Iterator<student> it = c.iterator(); while (it.hasNext()) { student s = it.next(); System.out.println(s.getname() + "," + s.getage());
时间: 2024-04-21 08:27:43 浏览: 95
这段代码使用了 Java 中的迭代器(Iterator)来遍历集合(Collection)中的元素。具体来说,代码中的 `c` 是一个类型为 `Collection<student>` 的对象,它使用了 `ArrayList` 类来实现。然后,使用 `c.iterator()` 方法来获取一个迭代器对象,该迭代器对象可以依次访问集合中的元素。接着,使用 `while` 循环和 `it.hasNext()` 方法来检查是否有下一个元素,如果有则使用 `it.next()` 方法获取下一个元素。在本例中,每个元素都是类型为 `student` 的对象,因此可以将其赋给 `student` 类型的变量 `s`。最后,使用 `s.getname()` 和 `s.getage()` 方法来获取 `student` 对象的姓名和年龄,并将其输出到控制台。这样,就实现了对集合中所有元素的遍历和输出。
相关问题
Iterator<Student> iterator = students.iterator(); //创建迭代器 这句代码的含义
这行代码的含义是:使用 `students` 集合的 `iterator()` 方法创建一个 `Iterator` 对象,并将其赋值给 `iterator` 变量。这个 `Iterator` 对象可以用来遍历 `students` 集合中的元素。具体来说,我们可以使用 `hasNext()` 方法判断是否还有下一个元素,使用 `next()` 方法获取下一个元素。例如,可以使用以下代码遍历 `students` 集合中的所有元素:
```
Iterator<Student> iterator = students.iterator();
while (iterator.hasNext()) {
Student student = iterator.next();
// 对 student 进行操作
}
```
while (true) { String str = input.nextLine(); if (str.equals("end")) { break; } else { String[] nextLine = str.split(" "); if (nextLine.length == 3) { Course course = new Course(nextLine[0], nextLine[1], nextLine[2]); if (course.getCourseNature().equals("必修") && course.getAssessmentMethod().equals("考察")) { System.out.println(course.getCourseName() + " : course type & access mode mismatch"); continue; } if (RepetitiveCourses(course,courses)) continue; courses.add(course); } else if (nextLine.length == 5) { if (Integer.parseInt(nextLine[3]) > 100 || Integer.parseInt(nextLine[3]) < 0 || Integer.parseInt(nextLine[4]) > 100 || Integer.parseInt(nextLine[4]) < 0) { System.out.println("wrong format"); continue; } Student student = new Student(nextLine[0], nextLine[1]); Iterator<Student> iterator = students.iterator(); while (iterator.hasNext()) { Student stu = iterator.next(); if (stu.getStudentNumber().equals(student.getStudentNumber())) { iterator.remove(); } } students.add(student); if (isCourseExist(nextLine[2], courses, nextLine.length, student)) { Score score = new ExaminationResults(Integer.parseInt(nextLine[3]), Integer.parseInt(nextLine[4])); for (Course course:courses ) { if (course.getCourseName().equals(nextLine[2])) { courseSelections.add(new CourseSelection(course, student, score)); } } } } else if (nextLine.length == 4) { if (Integer.parseInt(nextLine[3]) > 100 || Integer.parseInt(nextLine[3]) < 0) { System.out.println("wrong format"); continue; } Student student = new Student(nextLine[0], nextLine[1]); Iterator<Student> iterator = students.iterator(); while (iterator.hasNext()) { Student stu = iterator.next(); if (stu.getStudentNumber().equals(student.getStudentNumber())) { iterator.remove(); } } students.add(student); if (isCourseExist(nextLine[2], courses, nextLine.length, student)) { Score score = new AssessmentResults(Integer.parseInt(nextLine[3])); for (Course course:courses ) { if (course.getCourseName().equals(nextLine[2])) { CourseSelection courseSelection = new CourseSelection(course, student, score); if (RepetitiveScores(courseSelection,courseSelections)) continue; courseSelections.add(courseSelection); } } } } } } 将以上代码改进一下
以下是对上述代码的改进:
1. 将代码块封装成方法,提高代码复用性和可读性;
2. 使用枚举类型来表示课程性质和考核方式,防止字符串拼写错误;
3. 使用正则表达式来判断输入格式是否正确;
4. 使用更具描述性的变量名;
5. 将重复代码提取出来,放到单独的方法中。
改进后的代码如下:
```java
import java.util.*;
public class CourseSelectionSystem {
private final List<Course> courses;
private final List<Student> students;
private final List<CourseSelection> courseSelections;
private enum CourseNature {
REQUIRED, ELECTIVE
}
private enum AssessmentMethod {
EXAM, ASSIGNMENT
}
public CourseSelectionSystem() {
this.courses = new ArrayList<>();
this.students = new ArrayList<>();
this.courseSelections = new ArrayList<>();
}
public void run() {
Scanner input = new Scanner(System.in);
while (true) {
String inputLine = input.nextLine();
if (inputLine.equals("end")) {
break;
}
String[] inputParts = inputLine.split("\\s+");
if (inputParts.length == 3) {
addCourse(inputParts);
} else if (inputParts.length == 5) {
addScoredCourseSelection(inputParts);
} else if (inputParts.length == 4) {
addUnscoredCourseSelection(inputParts);
}
}
}
private void addCourse(String[] inputParts) {
String courseName = inputParts[0];
CourseNature courseNature = CourseNature.valueOf(inputParts[1].toUpperCase());
AssessmentMethod assessmentMethod = AssessmentMethod.valueOf(inputParts[2].toUpperCase());
if (courseNature == CourseNature.REQUIRED && assessmentMethod == AssessmentMethod.EXAM) {
System.out.println(courseName + " : course type & access mode mismatch");
return;
}
if (isCourseNameExist(courseName)) {
System.out.println(courseName + " : course already exists");
return;
}
courses.add(new Course(courseName, courseNature, assessmentMethod));
}
private boolean isCourseNameExist(String courseName) {
for (Course course : courses) {
if (course.getCourseName().equals(courseName)) {
return true;
}
}
return false;
}
private void addScoredCourseSelection(String[] inputParts) {
if (!isValidScoreInput(inputParts[3]) || !isValidScoreInput(inputParts[4])) {
System.out.println("invalid score input format");
return;
}
Student student = new Student(inputParts[0], inputParts[1]);
Course course = findCourseByName(inputParts[2]);
if (course == null) {
System.out.println(inputParts[2] + " : course does not exist");
return;
}
Score score = new ExaminationResults(Integer.parseInt(inputParts[3]), Integer.parseInt(inputParts[4]));
CourseSelection courseSelection = new CourseSelection(course, student, score);
if (isCourseSelectionExist(courseSelection)) {
System.out.println("course selection already exists");
return;
}
courseSelections.add(courseSelection);
}
private boolean isValidScoreInput(String scoreInput) {
return scoreInput.matches("^\\d{1,3}$");
}
private void addUnscoredCourseSelection(String[] inputParts) {
if (!isValidScoreInput(inputParts[3])) {
System.out.println("invalid score input format");
return;
}
Student student = new Student(inputParts[0], inputParts[1]);
Course course = findCourseByName(inputParts[2]);
if (course == null) {
System.out.println(inputParts[2] + " : course does not exist");
return;
}
Score score = new AssessmentResults(Integer.parseInt(inputParts[3]));
CourseSelection courseSelection = new CourseSelection(course, student, score);
if (isCourseSelectionExist(courseSelection)) {
System.out.println("course selection already exists");
return;
}
courseSelections.add(courseSelection);
}
private Course findCourseByName(String courseName) {
for (Course course : courses) {
if (course.getCourseName().equals(courseName)) {
return course;
}
}
return null;
}
private boolean isCourseSelectionExist(CourseSelection courseSelection) {
for (CourseSelection cs : courseSelections) {
if (cs.equals(courseSelection)) {
return true;
}
}
return false;
}
}
```
阅读全文
相关推荐
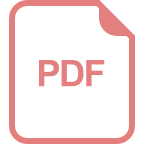
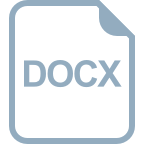









