void search_by_salary() {
int salary;
printf("请输入工资:");
scanf("%d", &salary);
printf("职工号\t姓名\t性别\t出生日期\t学历\t职务\t工资\t住址\t电话\n");
for (int i = 0; i < num_employees; i++) {
if (employees[i].salary == salary) {
printf("%d\t%s\t%c\t%d-%02d-%02d\t%s\t%s\t%d\t%s\t%s\n",
employees[i].id, employees[i].name, employees[i].gender,
employees[i].year, employees[i].month, employees[i].day,
employees[i].education, employees[i].position,
employees[i].salary, employees[i].address, employees[i].phone);
}
}
}
// 按学历查询
void search_by_education() {
char education[10];
printf("请输入学历:");
scanf("%s", education);
printf("职工号\t姓名\t性别\t出生日期\t学历\t职务\t工资\t住址\t电话\n");
for (int i = 0; i < num_employees; i++) {
if (strcmp(employees[i].education, education) == 0) {
printf("%d\t%s\t%c\t%d-%02d-%02d\t%s\t%s\t%d\t%s\t%s\n",
employees[i].id, employees[i].name, employees[i].gender,
employees[i].year, employees[i].month, employees[i].day,
employees[i].education, employees[i].position,
employees[i].salary, employees[i].address, employees[i].phone);
}
}
}
// 删除职工信息
void delete_employee() {
int id;
printf("请输入要删除的职工号:");
scanf("%d", &id);
for (int i = 0; i < num_employees; i++) {
if (employees[i].id == id) {
for (int j = i; j < num_employees - 1; j++) {
employees[j] = employees[j + 1];
}
num_employees--;
printf("职工信息删除成功!\n");
return;
}
}
printf("职工信息不存在,请重新输入!\n");
}
// 修改职工信息
void modify_employee() {
int id;
printf("请输入要修改的职工号:");
scanf("%d", &id);
for (int i = 0; i < num_employees; i++) {
if (employees[i].id == id) {
printf("请输入姓名:");
scanf("%s", employees[i].name);
printf("请输入性别(M/F):");
scanf(" %c", &employees[i].gender);
printf("请输入出生年月(YYYY MM DD):");
scanf("%d%d%d", &employees[i].year, &employees[i].month, &employees[i].day);
printf("请输入学历:");
scanf("%s", employees[i].education);
printf("请输入职务:");
scanf("%s", employees[i].position);
printf("请输入工资:");
scanf("%d", &employees[i].salary);
printf("请输入住址:");
scanf("%s", employees[i].address);
printf("请输入电话:");
scanf("%s", employees[i].phone);
printf("职工信息修改成功!\n");
return;
}
}
printf("职工信息不存在,请重新输入!\n");
}
// 按工资排序
void sort_employees() {
for (int i = 0; i < num_employees - 1; i++) {
for (int j = 0; j < num_employees - i - 1; j++) {
if (employees[j].salary > employees[j + 1].salary) {
Employee temp = employees[j];
employees[j] = employees[j + 1];
employees[j + 1] = temp;
}
}
}
browse_employees();
}
```
该程序使用了一个结构体 `Employee` 来存储职工信息,使用了全局变量 `employees` 和 `num_employees` 来存储职工信息和职工人数。各个功能函数的实现比较简单明了,可以根据需要进行修改和扩展。
阅读全文
相关推荐
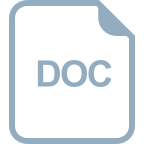
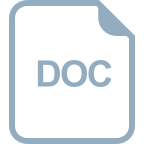
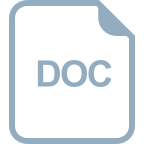






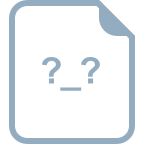
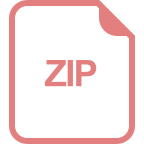
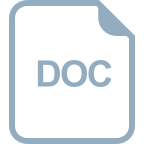
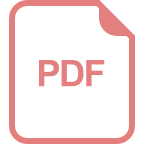
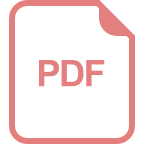
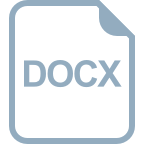
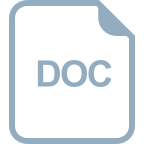


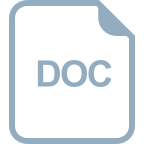