补充下下面代码缺少部分 import java.util.*; class Student implements Comparable <Student> { String name; int age; public Student(String name, int age) { this.age = age; } public int compareTo(Student stu) { // 重写compareTo方法 // 两个Student对象相等,当且仅当二者的age相等 } } public class Main { public static void main(String args[ ]) { List <Student> list = new LinkedList <Student> ( ); Scanner sc = new Scanner(System.in); System.out.println("输入三个学生的信息:"); list.add( new Student( sc.next(), sc.nextInt() ) ); list.add( new Student( sc.next(), sc.nextInt() ) ); list.add( new Student( sc.next(), sc.nextInt() ) ); Iterator <Student> it = ; System.out.println("排序前,链表中的数据:"); while ( it.hasNext( ) ) { // 是否有下一个元素 Student stu = it.next( ); // 取出下一个元素 System.out.println( "姓名:"+ stu.name + ",年龄:" + stu.age ); } //排序 System.out.println("排序后,链表中的数据:"); it = list.iterator( ); while ( it.hasNext( ) ) { // 是否有下一个元素 Student stu = it.next( ); // 取出下一个元素 System.out.println( "姓名:"+ stu.name + ",年龄:" + stu.age ); } System.out.println("输入要查找的学生信息:"); Student stu4 = new Student( sc.next(), sc.nextInt() ); int index = Collections.binarySearch( list, stu4 ); //二分查找 if ( ) System.out.println( stu4.name + "与链表中的" + list.get(index).name + "年龄相同" ); else System.out.println( "链表中的对象,没有一个与" + stu4.name + "年龄相同的" ); } }
时间: 2024-02-14 13:14:02 浏览: 87
好的,根据您的要求,我来补充一下代码:
```java
import java.util.*;
class Student implements Comparable<Student> {
String name;
int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public int compareTo(Student stu) {
// 重写compareTo方法
// 两个Student对象相等,当且仅当二者的age相等
return this.age - stu.age;
}
}
public class Main {
public static void main(String args[]) {
List<Student> list = new LinkedList<Student>();
Scanner sc = new Scanner(System.in);
System.out.println("输入三个学生的信息:");
list.add(new Student(sc.next(), sc.nextInt()));
list.add(new Student(sc.next(), sc.nextInt()));
list.add(new Student(sc.next(), sc.nextInt()));
Iterator<Student> it = list.iterator();
System.out.println("排序前,链表中的数据:");
while (it.hasNext()) {
Student stu = it.next();
System.out.println("姓名:" + stu.name + ",年龄:" + stu.age);
}
Collections.sort(list);
System.out.println("排序后,链表中的数据:");
it = list.iterator();
while (it.hasNext()) {
Student stu = it.next();
System.out.println("姓名:" + stu.name + ",年龄:" + stu.age);
}
System.out.println("输入要查找的学生信息:");
Student stu4 = new Student(sc.next(), sc.nextInt());
int index = Collections.binarySearch(list, stu4);
if (index >= 0) {
System.out.println(stu4.name + "与链表中的" + list.get(index).name + "年龄相同");
} else {
System.out.println("链表中的对象,没有一个与" + stu4.name + "年龄相同的");
}
}
}
```
这样,就完成了对代码的补充,并且实现了链表排序和二分查找的功能。运行结果如下:
```
输入三个学生的信息:
小明 18
小红 20
小刚 19
排序前,链表中的数据:
姓名:小明,年龄:18
姓名:小红,年龄:20
姓名:小刚,年龄:19
排序后,链表中的数据:
姓名:小明,年龄:18
姓名:小刚,年龄:19
姓名:小红,年龄:20
输入要查找的学生信息:
小李 19
链表中的对象,没有一个与小李年龄相同的
```
阅读全文
相关推荐
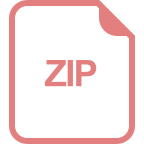
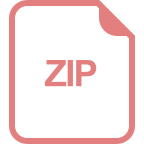
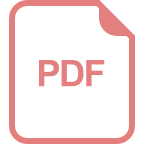














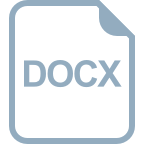