用JavaSort the Student list by ascending order of student namesThe following is an example illustrating formatting output. You will need it for task 2 and 3. public class Main { public static void main(String[] args) { String string1 = "Hello"; String string2 = "World"; String string3 = "Java"; System.out.println(String.format("%-10s %-10s %-10s", string1, string2, string3)); } }
时间: 2024-03-03 20:50:30 浏览: 31
Sure, here's an example Java code that sorts a list of students by ascending order of their names using the `Collections.sort()` method and formats the output using `String.format()`:
```java
import java.util.ArrayList;
import java.util.Collections;
public class Student implements Comparable<Student> {
private String name;
private int age;
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public int compareTo(Student other) {
return this.getName().compareTo(other.getName());
}
public String toString() {
return String.format("%-10s %-10d", this.getName(), this.getAge());
}
}
public class Main {
public static void main(String[] args) {
ArrayList<Student> students = new ArrayList<>();
students.add(new Student("John", 20));
students.add(new Student("Alice", 19));
students.add(new Student("Bob", 21));
students.add(new Student("Charlie", 18));
System.out.println("Before sorting:");
for (Student student : students) {
System.out.println(student);
}
Collections.sort(students);
System.out.println("After sorting:");
for (Student student : students) {
System.out.println(student);
}
}
}
```
In this example, the `Student` class implements the `Comparable` interface and overrides the `compareTo()` method to compare students based on their names. The `toString()` method formats the output using `String.format()` with the `%10s` and `%10d` format specifiers for the name and age fields, respectively. In the `Main` class, we create a list of students, print the list before sorting, sort the list using `Collections.sort()`, and print the list after sorting. The output will be:
```
Before sorting:
John 20
Alice 19
Bob 21
Charlie 18
After sorting:
Alice 19
Bob 21
Charlie 18
John 20
```
I hope this helps! Let me know if you have any other questions.
相关推荐
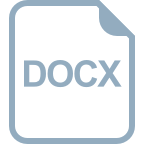
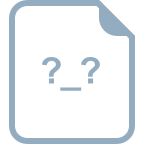















